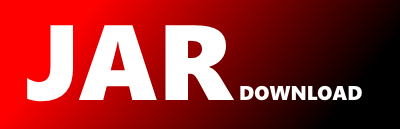
aws.sdk.kotlin.services.sagemaker.model.CreateProjectRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
class CreateProjectRequest private constructor(builder: BuilderImpl) {
/**
* A description for the project.
*/
val projectDescription: String? = builder.projectDescription
/**
* The name of the project.
*/
val projectName: String? = builder.projectName
/**
* The product ID and provisioning artifact ID to provision a service catalog. The provisioning
* artifact ID will default to the latest provisioning artifact ID of the product, if you don't
* provide the provisioning artifact ID. For more information, see What is Amazon Web Services Service
* Catalog.
*/
val serviceCatalogProvisioningDetails: ServiceCatalogProvisioningDetails? = builder.serviceCatalogProvisioningDetails
/**
* An array of key-value pairs that you want to use to organize and track your Amazon Web Services
* resource costs. For more information, see Tagging Amazon Web Services resources in the Amazon Web Services General Reference Guide.
*/
val tags: List? = builder.tags
companion object {
@JvmStatic
fun fluentBuilder(): FluentBuilder = BuilderImpl()
internal fun builder(): DslBuilder = BuilderImpl()
operator fun invoke(block: DslBuilder.() -> kotlin.Unit): CreateProjectRequest = BuilderImpl().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateProjectRequest(")
append("projectDescription=$projectDescription,")
append("projectName=$projectName,")
append("serviceCatalogProvisioningDetails=$serviceCatalogProvisioningDetails,")
append("tags=$tags)")
}
override fun hashCode(): kotlin.Int {
var result = projectDescription?.hashCode() ?: 0
result = 31 * result + (projectName?.hashCode() ?: 0)
result = 31 * result + (serviceCatalogProvisioningDetails?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as CreateProjectRequest
if (projectDescription != other.projectDescription) return false
if (projectName != other.projectName) return false
if (serviceCatalogProvisioningDetails != other.serviceCatalogProvisioningDetails) return false
if (tags != other.tags) return false
return true
}
fun copy(block: DslBuilder.() -> kotlin.Unit = {}): CreateProjectRequest = BuilderImpl(this).apply(block).build()
interface FluentBuilder {
fun build(): CreateProjectRequest
/**
* A description for the project.
*/
fun projectDescription(projectDescription: String): FluentBuilder
/**
* The name of the project.
*/
fun projectName(projectName: String): FluentBuilder
/**
* The product ID and provisioning artifact ID to provision a service catalog. The provisioning
* artifact ID will default to the latest provisioning artifact ID of the product, if you don't
* provide the provisioning artifact ID. For more information, see What is Amazon Web Services Service
* Catalog.
*/
fun serviceCatalogProvisioningDetails(serviceCatalogProvisioningDetails: ServiceCatalogProvisioningDetails): FluentBuilder
/**
* An array of key-value pairs that you want to use to organize and track your Amazon Web Services
* resource costs. For more information, see Tagging Amazon Web Services resources in the Amazon Web Services General Reference Guide.
*/
fun tags(tags: List): FluentBuilder
}
interface DslBuilder {
/**
* A description for the project.
*/
var projectDescription: String?
/**
* The name of the project.
*/
var projectName: String?
/**
* The product ID and provisioning artifact ID to provision a service catalog. The provisioning
* artifact ID will default to the latest provisioning artifact ID of the product, if you don't
* provide the provisioning artifact ID. For more information, see What is Amazon Web Services Service
* Catalog.
*/
var serviceCatalogProvisioningDetails: ServiceCatalogProvisioningDetails?
/**
* An array of key-value pairs that you want to use to organize and track your Amazon Web Services
* resource costs. For more information, see Tagging Amazon Web Services resources in the Amazon Web Services General Reference Guide.
*/
var tags: List?
fun build(): CreateProjectRequest
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.ServiceCatalogProvisioningDetails] inside the given [block]
*/
fun serviceCatalogProvisioningDetails(block: ServiceCatalogProvisioningDetails.DslBuilder.() -> kotlin.Unit) {
this.serviceCatalogProvisioningDetails = ServiceCatalogProvisioningDetails.invoke(block)
}
}
private class BuilderImpl() : FluentBuilder, DslBuilder {
override var projectDescription: String? = null
override var projectName: String? = null
override var serviceCatalogProvisioningDetails: ServiceCatalogProvisioningDetails? = null
override var tags: List? = null
constructor(x: CreateProjectRequest) : this() {
this.projectDescription = x.projectDescription
this.projectName = x.projectName
this.serviceCatalogProvisioningDetails = x.serviceCatalogProvisioningDetails
this.tags = x.tags
}
override fun build(): CreateProjectRequest = CreateProjectRequest(this)
override fun projectDescription(projectDescription: String): FluentBuilder = apply { this.projectDescription = projectDescription }
override fun projectName(projectName: String): FluentBuilder = apply { this.projectName = projectName }
override fun serviceCatalogProvisioningDetails(serviceCatalogProvisioningDetails: ServiceCatalogProvisioningDetails): FluentBuilder = apply { this.serviceCatalogProvisioningDetails = serviceCatalogProvisioningDetails }
override fun tags(tags: List): FluentBuilder = apply { this.tags = tags }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy