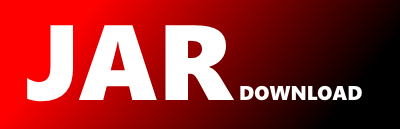
aws.sdk.kotlin.services.sagemaker.model.DebugRuleConfiguration.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
/**
* Configuration information for SageMaker Debugger rules for debugging. To learn more about
* how to configure the DebugRuleConfiguration parameter,
* see Use the SageMaker and Debugger Configuration API Operations to Create, Update, and Debug Your Training Job.
*/
class DebugRuleConfiguration private constructor(builder: BuilderImpl) {
/**
* The instance type to deploy a Debugger custom rule for debugging a training job.
*/
val instanceType: ProcessingInstanceType? = builder.instanceType
/**
* Path to local storage location for output of rules. Defaults to
* /opt/ml/processing/output/rule/.
*/
val localPath: String? = builder.localPath
/**
* The name of the rule configuration. It must be unique relative to other rule
* configuration names.
*/
val ruleConfigurationName: String? = builder.ruleConfigurationName
/**
* The Amazon Elastic Container (ECR) Image for the managed rule evaluation.
*/
val ruleEvaluatorImage: String? = builder.ruleEvaluatorImage
/**
* Runtime configuration for rule container.
*/
val ruleParameters: Map? = builder.ruleParameters
/**
* Path to Amazon S3 storage location for rules.
*/
val s3OutputPath: String? = builder.s3OutputPath
/**
* The size, in GB, of the ML storage volume attached to the processing instance.
*/
val volumeSizeInGb: Int = builder.volumeSizeInGb
companion object {
@JvmStatic
fun fluentBuilder(): FluentBuilder = BuilderImpl()
internal fun builder(): DslBuilder = BuilderImpl()
operator fun invoke(block: DslBuilder.() -> kotlin.Unit): DebugRuleConfiguration = BuilderImpl().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DebugRuleConfiguration(")
append("instanceType=$instanceType,")
append("localPath=$localPath,")
append("ruleConfigurationName=$ruleConfigurationName,")
append("ruleEvaluatorImage=$ruleEvaluatorImage,")
append("ruleParameters=$ruleParameters,")
append("s3OutputPath=$s3OutputPath,")
append("volumeSizeInGb=$volumeSizeInGb)")
}
override fun hashCode(): kotlin.Int {
var result = instanceType?.hashCode() ?: 0
result = 31 * result + (localPath?.hashCode() ?: 0)
result = 31 * result + (ruleConfigurationName?.hashCode() ?: 0)
result = 31 * result + (ruleEvaluatorImage?.hashCode() ?: 0)
result = 31 * result + (ruleParameters?.hashCode() ?: 0)
result = 31 * result + (s3OutputPath?.hashCode() ?: 0)
result = 31 * result + (volumeSizeInGb)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as DebugRuleConfiguration
if (instanceType != other.instanceType) return false
if (localPath != other.localPath) return false
if (ruleConfigurationName != other.ruleConfigurationName) return false
if (ruleEvaluatorImage != other.ruleEvaluatorImage) return false
if (ruleParameters != other.ruleParameters) return false
if (s3OutputPath != other.s3OutputPath) return false
if (volumeSizeInGb != other.volumeSizeInGb) return false
return true
}
fun copy(block: DslBuilder.() -> kotlin.Unit = {}): DebugRuleConfiguration = BuilderImpl(this).apply(block).build()
interface FluentBuilder {
fun build(): DebugRuleConfiguration
/**
* The instance type to deploy a Debugger custom rule for debugging a training job.
*/
fun instanceType(instanceType: ProcessingInstanceType): FluentBuilder
/**
* Path to local storage location for output of rules. Defaults to
* /opt/ml/processing/output/rule/.
*/
fun localPath(localPath: String): FluentBuilder
/**
* The name of the rule configuration. It must be unique relative to other rule
* configuration names.
*/
fun ruleConfigurationName(ruleConfigurationName: String): FluentBuilder
/**
* The Amazon Elastic Container (ECR) Image for the managed rule evaluation.
*/
fun ruleEvaluatorImage(ruleEvaluatorImage: String): FluentBuilder
/**
* Runtime configuration for rule container.
*/
fun ruleParameters(ruleParameters: Map): FluentBuilder
/**
* Path to Amazon S3 storage location for rules.
*/
fun s3OutputPath(s3OutputPath: String): FluentBuilder
/**
* The size, in GB, of the ML storage volume attached to the processing instance.
*/
fun volumeSizeInGb(volumeSizeInGb: Int): FluentBuilder
}
interface DslBuilder {
/**
* The instance type to deploy a Debugger custom rule for debugging a training job.
*/
var instanceType: ProcessingInstanceType?
/**
* Path to local storage location for output of rules. Defaults to
* /opt/ml/processing/output/rule/.
*/
var localPath: String?
/**
* The name of the rule configuration. It must be unique relative to other rule
* configuration names.
*/
var ruleConfigurationName: String?
/**
* The Amazon Elastic Container (ECR) Image for the managed rule evaluation.
*/
var ruleEvaluatorImage: String?
/**
* Runtime configuration for rule container.
*/
var ruleParameters: Map?
/**
* Path to Amazon S3 storage location for rules.
*/
var s3OutputPath: String?
/**
* The size, in GB, of the ML storage volume attached to the processing instance.
*/
var volumeSizeInGb: Int
fun build(): DebugRuleConfiguration
}
private class BuilderImpl() : FluentBuilder, DslBuilder {
override var instanceType: ProcessingInstanceType? = null
override var localPath: String? = null
override var ruleConfigurationName: String? = null
override var ruleEvaluatorImage: String? = null
override var ruleParameters: Map? = null
override var s3OutputPath: String? = null
override var volumeSizeInGb: Int = 0
constructor(x: DebugRuleConfiguration) : this() {
this.instanceType = x.instanceType
this.localPath = x.localPath
this.ruleConfigurationName = x.ruleConfigurationName
this.ruleEvaluatorImage = x.ruleEvaluatorImage
this.ruleParameters = x.ruleParameters
this.s3OutputPath = x.s3OutputPath
this.volumeSizeInGb = x.volumeSizeInGb
}
override fun build(): DebugRuleConfiguration = DebugRuleConfiguration(this)
override fun instanceType(instanceType: ProcessingInstanceType): FluentBuilder = apply { this.instanceType = instanceType }
override fun localPath(localPath: String): FluentBuilder = apply { this.localPath = localPath }
override fun ruleConfigurationName(ruleConfigurationName: String): FluentBuilder = apply { this.ruleConfigurationName = ruleConfigurationName }
override fun ruleEvaluatorImage(ruleEvaluatorImage: String): FluentBuilder = apply { this.ruleEvaluatorImage = ruleEvaluatorImage }
override fun ruleParameters(ruleParameters: Map): FluentBuilder = apply { this.ruleParameters = ruleParameters }
override fun s3OutputPath(s3OutputPath: String): FluentBuilder = apply { this.s3OutputPath = s3OutputPath }
override fun volumeSizeInGb(volumeSizeInGb: Int): FluentBuilder = apply { this.volumeSizeInGb = volumeSizeInGb }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy