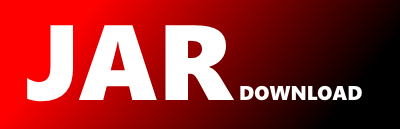
aws.sdk.kotlin.services.sagemaker.model.DatasetDefinition.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
/**
* Configuration for Dataset Definition inputs. The Dataset Definition input must specify
* exactly one of either AthenaDatasetDefinition or RedshiftDatasetDefinition
* types.
*/
class DatasetDefinition private constructor(builder: BuilderImpl) {
/**
* Configuration for Athena Dataset Definition input.
*/
val athenaDatasetDefinition: AthenaDatasetDefinition? = builder.athenaDatasetDefinition
/**
* Whether the generated dataset is FullyReplicated or
* ShardedByS3Key (default).
*/
val dataDistributionType: DataDistributionType? = builder.dataDistributionType
/**
* Whether to use File or Pipe input mode. In File (default) mode,
* Amazon SageMaker copies the data from the input source onto the local Amazon Elastic Block Store
* (Amazon EBS) volumes before starting your training algorithm. This is the most commonly used
* input mode. In Pipe mode, Amazon SageMaker streams input data from the source directly to your
* algorithm without using the EBS volume.
*/
val inputMode: InputMode? = builder.inputMode
/**
* The local path where you want Amazon SageMaker to download the Dataset Definition inputs to run a
* processing job. LocalPath is an absolute path to the input data. This is a required
* parameter when AppManaged is False (default).
*/
val localPath: String? = builder.localPath
/**
* Configuration for Redshift Dataset Definition input.
*/
val redshiftDatasetDefinition: RedshiftDatasetDefinition? = builder.redshiftDatasetDefinition
companion object {
@JvmStatic
fun fluentBuilder(): FluentBuilder = BuilderImpl()
internal fun builder(): DslBuilder = BuilderImpl()
operator fun invoke(block: DslBuilder.() -> kotlin.Unit): DatasetDefinition = BuilderImpl().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DatasetDefinition(")
append("athenaDatasetDefinition=$athenaDatasetDefinition,")
append("dataDistributionType=$dataDistributionType,")
append("inputMode=$inputMode,")
append("localPath=$localPath,")
append("redshiftDatasetDefinition=$redshiftDatasetDefinition)")
}
override fun hashCode(): kotlin.Int {
var result = athenaDatasetDefinition?.hashCode() ?: 0
result = 31 * result + (dataDistributionType?.hashCode() ?: 0)
result = 31 * result + (inputMode?.hashCode() ?: 0)
result = 31 * result + (localPath?.hashCode() ?: 0)
result = 31 * result + (redshiftDatasetDefinition?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as DatasetDefinition
if (athenaDatasetDefinition != other.athenaDatasetDefinition) return false
if (dataDistributionType != other.dataDistributionType) return false
if (inputMode != other.inputMode) return false
if (localPath != other.localPath) return false
if (redshiftDatasetDefinition != other.redshiftDatasetDefinition) return false
return true
}
fun copy(block: DslBuilder.() -> kotlin.Unit = {}): DatasetDefinition = BuilderImpl(this).apply(block).build()
interface FluentBuilder {
fun build(): DatasetDefinition
/**
* Configuration for Athena Dataset Definition input.
*/
fun athenaDatasetDefinition(athenaDatasetDefinition: AthenaDatasetDefinition): FluentBuilder
/**
* Whether the generated dataset is FullyReplicated or
* ShardedByS3Key (default).
*/
fun dataDistributionType(dataDistributionType: DataDistributionType): FluentBuilder
/**
* Whether to use File or Pipe input mode. In File (default) mode,
* Amazon SageMaker copies the data from the input source onto the local Amazon Elastic Block Store
* (Amazon EBS) volumes before starting your training algorithm. This is the most commonly used
* input mode. In Pipe mode, Amazon SageMaker streams input data from the source directly to your
* algorithm without using the EBS volume.
*/
fun inputMode(inputMode: InputMode): FluentBuilder
/**
* The local path where you want Amazon SageMaker to download the Dataset Definition inputs to run a
* processing job. LocalPath is an absolute path to the input data. This is a required
* parameter when AppManaged is False (default).
*/
fun localPath(localPath: String): FluentBuilder
/**
* Configuration for Redshift Dataset Definition input.
*/
fun redshiftDatasetDefinition(redshiftDatasetDefinition: RedshiftDatasetDefinition): FluentBuilder
}
interface DslBuilder {
/**
* Configuration for Athena Dataset Definition input.
*/
var athenaDatasetDefinition: AthenaDatasetDefinition?
/**
* Whether the generated dataset is FullyReplicated or
* ShardedByS3Key (default).
*/
var dataDistributionType: DataDistributionType?
/**
* Whether to use File or Pipe input mode. In File (default) mode,
* Amazon SageMaker copies the data from the input source onto the local Amazon Elastic Block Store
* (Amazon EBS) volumes before starting your training algorithm. This is the most commonly used
* input mode. In Pipe mode, Amazon SageMaker streams input data from the source directly to your
* algorithm without using the EBS volume.
*/
var inputMode: InputMode?
/**
* The local path where you want Amazon SageMaker to download the Dataset Definition inputs to run a
* processing job. LocalPath is an absolute path to the input data. This is a required
* parameter when AppManaged is False (default).
*/
var localPath: String?
/**
* Configuration for Redshift Dataset Definition input.
*/
var redshiftDatasetDefinition: RedshiftDatasetDefinition?
fun build(): DatasetDefinition
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.AthenaDatasetDefinition] inside the given [block]
*/
fun athenaDatasetDefinition(block: AthenaDatasetDefinition.DslBuilder.() -> kotlin.Unit) {
this.athenaDatasetDefinition = AthenaDatasetDefinition.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.RedshiftDatasetDefinition] inside the given [block]
*/
fun redshiftDatasetDefinition(block: RedshiftDatasetDefinition.DslBuilder.() -> kotlin.Unit) {
this.redshiftDatasetDefinition = RedshiftDatasetDefinition.invoke(block)
}
}
private class BuilderImpl() : FluentBuilder, DslBuilder {
override var athenaDatasetDefinition: AthenaDatasetDefinition? = null
override var dataDistributionType: DataDistributionType? = null
override var inputMode: InputMode? = null
override var localPath: String? = null
override var redshiftDatasetDefinition: RedshiftDatasetDefinition? = null
constructor(x: DatasetDefinition) : this() {
this.athenaDatasetDefinition = x.athenaDatasetDefinition
this.dataDistributionType = x.dataDistributionType
this.inputMode = x.inputMode
this.localPath = x.localPath
this.redshiftDatasetDefinition = x.redshiftDatasetDefinition
}
override fun build(): DatasetDefinition = DatasetDefinition(this)
override fun athenaDatasetDefinition(athenaDatasetDefinition: AthenaDatasetDefinition): FluentBuilder = apply { this.athenaDatasetDefinition = athenaDatasetDefinition }
override fun dataDistributionType(dataDistributionType: DataDistributionType): FluentBuilder = apply { this.dataDistributionType = dataDistributionType }
override fun inputMode(inputMode: InputMode): FluentBuilder = apply { this.inputMode = inputMode }
override fun localPath(localPath: String): FluentBuilder = apply { this.localPath = localPath }
override fun redshiftDatasetDefinition(redshiftDatasetDefinition: RedshiftDatasetDefinition): FluentBuilder = apply { this.redshiftDatasetDefinition = redshiftDatasetDefinition }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy