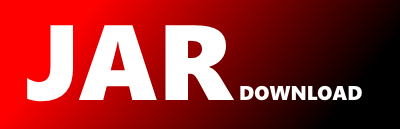
aws.sdk.kotlin.services.sagemaker.model.CreateContextRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
class CreateContextRequest private constructor(builder: BuilderImpl) {
/**
* The name of the context. Must be unique to your account in an Amazon Web Services Region.
*/
val contextName: String? = builder.contextName
/**
* The context type.
*/
val contextType: String? = builder.contextType
/**
* The description of the context.
*/
val description: String? = builder.description
/**
* A list of properties to add to the context.
*/
val properties: Map? = builder.properties
/**
* The source type, ID, and URI.
*/
val source: ContextSource? = builder.source
/**
* A list of tags to apply to the context.
*/
val tags: List? = builder.tags
companion object {
@JvmStatic
fun fluentBuilder(): FluentBuilder = BuilderImpl()
internal fun builder(): DslBuilder = BuilderImpl()
operator fun invoke(block: DslBuilder.() -> kotlin.Unit): CreateContextRequest = BuilderImpl().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateContextRequest(")
append("contextName=$contextName,")
append("contextType=$contextType,")
append("description=$description,")
append("properties=$properties,")
append("source=$source,")
append("tags=$tags)")
}
override fun hashCode(): kotlin.Int {
var result = contextName?.hashCode() ?: 0
result = 31 * result + (contextType?.hashCode() ?: 0)
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (properties?.hashCode() ?: 0)
result = 31 * result + (source?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as CreateContextRequest
if (contextName != other.contextName) return false
if (contextType != other.contextType) return false
if (description != other.description) return false
if (properties != other.properties) return false
if (source != other.source) return false
if (tags != other.tags) return false
return true
}
fun copy(block: DslBuilder.() -> kotlin.Unit = {}): CreateContextRequest = BuilderImpl(this).apply(block).build()
interface FluentBuilder {
fun build(): CreateContextRequest
/**
* The name of the context. Must be unique to your account in an Amazon Web Services Region.
*/
fun contextName(contextName: String): FluentBuilder
/**
* The context type.
*/
fun contextType(contextType: String): FluentBuilder
/**
* The description of the context.
*/
fun description(description: String): FluentBuilder
/**
* A list of properties to add to the context.
*/
fun properties(properties: Map): FluentBuilder
/**
* The source type, ID, and URI.
*/
fun source(source: ContextSource): FluentBuilder
/**
* A list of tags to apply to the context.
*/
fun tags(tags: List): FluentBuilder
}
interface DslBuilder {
/**
* The name of the context. Must be unique to your account in an Amazon Web Services Region.
*/
var contextName: String?
/**
* The context type.
*/
var contextType: String?
/**
* The description of the context.
*/
var description: String?
/**
* A list of properties to add to the context.
*/
var properties: Map?
/**
* The source type, ID, and URI.
*/
var source: ContextSource?
/**
* A list of tags to apply to the context.
*/
var tags: List?
fun build(): CreateContextRequest
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.ContextSource] inside the given [block]
*/
fun source(block: ContextSource.DslBuilder.() -> kotlin.Unit) {
this.source = ContextSource.invoke(block)
}
}
private class BuilderImpl() : FluentBuilder, DslBuilder {
override var contextName: String? = null
override var contextType: String? = null
override var description: String? = null
override var properties: Map? = null
override var source: ContextSource? = null
override var tags: List? = null
constructor(x: CreateContextRequest) : this() {
this.contextName = x.contextName
this.contextType = x.contextType
this.description = x.description
this.properties = x.properties
this.source = x.source
this.tags = x.tags
}
override fun build(): CreateContextRequest = CreateContextRequest(this)
override fun contextName(contextName: String): FluentBuilder = apply { this.contextName = contextName }
override fun contextType(contextType: String): FluentBuilder = apply { this.contextType = contextType }
override fun description(description: String): FluentBuilder = apply { this.description = description }
override fun properties(properties: Map): FluentBuilder = apply { this.properties = properties }
override fun source(source: ContextSource): FluentBuilder = apply { this.source = source }
override fun tags(tags: List): FluentBuilder = apply { this.tags = tags }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy