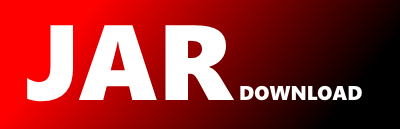
aws.sdk.kotlin.services.sagemaker.model.ChannelSpecification.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
/**
* Defines a named input source, called a channel, to be used by an algorithm.
*/
class ChannelSpecification private constructor(builder: BuilderImpl) {
/**
* A brief description of the channel.
*/
val description: String? = builder.description
/**
* Indicates whether the channel is required by the algorithm.
*/
val isRequired: Boolean = builder.isRequired
/**
* The name of the channel.
*/
val name: String? = builder.name
/**
* The allowed compression types, if data compression is used.
*/
val supportedCompressionTypes: List? = builder.supportedCompressionTypes
/**
* The supported MIME types for the data.
*/
val supportedContentTypes: List? = builder.supportedContentTypes
/**
* The allowed input mode, either FILE or PIPE.
* In FILE mode, Amazon SageMaker copies the data from the input source onto the local
* Amazon Elastic Block Store (Amazon EBS) volumes before starting your training algorithm.
* This is the most commonly used input mode.
* In PIPE mode, Amazon SageMaker streams input data from the source directly to your
* algorithm without using the EBS volume.
*/
val supportedInputModes: List? = builder.supportedInputModes
companion object {
@JvmStatic
fun fluentBuilder(): FluentBuilder = BuilderImpl()
internal fun builder(): DslBuilder = BuilderImpl()
operator fun invoke(block: DslBuilder.() -> kotlin.Unit): ChannelSpecification = BuilderImpl().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ChannelSpecification(")
append("description=$description,")
append("isRequired=$isRequired,")
append("name=$name,")
append("supportedCompressionTypes=$supportedCompressionTypes,")
append("supportedContentTypes=$supportedContentTypes,")
append("supportedInputModes=$supportedInputModes)")
}
override fun hashCode(): kotlin.Int {
var result = description?.hashCode() ?: 0
result = 31 * result + (isRequired.hashCode())
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (supportedCompressionTypes?.hashCode() ?: 0)
result = 31 * result + (supportedContentTypes?.hashCode() ?: 0)
result = 31 * result + (supportedInputModes?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as ChannelSpecification
if (description != other.description) return false
if (isRequired != other.isRequired) return false
if (name != other.name) return false
if (supportedCompressionTypes != other.supportedCompressionTypes) return false
if (supportedContentTypes != other.supportedContentTypes) return false
if (supportedInputModes != other.supportedInputModes) return false
return true
}
fun copy(block: DslBuilder.() -> kotlin.Unit = {}): ChannelSpecification = BuilderImpl(this).apply(block).build()
interface FluentBuilder {
fun build(): ChannelSpecification
/**
* A brief description of the channel.
*/
fun description(description: String): FluentBuilder
/**
* Indicates whether the channel is required by the algorithm.
*/
fun isRequired(isRequired: Boolean): FluentBuilder
/**
* The name of the channel.
*/
fun name(name: String): FluentBuilder
/**
* The allowed compression types, if data compression is used.
*/
fun supportedCompressionTypes(supportedCompressionTypes: List): FluentBuilder
/**
* The supported MIME types for the data.
*/
fun supportedContentTypes(supportedContentTypes: List): FluentBuilder
/**
* The allowed input mode, either FILE or PIPE.
* In FILE mode, Amazon SageMaker copies the data from the input source onto the local
* Amazon Elastic Block Store (Amazon EBS) volumes before starting your training algorithm.
* This is the most commonly used input mode.
* In PIPE mode, Amazon SageMaker streams input data from the source directly to your
* algorithm without using the EBS volume.
*/
fun supportedInputModes(supportedInputModes: List): FluentBuilder
}
interface DslBuilder {
/**
* A brief description of the channel.
*/
var description: String?
/**
* Indicates whether the channel is required by the algorithm.
*/
var isRequired: Boolean
/**
* The name of the channel.
*/
var name: String?
/**
* The allowed compression types, if data compression is used.
*/
var supportedCompressionTypes: List?
/**
* The supported MIME types for the data.
*/
var supportedContentTypes: List?
/**
* The allowed input mode, either FILE or PIPE.
* In FILE mode, Amazon SageMaker copies the data from the input source onto the local
* Amazon Elastic Block Store (Amazon EBS) volumes before starting your training algorithm.
* This is the most commonly used input mode.
* In PIPE mode, Amazon SageMaker streams input data from the source directly to your
* algorithm without using the EBS volume.
*/
var supportedInputModes: List?
fun build(): ChannelSpecification
}
private class BuilderImpl() : FluentBuilder, DslBuilder {
override var description: String? = null
override var isRequired: Boolean = false
override var name: String? = null
override var supportedCompressionTypes: List? = null
override var supportedContentTypes: List? = null
override var supportedInputModes: List? = null
constructor(x: ChannelSpecification) : this() {
this.description = x.description
this.isRequired = x.isRequired
this.name = x.name
this.supportedCompressionTypes = x.supportedCompressionTypes
this.supportedContentTypes = x.supportedContentTypes
this.supportedInputModes = x.supportedInputModes
}
override fun build(): ChannelSpecification = ChannelSpecification(this)
override fun description(description: String): FluentBuilder = apply { this.description = description }
override fun isRequired(isRequired: Boolean): FluentBuilder = apply { this.isRequired = isRequired }
override fun name(name: String): FluentBuilder = apply { this.name = name }
override fun supportedCompressionTypes(supportedCompressionTypes: List): FluentBuilder = apply { this.supportedCompressionTypes = supportedCompressionTypes }
override fun supportedContentTypes(supportedContentTypes: List): FluentBuilder = apply { this.supportedContentTypes = supportedContentTypes }
override fun supportedInputModes(supportedInputModes: List): FluentBuilder = apply { this.supportedInputModes = supportedInputModes }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy