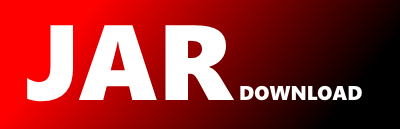
aws.sdk.kotlin.services.sagemaker.model.ActionSummary.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* Lists the properties of an action. An action represents an action
* or activity. Some examples are a workflow step and a model deployment. Generally, an
* action involves at least one input artifact or output artifact.
*/
class ActionSummary private constructor(builder: BuilderImpl) {
/**
* The Amazon Resource Name (ARN) of the action.
*/
val actionArn: String? = builder.actionArn
/**
* The name of the action.
*/
val actionName: String? = builder.actionName
/**
* The type of the action.
*/
val actionType: String? = builder.actionType
/**
* When the action was created.
*/
val creationTime: Instant? = builder.creationTime
/**
* When the action was last modified.
*/
val lastModifiedTime: Instant? = builder.lastModifiedTime
/**
* The source of the action.
*/
val source: ActionSource? = builder.source
/**
* The status of the action.
*/
val status: ActionStatus? = builder.status
companion object {
@JvmStatic
fun fluentBuilder(): FluentBuilder = BuilderImpl()
internal fun builder(): DslBuilder = BuilderImpl()
operator fun invoke(block: DslBuilder.() -> kotlin.Unit): ActionSummary = BuilderImpl().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ActionSummary(")
append("actionArn=$actionArn,")
append("actionName=$actionName,")
append("actionType=$actionType,")
append("creationTime=$creationTime,")
append("lastModifiedTime=$lastModifiedTime,")
append("source=$source,")
append("status=$status)")
}
override fun hashCode(): kotlin.Int {
var result = actionArn?.hashCode() ?: 0
result = 31 * result + (actionName?.hashCode() ?: 0)
result = 31 * result + (actionType?.hashCode() ?: 0)
result = 31 * result + (creationTime?.hashCode() ?: 0)
result = 31 * result + (lastModifiedTime?.hashCode() ?: 0)
result = 31 * result + (source?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as ActionSummary
if (actionArn != other.actionArn) return false
if (actionName != other.actionName) return false
if (actionType != other.actionType) return false
if (creationTime != other.creationTime) return false
if (lastModifiedTime != other.lastModifiedTime) return false
if (source != other.source) return false
if (status != other.status) return false
return true
}
fun copy(block: DslBuilder.() -> kotlin.Unit = {}): ActionSummary = BuilderImpl(this).apply(block).build()
interface FluentBuilder {
fun build(): ActionSummary
/**
* The Amazon Resource Name (ARN) of the action.
*/
fun actionArn(actionArn: String): FluentBuilder
/**
* The name of the action.
*/
fun actionName(actionName: String): FluentBuilder
/**
* The type of the action.
*/
fun actionType(actionType: String): FluentBuilder
/**
* When the action was created.
*/
fun creationTime(creationTime: Instant): FluentBuilder
/**
* When the action was last modified.
*/
fun lastModifiedTime(lastModifiedTime: Instant): FluentBuilder
/**
* The source of the action.
*/
fun source(source: ActionSource): FluentBuilder
/**
* The status of the action.
*/
fun status(status: ActionStatus): FluentBuilder
}
interface DslBuilder {
/**
* The Amazon Resource Name (ARN) of the action.
*/
var actionArn: String?
/**
* The name of the action.
*/
var actionName: String?
/**
* The type of the action.
*/
var actionType: String?
/**
* When the action was created.
*/
var creationTime: Instant?
/**
* When the action was last modified.
*/
var lastModifiedTime: Instant?
/**
* The source of the action.
*/
var source: ActionSource?
/**
* The status of the action.
*/
var status: ActionStatus?
fun build(): ActionSummary
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.ActionSource] inside the given [block]
*/
fun source(block: ActionSource.DslBuilder.() -> kotlin.Unit) {
this.source = ActionSource.invoke(block)
}
}
private class BuilderImpl() : FluentBuilder, DslBuilder {
override var actionArn: String? = null
override var actionName: String? = null
override var actionType: String? = null
override var creationTime: Instant? = null
override var lastModifiedTime: Instant? = null
override var source: ActionSource? = null
override var status: ActionStatus? = null
constructor(x: ActionSummary) : this() {
this.actionArn = x.actionArn
this.actionName = x.actionName
this.actionType = x.actionType
this.creationTime = x.creationTime
this.lastModifiedTime = x.lastModifiedTime
this.source = x.source
this.status = x.status
}
override fun build(): ActionSummary = ActionSummary(this)
override fun actionArn(actionArn: String): FluentBuilder = apply { this.actionArn = actionArn }
override fun actionName(actionName: String): FluentBuilder = apply { this.actionName = actionName }
override fun actionType(actionType: String): FluentBuilder = apply { this.actionType = actionType }
override fun creationTime(creationTime: Instant): FluentBuilder = apply { this.creationTime = creationTime }
override fun lastModifiedTime(lastModifiedTime: Instant): FluentBuilder = apply { this.lastModifiedTime = lastModifiedTime }
override fun source(source: ActionSource): FluentBuilder = apply { this.source = source }
override fun status(status: ActionStatus): FluentBuilder = apply { this.status = status }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy