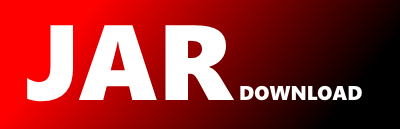
aws.sdk.kotlin.services.sagemaker.model.DescribeNotebookInstanceResponse.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
import aws.smithy.kotlin.runtime.time.Instant
class DescribeNotebookInstanceResponse private constructor(builder: BuilderImpl) {
/**
* A list of the Elastic Inference (EI) instance types associated with this notebook
* instance. Currently only one EI instance type can be associated with a notebook
* instance. For more information, see Using Elastic Inference in Amazon
* SageMaker.
*/
val acceleratorTypes: List? = builder.acceleratorTypes
/**
* An array of up to three Git repositories associated with the notebook instance. These
* can be either the names of Git repositories stored as resources in your account, or the
* URL of Git repositories in Amazon Web Services CodeCommit or in any
* other Git repository. These repositories are cloned at the same level as the default
* repository of your notebook instance. For more information, see Associating Git
* Repositories with Amazon SageMaker Notebook Instances.
*/
val additionalCodeRepositories: List? = builder.additionalCodeRepositories
/**
* A timestamp. Use this parameter to return the time when the notebook instance was
* created
*/
val creationTime: Instant? = builder.creationTime
/**
* The Git repository associated with the notebook instance as its default code
* repository. This can be either the name of a Git repository stored as a resource in your
* account, or the URL of a Git repository in Amazon Web Services CodeCommit or in any
* other Git repository. When you open a notebook instance, it opens in the directory that
* contains this repository. For more information, see Associating Git Repositories with Amazon SageMaker
* Notebook Instances.
*/
val defaultCodeRepository: String? = builder.defaultCodeRepository
/**
* Describes whether Amazon SageMaker provides internet access to the notebook instance. If this
* value is set to Disabled, the notebook instance does not have
* internet access, and cannot connect to Amazon SageMaker training and endpoint services.
* For more information, see Notebook Instances Are Internet-Enabled by Default.
*/
val directInternetAccess: DirectInternetAccess? = builder.directInternetAccess
/**
* If status is Failed, the reason it failed.
*/
val failureReason: String? = builder.failureReason
/**
* The type of ML compute instance running on the notebook instance.
*/
val instanceType: InstanceType? = builder.instanceType
/**
* The Amazon Web Services KMS key ID Amazon SageMaker uses to encrypt data when storing it on the ML storage
* volume attached to the instance.
*/
val kmsKeyId: String? = builder.kmsKeyId
/**
* A timestamp. Use this parameter to retrieve the time when the notebook instance was
* last modified.
*/
val lastModifiedTime: Instant? = builder.lastModifiedTime
/**
* The network interface IDs that Amazon SageMaker created at the time of creating the instance.
*/
val networkInterfaceId: String? = builder.networkInterfaceId
/**
* The Amazon Resource Name (ARN) of the notebook instance.
*/
val notebookInstanceArn: String? = builder.notebookInstanceArn
/**
* Returns the name of a notebook instance lifecycle configuration.
* For information about notebook instance lifestyle configurations, see Step
* 2.1: (Optional) Customize a Notebook Instance
*/
val notebookInstanceLifecycleConfigName: String? = builder.notebookInstanceLifecycleConfigName
/**
* The name of the Amazon SageMaker notebook instance.
*/
val notebookInstanceName: String? = builder.notebookInstanceName
/**
* The status of the notebook instance.
*/
val notebookInstanceStatus: NotebookInstanceStatus? = builder.notebookInstanceStatus
/**
* The platform identifier of the notebook instance runtime environment.
*/
val platformIdentifier: String? = builder.platformIdentifier
/**
* The Amazon Resource Name (ARN) of the IAM role associated with the instance.
*/
val roleArn: String? = builder.roleArn
/**
* Whether root access is enabled or disabled for users of the notebook instance.
* Lifecycle configurations need root access to be able to set up a notebook
* instance. Because of this, lifecycle configurations associated with a notebook
* instance always run with root access even if you disable root access for
* users.
*/
val rootAccess: RootAccess? = builder.rootAccess
/**
* The IDs of the VPC security groups.
*/
val securityGroups: List? = builder.securityGroups
/**
* The ID of the VPC subnet.
*/
val subnetId: String? = builder.subnetId
/**
* The URL that you use to connect to the Jupyter notebook that is running in your
* notebook instance.
*/
val url: String? = builder.url
/**
* The size, in GB, of the ML storage volume attached to the notebook instance.
*/
val volumeSizeInGb: Int? = builder.volumeSizeInGb
companion object {
@JvmStatic
fun fluentBuilder(): FluentBuilder = BuilderImpl()
internal fun builder(): DslBuilder = BuilderImpl()
operator fun invoke(block: DslBuilder.() -> kotlin.Unit): DescribeNotebookInstanceResponse = BuilderImpl().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeNotebookInstanceResponse(")
append("acceleratorTypes=$acceleratorTypes,")
append("additionalCodeRepositories=$additionalCodeRepositories,")
append("creationTime=$creationTime,")
append("defaultCodeRepository=$defaultCodeRepository,")
append("directInternetAccess=$directInternetAccess,")
append("failureReason=$failureReason,")
append("instanceType=$instanceType,")
append("kmsKeyId=$kmsKeyId,")
append("lastModifiedTime=$lastModifiedTime,")
append("networkInterfaceId=$networkInterfaceId,")
append("notebookInstanceArn=$notebookInstanceArn,")
append("notebookInstanceLifecycleConfigName=$notebookInstanceLifecycleConfigName,")
append("notebookInstanceName=$notebookInstanceName,")
append("notebookInstanceStatus=$notebookInstanceStatus,")
append("platformIdentifier=$platformIdentifier,")
append("roleArn=$roleArn,")
append("rootAccess=$rootAccess,")
append("securityGroups=$securityGroups,")
append("subnetId=$subnetId,")
append("url=$url,")
append("volumeSizeInGb=$volumeSizeInGb)")
}
override fun hashCode(): kotlin.Int {
var result = acceleratorTypes?.hashCode() ?: 0
result = 31 * result + (additionalCodeRepositories?.hashCode() ?: 0)
result = 31 * result + (creationTime?.hashCode() ?: 0)
result = 31 * result + (defaultCodeRepository?.hashCode() ?: 0)
result = 31 * result + (directInternetAccess?.hashCode() ?: 0)
result = 31 * result + (failureReason?.hashCode() ?: 0)
result = 31 * result + (instanceType?.hashCode() ?: 0)
result = 31 * result + (kmsKeyId?.hashCode() ?: 0)
result = 31 * result + (lastModifiedTime?.hashCode() ?: 0)
result = 31 * result + (networkInterfaceId?.hashCode() ?: 0)
result = 31 * result + (notebookInstanceArn?.hashCode() ?: 0)
result = 31 * result + (notebookInstanceLifecycleConfigName?.hashCode() ?: 0)
result = 31 * result + (notebookInstanceName?.hashCode() ?: 0)
result = 31 * result + (notebookInstanceStatus?.hashCode() ?: 0)
result = 31 * result + (platformIdentifier?.hashCode() ?: 0)
result = 31 * result + (roleArn?.hashCode() ?: 0)
result = 31 * result + (rootAccess?.hashCode() ?: 0)
result = 31 * result + (securityGroups?.hashCode() ?: 0)
result = 31 * result + (subnetId?.hashCode() ?: 0)
result = 31 * result + (url?.hashCode() ?: 0)
result = 31 * result + (volumeSizeInGb ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as DescribeNotebookInstanceResponse
if (acceleratorTypes != other.acceleratorTypes) return false
if (additionalCodeRepositories != other.additionalCodeRepositories) return false
if (creationTime != other.creationTime) return false
if (defaultCodeRepository != other.defaultCodeRepository) return false
if (directInternetAccess != other.directInternetAccess) return false
if (failureReason != other.failureReason) return false
if (instanceType != other.instanceType) return false
if (kmsKeyId != other.kmsKeyId) return false
if (lastModifiedTime != other.lastModifiedTime) return false
if (networkInterfaceId != other.networkInterfaceId) return false
if (notebookInstanceArn != other.notebookInstanceArn) return false
if (notebookInstanceLifecycleConfigName != other.notebookInstanceLifecycleConfigName) return false
if (notebookInstanceName != other.notebookInstanceName) return false
if (notebookInstanceStatus != other.notebookInstanceStatus) return false
if (platformIdentifier != other.platformIdentifier) return false
if (roleArn != other.roleArn) return false
if (rootAccess != other.rootAccess) return false
if (securityGroups != other.securityGroups) return false
if (subnetId != other.subnetId) return false
if (url != other.url) return false
if (volumeSizeInGb != other.volumeSizeInGb) return false
return true
}
fun copy(block: DslBuilder.() -> kotlin.Unit = {}): DescribeNotebookInstanceResponse = BuilderImpl(this).apply(block).build()
interface FluentBuilder {
fun build(): DescribeNotebookInstanceResponse
/**
* A list of the Elastic Inference (EI) instance types associated with this notebook
* instance. Currently only one EI instance type can be associated with a notebook
* instance. For more information, see Using Elastic Inference in Amazon
* SageMaker.
*/
fun acceleratorTypes(acceleratorTypes: List): FluentBuilder
/**
* An array of up to three Git repositories associated with the notebook instance. These
* can be either the names of Git repositories stored as resources in your account, or the
* URL of Git repositories in Amazon Web Services CodeCommit or in any
* other Git repository. These repositories are cloned at the same level as the default
* repository of your notebook instance. For more information, see Associating Git
* Repositories with Amazon SageMaker Notebook Instances.
*/
fun additionalCodeRepositories(additionalCodeRepositories: List): FluentBuilder
/**
* A timestamp. Use this parameter to return the time when the notebook instance was
* created
*/
fun creationTime(creationTime: Instant): FluentBuilder
/**
* The Git repository associated with the notebook instance as its default code
* repository. This can be either the name of a Git repository stored as a resource in your
* account, or the URL of a Git repository in Amazon Web Services CodeCommit or in any
* other Git repository. When you open a notebook instance, it opens in the directory that
* contains this repository. For more information, see Associating Git Repositories with Amazon SageMaker
* Notebook Instances.
*/
fun defaultCodeRepository(defaultCodeRepository: String): FluentBuilder
/**
* Describes whether Amazon SageMaker provides internet access to the notebook instance. If this
* value is set to Disabled, the notebook instance does not have
* internet access, and cannot connect to Amazon SageMaker training and endpoint services.
* For more information, see Notebook Instances Are Internet-Enabled by Default.
*/
fun directInternetAccess(directInternetAccess: DirectInternetAccess): FluentBuilder
/**
* If status is Failed, the reason it failed.
*/
fun failureReason(failureReason: String): FluentBuilder
/**
* The type of ML compute instance running on the notebook instance.
*/
fun instanceType(instanceType: InstanceType): FluentBuilder
/**
* The Amazon Web Services KMS key ID Amazon SageMaker uses to encrypt data when storing it on the ML storage
* volume attached to the instance.
*/
fun kmsKeyId(kmsKeyId: String): FluentBuilder
/**
* A timestamp. Use this parameter to retrieve the time when the notebook instance was
* last modified.
*/
fun lastModifiedTime(lastModifiedTime: Instant): FluentBuilder
/**
* The network interface IDs that Amazon SageMaker created at the time of creating the instance.
*/
fun networkInterfaceId(networkInterfaceId: String): FluentBuilder
/**
* The Amazon Resource Name (ARN) of the notebook instance.
*/
fun notebookInstanceArn(notebookInstanceArn: String): FluentBuilder
/**
* Returns the name of a notebook instance lifecycle configuration.
* For information about notebook instance lifestyle configurations, see Step
* 2.1: (Optional) Customize a Notebook Instance
*/
fun notebookInstanceLifecycleConfigName(notebookInstanceLifecycleConfigName: String): FluentBuilder
/**
* The name of the Amazon SageMaker notebook instance.
*/
fun notebookInstanceName(notebookInstanceName: String): FluentBuilder
/**
* The status of the notebook instance.
*/
fun notebookInstanceStatus(notebookInstanceStatus: NotebookInstanceStatus): FluentBuilder
/**
* The platform identifier of the notebook instance runtime environment.
*/
fun platformIdentifier(platformIdentifier: String): FluentBuilder
/**
* The Amazon Resource Name (ARN) of the IAM role associated with the instance.
*/
fun roleArn(roleArn: String): FluentBuilder
/**
* Whether root access is enabled or disabled for users of the notebook instance.
* Lifecycle configurations need root access to be able to set up a notebook
* instance. Because of this, lifecycle configurations associated with a notebook
* instance always run with root access even if you disable root access for
* users.
*/
fun rootAccess(rootAccess: RootAccess): FluentBuilder
/**
* The IDs of the VPC security groups.
*/
fun securityGroups(securityGroups: List): FluentBuilder
/**
* The ID of the VPC subnet.
*/
fun subnetId(subnetId: String): FluentBuilder
/**
* The URL that you use to connect to the Jupyter notebook that is running in your
* notebook instance.
*/
fun url(url: String): FluentBuilder
/**
* The size, in GB, of the ML storage volume attached to the notebook instance.
*/
fun volumeSizeInGb(volumeSizeInGb: Int): FluentBuilder
}
interface DslBuilder {
/**
* A list of the Elastic Inference (EI) instance types associated with this notebook
* instance. Currently only one EI instance type can be associated with a notebook
* instance. For more information, see Using Elastic Inference in Amazon
* SageMaker.
*/
var acceleratorTypes: List?
/**
* An array of up to three Git repositories associated with the notebook instance. These
* can be either the names of Git repositories stored as resources in your account, or the
* URL of Git repositories in Amazon Web Services CodeCommit or in any
* other Git repository. These repositories are cloned at the same level as the default
* repository of your notebook instance. For more information, see Associating Git
* Repositories with Amazon SageMaker Notebook Instances.
*/
var additionalCodeRepositories: List?
/**
* A timestamp. Use this parameter to return the time when the notebook instance was
* created
*/
var creationTime: Instant?
/**
* The Git repository associated with the notebook instance as its default code
* repository. This can be either the name of a Git repository stored as a resource in your
* account, or the URL of a Git repository in Amazon Web Services CodeCommit or in any
* other Git repository. When you open a notebook instance, it opens in the directory that
* contains this repository. For more information, see Associating Git Repositories with Amazon SageMaker
* Notebook Instances.
*/
var defaultCodeRepository: String?
/**
* Describes whether Amazon SageMaker provides internet access to the notebook instance. If this
* value is set to Disabled, the notebook instance does not have
* internet access, and cannot connect to Amazon SageMaker training and endpoint services.
* For more information, see Notebook Instances Are Internet-Enabled by Default.
*/
var directInternetAccess: DirectInternetAccess?
/**
* If status is Failed, the reason it failed.
*/
var failureReason: String?
/**
* The type of ML compute instance running on the notebook instance.
*/
var instanceType: InstanceType?
/**
* The Amazon Web Services KMS key ID Amazon SageMaker uses to encrypt data when storing it on the ML storage
* volume attached to the instance.
*/
var kmsKeyId: String?
/**
* A timestamp. Use this parameter to retrieve the time when the notebook instance was
* last modified.
*/
var lastModifiedTime: Instant?
/**
* The network interface IDs that Amazon SageMaker created at the time of creating the instance.
*/
var networkInterfaceId: String?
/**
* The Amazon Resource Name (ARN) of the notebook instance.
*/
var notebookInstanceArn: String?
/**
* Returns the name of a notebook instance lifecycle configuration.
* For information about notebook instance lifestyle configurations, see Step
* 2.1: (Optional) Customize a Notebook Instance
*/
var notebookInstanceLifecycleConfigName: String?
/**
* The name of the Amazon SageMaker notebook instance.
*/
var notebookInstanceName: String?
/**
* The status of the notebook instance.
*/
var notebookInstanceStatus: NotebookInstanceStatus?
/**
* The platform identifier of the notebook instance runtime environment.
*/
var platformIdentifier: String?
/**
* The Amazon Resource Name (ARN) of the IAM role associated with the instance.
*/
var roleArn: String?
/**
* Whether root access is enabled or disabled for users of the notebook instance.
* Lifecycle configurations need root access to be able to set up a notebook
* instance. Because of this, lifecycle configurations associated with a notebook
* instance always run with root access even if you disable root access for
* users.
*/
var rootAccess: RootAccess?
/**
* The IDs of the VPC security groups.
*/
var securityGroups: List?
/**
* The ID of the VPC subnet.
*/
var subnetId: String?
/**
* The URL that you use to connect to the Jupyter notebook that is running in your
* notebook instance.
*/
var url: String?
/**
* The size, in GB, of the ML storage volume attached to the notebook instance.
*/
var volumeSizeInGb: Int?
fun build(): DescribeNotebookInstanceResponse
}
private class BuilderImpl() : FluentBuilder, DslBuilder {
override var acceleratorTypes: List? = null
override var additionalCodeRepositories: List? = null
override var creationTime: Instant? = null
override var defaultCodeRepository: String? = null
override var directInternetAccess: DirectInternetAccess? = null
override var failureReason: String? = null
override var instanceType: InstanceType? = null
override var kmsKeyId: String? = null
override var lastModifiedTime: Instant? = null
override var networkInterfaceId: String? = null
override var notebookInstanceArn: String? = null
override var notebookInstanceLifecycleConfigName: String? = null
override var notebookInstanceName: String? = null
override var notebookInstanceStatus: NotebookInstanceStatus? = null
override var platformIdentifier: String? = null
override var roleArn: String? = null
override var rootAccess: RootAccess? = null
override var securityGroups: List? = null
override var subnetId: String? = null
override var url: String? = null
override var volumeSizeInGb: Int? = null
constructor(x: DescribeNotebookInstanceResponse) : this() {
this.acceleratorTypes = x.acceleratorTypes
this.additionalCodeRepositories = x.additionalCodeRepositories
this.creationTime = x.creationTime
this.defaultCodeRepository = x.defaultCodeRepository
this.directInternetAccess = x.directInternetAccess
this.failureReason = x.failureReason
this.instanceType = x.instanceType
this.kmsKeyId = x.kmsKeyId
this.lastModifiedTime = x.lastModifiedTime
this.networkInterfaceId = x.networkInterfaceId
this.notebookInstanceArn = x.notebookInstanceArn
this.notebookInstanceLifecycleConfigName = x.notebookInstanceLifecycleConfigName
this.notebookInstanceName = x.notebookInstanceName
this.notebookInstanceStatus = x.notebookInstanceStatus
this.platformIdentifier = x.platformIdentifier
this.roleArn = x.roleArn
this.rootAccess = x.rootAccess
this.securityGroups = x.securityGroups
this.subnetId = x.subnetId
this.url = x.url
this.volumeSizeInGb = x.volumeSizeInGb
}
override fun build(): DescribeNotebookInstanceResponse = DescribeNotebookInstanceResponse(this)
override fun acceleratorTypes(acceleratorTypes: List): FluentBuilder = apply { this.acceleratorTypes = acceleratorTypes }
override fun additionalCodeRepositories(additionalCodeRepositories: List): FluentBuilder = apply { this.additionalCodeRepositories = additionalCodeRepositories }
override fun creationTime(creationTime: Instant): FluentBuilder = apply { this.creationTime = creationTime }
override fun defaultCodeRepository(defaultCodeRepository: String): FluentBuilder = apply { this.defaultCodeRepository = defaultCodeRepository }
override fun directInternetAccess(directInternetAccess: DirectInternetAccess): FluentBuilder = apply { this.directInternetAccess = directInternetAccess }
override fun failureReason(failureReason: String): FluentBuilder = apply { this.failureReason = failureReason }
override fun instanceType(instanceType: InstanceType): FluentBuilder = apply { this.instanceType = instanceType }
override fun kmsKeyId(kmsKeyId: String): FluentBuilder = apply { this.kmsKeyId = kmsKeyId }
override fun lastModifiedTime(lastModifiedTime: Instant): FluentBuilder = apply { this.lastModifiedTime = lastModifiedTime }
override fun networkInterfaceId(networkInterfaceId: String): FluentBuilder = apply { this.networkInterfaceId = networkInterfaceId }
override fun notebookInstanceArn(notebookInstanceArn: String): FluentBuilder = apply { this.notebookInstanceArn = notebookInstanceArn }
override fun notebookInstanceLifecycleConfigName(notebookInstanceLifecycleConfigName: String): FluentBuilder = apply { this.notebookInstanceLifecycleConfigName = notebookInstanceLifecycleConfigName }
override fun notebookInstanceName(notebookInstanceName: String): FluentBuilder = apply { this.notebookInstanceName = notebookInstanceName }
override fun notebookInstanceStatus(notebookInstanceStatus: NotebookInstanceStatus): FluentBuilder = apply { this.notebookInstanceStatus = notebookInstanceStatus }
override fun platformIdentifier(platformIdentifier: String): FluentBuilder = apply { this.platformIdentifier = platformIdentifier }
override fun roleArn(roleArn: String): FluentBuilder = apply { this.roleArn = roleArn }
override fun rootAccess(rootAccess: RootAccess): FluentBuilder = apply { this.rootAccess = rootAccess }
override fun securityGroups(securityGroups: List): FluentBuilder = apply { this.securityGroups = securityGroups }
override fun subnetId(subnetId: String): FluentBuilder = apply { this.subnetId = subnetId }
override fun url(url: String): FluentBuilder = apply { this.url = url }
override fun volumeSizeInGb(volumeSizeInGb: Int): FluentBuilder = apply { this.volumeSizeInGb = volumeSizeInGb }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy