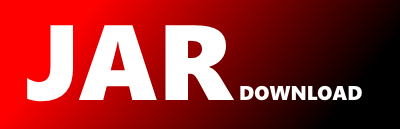
aws.sdk.kotlin.services.sagemaker.model.CognitoMemberDefinition.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
/**
* Identifies a Amazon Cognito user group. A user group can be used in on or more work
* teams.
*/
class CognitoMemberDefinition private constructor(builder: BuilderImpl) {
/**
* An identifier for an application client. You must create the app client ID using
* Amazon Cognito.
*/
val clientId: String? = builder.clientId
/**
* An identifier for a user group.
*/
val userGroup: String? = builder.userGroup
/**
* An identifier for a user pool. The user pool must be in the same region as the service
* that you are calling.
*/
val userPool: String? = builder.userPool
companion object {
@JvmStatic
fun fluentBuilder(): FluentBuilder = BuilderImpl()
internal fun builder(): DslBuilder = BuilderImpl()
operator fun invoke(block: DslBuilder.() -> kotlin.Unit): CognitoMemberDefinition = BuilderImpl().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CognitoMemberDefinition(")
append("clientId=$clientId,")
append("userGroup=$userGroup,")
append("userPool=$userPool)")
}
override fun hashCode(): kotlin.Int {
var result = clientId?.hashCode() ?: 0
result = 31 * result + (userGroup?.hashCode() ?: 0)
result = 31 * result + (userPool?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as CognitoMemberDefinition
if (clientId != other.clientId) return false
if (userGroup != other.userGroup) return false
if (userPool != other.userPool) return false
return true
}
fun copy(block: DslBuilder.() -> kotlin.Unit = {}): CognitoMemberDefinition = BuilderImpl(this).apply(block).build()
interface FluentBuilder {
fun build(): CognitoMemberDefinition
/**
* An identifier for an application client. You must create the app client ID using
* Amazon Cognito.
*/
fun clientId(clientId: String): FluentBuilder
/**
* An identifier for a user group.
*/
fun userGroup(userGroup: String): FluentBuilder
/**
* An identifier for a user pool. The user pool must be in the same region as the service
* that you are calling.
*/
fun userPool(userPool: String): FluentBuilder
}
interface DslBuilder {
/**
* An identifier for an application client. You must create the app client ID using
* Amazon Cognito.
*/
var clientId: String?
/**
* An identifier for a user group.
*/
var userGroup: String?
/**
* An identifier for a user pool. The user pool must be in the same region as the service
* that you are calling.
*/
var userPool: String?
fun build(): CognitoMemberDefinition
}
private class BuilderImpl() : FluentBuilder, DslBuilder {
override var clientId: String? = null
override var userGroup: String? = null
override var userPool: String? = null
constructor(x: CognitoMemberDefinition) : this() {
this.clientId = x.clientId
this.userGroup = x.userGroup
this.userPool = x.userPool
}
override fun build(): CognitoMemberDefinition = CognitoMemberDefinition(this)
override fun clientId(clientId: String): FluentBuilder = apply { this.clientId = clientId }
override fun userGroup(userGroup: String): FluentBuilder = apply { this.userGroup = userGroup }
override fun userPool(userPool: String): FluentBuilder = apply { this.userPool = userPool }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy