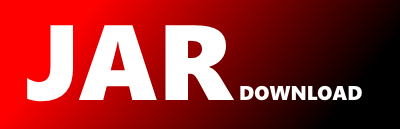
aws.sdk.kotlin.services.sagemaker.model.CreateActionRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
class CreateActionRequest private constructor(builder: BuilderImpl) {
/**
* The name of the action. Must be unique to your account in an Amazon Web Services Region.
*/
val actionName: String? = builder.actionName
/**
* The action type.
*/
val actionType: String? = builder.actionType
/**
* The description of the action.
*/
val description: String? = builder.description
/**
* Metadata properties of the tracking entity, trial, or trial component.
*/
val metadataProperties: MetadataProperties? = builder.metadataProperties
/**
* A list of properties to add to the action.
*/
val properties: Map? = builder.properties
/**
* The source type, ID, and URI.
*/
val source: ActionSource? = builder.source
/**
* The status of the action.
*/
val status: ActionStatus? = builder.status
/**
* A list of tags to apply to the action.
*/
val tags: List? = builder.tags
companion object {
@JvmStatic
fun fluentBuilder(): FluentBuilder = BuilderImpl()
internal fun builder(): DslBuilder = BuilderImpl()
operator fun invoke(block: DslBuilder.() -> kotlin.Unit): CreateActionRequest = BuilderImpl().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateActionRequest(")
append("actionName=$actionName,")
append("actionType=$actionType,")
append("description=$description,")
append("metadataProperties=$metadataProperties,")
append("properties=$properties,")
append("source=$source,")
append("status=$status,")
append("tags=$tags)")
}
override fun hashCode(): kotlin.Int {
var result = actionName?.hashCode() ?: 0
result = 31 * result + (actionType?.hashCode() ?: 0)
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (metadataProperties?.hashCode() ?: 0)
result = 31 * result + (properties?.hashCode() ?: 0)
result = 31 * result + (source?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as CreateActionRequest
if (actionName != other.actionName) return false
if (actionType != other.actionType) return false
if (description != other.description) return false
if (metadataProperties != other.metadataProperties) return false
if (properties != other.properties) return false
if (source != other.source) return false
if (status != other.status) return false
if (tags != other.tags) return false
return true
}
fun copy(block: DslBuilder.() -> kotlin.Unit = {}): CreateActionRequest = BuilderImpl(this).apply(block).build()
interface FluentBuilder {
fun build(): CreateActionRequest
/**
* The name of the action. Must be unique to your account in an Amazon Web Services Region.
*/
fun actionName(actionName: String): FluentBuilder
/**
* The action type.
*/
fun actionType(actionType: String): FluentBuilder
/**
* The description of the action.
*/
fun description(description: String): FluentBuilder
/**
* Metadata properties of the tracking entity, trial, or trial component.
*/
fun metadataProperties(metadataProperties: MetadataProperties): FluentBuilder
/**
* A list of properties to add to the action.
*/
fun properties(properties: Map): FluentBuilder
/**
* The source type, ID, and URI.
*/
fun source(source: ActionSource): FluentBuilder
/**
* The status of the action.
*/
fun status(status: ActionStatus): FluentBuilder
/**
* A list of tags to apply to the action.
*/
fun tags(tags: List): FluentBuilder
}
interface DslBuilder {
/**
* The name of the action. Must be unique to your account in an Amazon Web Services Region.
*/
var actionName: String?
/**
* The action type.
*/
var actionType: String?
/**
* The description of the action.
*/
var description: String?
/**
* Metadata properties of the tracking entity, trial, or trial component.
*/
var metadataProperties: MetadataProperties?
/**
* A list of properties to add to the action.
*/
var properties: Map?
/**
* The source type, ID, and URI.
*/
var source: ActionSource?
/**
* The status of the action.
*/
var status: ActionStatus?
/**
* A list of tags to apply to the action.
*/
var tags: List?
fun build(): CreateActionRequest
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.MetadataProperties] inside the given [block]
*/
fun metadataProperties(block: MetadataProperties.DslBuilder.() -> kotlin.Unit) {
this.metadataProperties = MetadataProperties.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.ActionSource] inside the given [block]
*/
fun source(block: ActionSource.DslBuilder.() -> kotlin.Unit) {
this.source = ActionSource.invoke(block)
}
}
private class BuilderImpl() : FluentBuilder, DslBuilder {
override var actionName: String? = null
override var actionType: String? = null
override var description: String? = null
override var metadataProperties: MetadataProperties? = null
override var properties: Map? = null
override var source: ActionSource? = null
override var status: ActionStatus? = null
override var tags: List? = null
constructor(x: CreateActionRequest) : this() {
this.actionName = x.actionName
this.actionType = x.actionType
this.description = x.description
this.metadataProperties = x.metadataProperties
this.properties = x.properties
this.source = x.source
this.status = x.status
this.tags = x.tags
}
override fun build(): CreateActionRequest = CreateActionRequest(this)
override fun actionName(actionName: String): FluentBuilder = apply { this.actionName = actionName }
override fun actionType(actionType: String): FluentBuilder = apply { this.actionType = actionType }
override fun description(description: String): FluentBuilder = apply { this.description = description }
override fun metadataProperties(metadataProperties: MetadataProperties): FluentBuilder = apply { this.metadataProperties = metadataProperties }
override fun properties(properties: Map): FluentBuilder = apply { this.properties = properties }
override fun source(source: ActionSource): FluentBuilder = apply { this.source = source }
override fun status(status: ActionStatus): FluentBuilder = apply { this.status = status }
override fun tags(tags: List): FluentBuilder = apply { this.tags = tags }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy