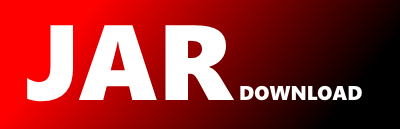
aws.sdk.kotlin.services.sagemaker.model.DescribeDeviceResponse.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
import aws.smithy.kotlin.runtime.time.Instant
class DescribeDeviceResponse private constructor(builder: BuilderImpl) {
/**
* A description of the device.
*/
val description: String? = builder.description
/**
* The Amazon Resource Name (ARN) of the device.
*/
val deviceArn: String? = builder.deviceArn
/**
* The name of the fleet the device belongs to.
*/
val deviceFleetName: String? = builder.deviceFleetName
/**
* The unique identifier of the device.
*/
val deviceName: String? = builder.deviceName
/**
* The Amazon Web Services Internet of Things (IoT) object thing name associated with the device.
*/
val iotThingName: String? = builder.iotThingName
/**
* The last heartbeat received from the device.
*/
val latestHeartbeat: Instant? = builder.latestHeartbeat
/**
* The maximum number of models.
*/
val maxModels: Int = builder.maxModels
/**
* Models on the device.
*/
val models: List? = builder.models
/**
* The response from the last list when returning a list large enough to need tokening.
*/
val nextToken: String? = builder.nextToken
/**
* The timestamp of the last registration or de-reregistration.
*/
val registrationTime: Instant? = builder.registrationTime
companion object {
@JvmStatic
fun fluentBuilder(): FluentBuilder = BuilderImpl()
internal fun builder(): DslBuilder = BuilderImpl()
operator fun invoke(block: DslBuilder.() -> kotlin.Unit): DescribeDeviceResponse = BuilderImpl().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeDeviceResponse(")
append("description=$description,")
append("deviceArn=$deviceArn,")
append("deviceFleetName=$deviceFleetName,")
append("deviceName=$deviceName,")
append("iotThingName=$iotThingName,")
append("latestHeartbeat=$latestHeartbeat,")
append("maxModels=$maxModels,")
append("models=$models,")
append("nextToken=$nextToken,")
append("registrationTime=$registrationTime)")
}
override fun hashCode(): kotlin.Int {
var result = description?.hashCode() ?: 0
result = 31 * result + (deviceArn?.hashCode() ?: 0)
result = 31 * result + (deviceFleetName?.hashCode() ?: 0)
result = 31 * result + (deviceName?.hashCode() ?: 0)
result = 31 * result + (iotThingName?.hashCode() ?: 0)
result = 31 * result + (latestHeartbeat?.hashCode() ?: 0)
result = 31 * result + (maxModels)
result = 31 * result + (models?.hashCode() ?: 0)
result = 31 * result + (nextToken?.hashCode() ?: 0)
result = 31 * result + (registrationTime?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as DescribeDeviceResponse
if (description != other.description) return false
if (deviceArn != other.deviceArn) return false
if (deviceFleetName != other.deviceFleetName) return false
if (deviceName != other.deviceName) return false
if (iotThingName != other.iotThingName) return false
if (latestHeartbeat != other.latestHeartbeat) return false
if (maxModels != other.maxModels) return false
if (models != other.models) return false
if (nextToken != other.nextToken) return false
if (registrationTime != other.registrationTime) return false
return true
}
fun copy(block: DslBuilder.() -> kotlin.Unit = {}): DescribeDeviceResponse = BuilderImpl(this).apply(block).build()
interface FluentBuilder {
fun build(): DescribeDeviceResponse
/**
* A description of the device.
*/
fun description(description: String): FluentBuilder
/**
* The Amazon Resource Name (ARN) of the device.
*/
fun deviceArn(deviceArn: String): FluentBuilder
/**
* The name of the fleet the device belongs to.
*/
fun deviceFleetName(deviceFleetName: String): FluentBuilder
/**
* The unique identifier of the device.
*/
fun deviceName(deviceName: String): FluentBuilder
/**
* The Amazon Web Services Internet of Things (IoT) object thing name associated with the device.
*/
fun iotThingName(iotThingName: String): FluentBuilder
/**
* The last heartbeat received from the device.
*/
fun latestHeartbeat(latestHeartbeat: Instant): FluentBuilder
/**
* The maximum number of models.
*/
fun maxModels(maxModels: Int): FluentBuilder
/**
* Models on the device.
*/
fun models(models: List): FluentBuilder
/**
* The response from the last list when returning a list large enough to need tokening.
*/
fun nextToken(nextToken: String): FluentBuilder
/**
* The timestamp of the last registration or de-reregistration.
*/
fun registrationTime(registrationTime: Instant): FluentBuilder
}
interface DslBuilder {
/**
* A description of the device.
*/
var description: String?
/**
* The Amazon Resource Name (ARN) of the device.
*/
var deviceArn: String?
/**
* The name of the fleet the device belongs to.
*/
var deviceFleetName: String?
/**
* The unique identifier of the device.
*/
var deviceName: String?
/**
* The Amazon Web Services Internet of Things (IoT) object thing name associated with the device.
*/
var iotThingName: String?
/**
* The last heartbeat received from the device.
*/
var latestHeartbeat: Instant?
/**
* The maximum number of models.
*/
var maxModels: Int
/**
* Models on the device.
*/
var models: List?
/**
* The response from the last list when returning a list large enough to need tokening.
*/
var nextToken: String?
/**
* The timestamp of the last registration or de-reregistration.
*/
var registrationTime: Instant?
fun build(): DescribeDeviceResponse
}
private class BuilderImpl() : FluentBuilder, DslBuilder {
override var description: String? = null
override var deviceArn: String? = null
override var deviceFleetName: String? = null
override var deviceName: String? = null
override var iotThingName: String? = null
override var latestHeartbeat: Instant? = null
override var maxModels: Int = 0
override var models: List? = null
override var nextToken: String? = null
override var registrationTime: Instant? = null
constructor(x: DescribeDeviceResponse) : this() {
this.description = x.description
this.deviceArn = x.deviceArn
this.deviceFleetName = x.deviceFleetName
this.deviceName = x.deviceName
this.iotThingName = x.iotThingName
this.latestHeartbeat = x.latestHeartbeat
this.maxModels = x.maxModels
this.models = x.models
this.nextToken = x.nextToken
this.registrationTime = x.registrationTime
}
override fun build(): DescribeDeviceResponse = DescribeDeviceResponse(this)
override fun description(description: String): FluentBuilder = apply { this.description = description }
override fun deviceArn(deviceArn: String): FluentBuilder = apply { this.deviceArn = deviceArn }
override fun deviceFleetName(deviceFleetName: String): FluentBuilder = apply { this.deviceFleetName = deviceFleetName }
override fun deviceName(deviceName: String): FluentBuilder = apply { this.deviceName = deviceName }
override fun iotThingName(iotThingName: String): FluentBuilder = apply { this.iotThingName = iotThingName }
override fun latestHeartbeat(latestHeartbeat: Instant): FluentBuilder = apply { this.latestHeartbeat = latestHeartbeat }
override fun maxModels(maxModels: Int): FluentBuilder = apply { this.maxModels = maxModels }
override fun models(models: List): FluentBuilder = apply { this.models = models }
override fun nextToken(nextToken: String): FluentBuilder = apply { this.nextToken = nextToken }
override fun registrationTime(registrationTime: Instant): FluentBuilder = apply { this.registrationTime = registrationTime }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy