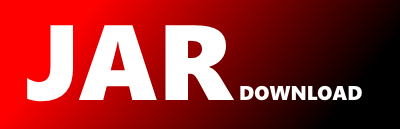
aws.sdk.kotlin.services.sagemaker.model.DescribeUserProfileResponse.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
import aws.smithy.kotlin.runtime.time.Instant
class DescribeUserProfileResponse private constructor(builder: BuilderImpl) {
/**
* The creation time.
*/
val creationTime: Instant? = builder.creationTime
/**
* The ID of the domain that contains the profile.
*/
val domainId: String? = builder.domainId
/**
* The failure reason.
*/
val failureReason: String? = builder.failureReason
/**
* The ID of the user's profile in the Amazon Elastic File System (EFS) volume.
*/
val homeEfsFileSystemUid: String? = builder.homeEfsFileSystemUid
/**
* The last modified time.
*/
val lastModifiedTime: Instant? = builder.lastModifiedTime
/**
* The SSO user identifier.
*/
val singleSignOnUserIdentifier: String? = builder.singleSignOnUserIdentifier
/**
* The SSO user value.
*/
val singleSignOnUserValue: String? = builder.singleSignOnUserValue
/**
* The status.
*/
val status: UserProfileStatus? = builder.status
/**
* The user profile Amazon Resource Name (ARN).
*/
val userProfileArn: String? = builder.userProfileArn
/**
* The user profile name.
*/
val userProfileName: String? = builder.userProfileName
/**
* A collection of settings.
*/
val userSettings: UserSettings? = builder.userSettings
companion object {
@JvmStatic
fun fluentBuilder(): FluentBuilder = BuilderImpl()
internal fun builder(): DslBuilder = BuilderImpl()
operator fun invoke(block: DslBuilder.() -> kotlin.Unit): DescribeUserProfileResponse = BuilderImpl().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeUserProfileResponse(")
append("creationTime=$creationTime,")
append("domainId=$domainId,")
append("failureReason=$failureReason,")
append("homeEfsFileSystemUid=$homeEfsFileSystemUid,")
append("lastModifiedTime=$lastModifiedTime,")
append("singleSignOnUserIdentifier=$singleSignOnUserIdentifier,")
append("singleSignOnUserValue=$singleSignOnUserValue,")
append("status=$status,")
append("userProfileArn=$userProfileArn,")
append("userProfileName=$userProfileName,")
append("userSettings=$userSettings)")
}
override fun hashCode(): kotlin.Int {
var result = creationTime?.hashCode() ?: 0
result = 31 * result + (domainId?.hashCode() ?: 0)
result = 31 * result + (failureReason?.hashCode() ?: 0)
result = 31 * result + (homeEfsFileSystemUid?.hashCode() ?: 0)
result = 31 * result + (lastModifiedTime?.hashCode() ?: 0)
result = 31 * result + (singleSignOnUserIdentifier?.hashCode() ?: 0)
result = 31 * result + (singleSignOnUserValue?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
result = 31 * result + (userProfileArn?.hashCode() ?: 0)
result = 31 * result + (userProfileName?.hashCode() ?: 0)
result = 31 * result + (userSettings?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as DescribeUserProfileResponse
if (creationTime != other.creationTime) return false
if (domainId != other.domainId) return false
if (failureReason != other.failureReason) return false
if (homeEfsFileSystemUid != other.homeEfsFileSystemUid) return false
if (lastModifiedTime != other.lastModifiedTime) return false
if (singleSignOnUserIdentifier != other.singleSignOnUserIdentifier) return false
if (singleSignOnUserValue != other.singleSignOnUserValue) return false
if (status != other.status) return false
if (userProfileArn != other.userProfileArn) return false
if (userProfileName != other.userProfileName) return false
if (userSettings != other.userSettings) return false
return true
}
fun copy(block: DslBuilder.() -> kotlin.Unit = {}): DescribeUserProfileResponse = BuilderImpl(this).apply(block).build()
interface FluentBuilder {
fun build(): DescribeUserProfileResponse
/**
* The creation time.
*/
fun creationTime(creationTime: Instant): FluentBuilder
/**
* The ID of the domain that contains the profile.
*/
fun domainId(domainId: String): FluentBuilder
/**
* The failure reason.
*/
fun failureReason(failureReason: String): FluentBuilder
/**
* The ID of the user's profile in the Amazon Elastic File System (EFS) volume.
*/
fun homeEfsFileSystemUid(homeEfsFileSystemUid: String): FluentBuilder
/**
* The last modified time.
*/
fun lastModifiedTime(lastModifiedTime: Instant): FluentBuilder
/**
* The SSO user identifier.
*/
fun singleSignOnUserIdentifier(singleSignOnUserIdentifier: String): FluentBuilder
/**
* The SSO user value.
*/
fun singleSignOnUserValue(singleSignOnUserValue: String): FluentBuilder
/**
* The status.
*/
fun status(status: UserProfileStatus): FluentBuilder
/**
* The user profile Amazon Resource Name (ARN).
*/
fun userProfileArn(userProfileArn: String): FluentBuilder
/**
* The user profile name.
*/
fun userProfileName(userProfileName: String): FluentBuilder
/**
* A collection of settings.
*/
fun userSettings(userSettings: UserSettings): FluentBuilder
}
interface DslBuilder {
/**
* The creation time.
*/
var creationTime: Instant?
/**
* The ID of the domain that contains the profile.
*/
var domainId: String?
/**
* The failure reason.
*/
var failureReason: String?
/**
* The ID of the user's profile in the Amazon Elastic File System (EFS) volume.
*/
var homeEfsFileSystemUid: String?
/**
* The last modified time.
*/
var lastModifiedTime: Instant?
/**
* The SSO user identifier.
*/
var singleSignOnUserIdentifier: String?
/**
* The SSO user value.
*/
var singleSignOnUserValue: String?
/**
* The status.
*/
var status: UserProfileStatus?
/**
* The user profile Amazon Resource Name (ARN).
*/
var userProfileArn: String?
/**
* The user profile name.
*/
var userProfileName: String?
/**
* A collection of settings.
*/
var userSettings: UserSettings?
fun build(): DescribeUserProfileResponse
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.UserSettings] inside the given [block]
*/
fun userSettings(block: UserSettings.DslBuilder.() -> kotlin.Unit) {
this.userSettings = UserSettings.invoke(block)
}
}
private class BuilderImpl() : FluentBuilder, DslBuilder {
override var creationTime: Instant? = null
override var domainId: String? = null
override var failureReason: String? = null
override var homeEfsFileSystemUid: String? = null
override var lastModifiedTime: Instant? = null
override var singleSignOnUserIdentifier: String? = null
override var singleSignOnUserValue: String? = null
override var status: UserProfileStatus? = null
override var userProfileArn: String? = null
override var userProfileName: String? = null
override var userSettings: UserSettings? = null
constructor(x: DescribeUserProfileResponse) : this() {
this.creationTime = x.creationTime
this.domainId = x.domainId
this.failureReason = x.failureReason
this.homeEfsFileSystemUid = x.homeEfsFileSystemUid
this.lastModifiedTime = x.lastModifiedTime
this.singleSignOnUserIdentifier = x.singleSignOnUserIdentifier
this.singleSignOnUserValue = x.singleSignOnUserValue
this.status = x.status
this.userProfileArn = x.userProfileArn
this.userProfileName = x.userProfileName
this.userSettings = x.userSettings
}
override fun build(): DescribeUserProfileResponse = DescribeUserProfileResponse(this)
override fun creationTime(creationTime: Instant): FluentBuilder = apply { this.creationTime = creationTime }
override fun domainId(domainId: String): FluentBuilder = apply { this.domainId = domainId }
override fun failureReason(failureReason: String): FluentBuilder = apply { this.failureReason = failureReason }
override fun homeEfsFileSystemUid(homeEfsFileSystemUid: String): FluentBuilder = apply { this.homeEfsFileSystemUid = homeEfsFileSystemUid }
override fun lastModifiedTime(lastModifiedTime: Instant): FluentBuilder = apply { this.lastModifiedTime = lastModifiedTime }
override fun singleSignOnUserIdentifier(singleSignOnUserIdentifier: String): FluentBuilder = apply { this.singleSignOnUserIdentifier = singleSignOnUserIdentifier }
override fun singleSignOnUserValue(singleSignOnUserValue: String): FluentBuilder = apply { this.singleSignOnUserValue = singleSignOnUserValue }
override fun status(status: UserProfileStatus): FluentBuilder = apply { this.status = status }
override fun userProfileArn(userProfileArn: String): FluentBuilder = apply { this.userProfileArn = userProfileArn }
override fun userProfileName(userProfileName: String): FluentBuilder = apply { this.userProfileName = userProfileName }
override fun userSettings(userSettings: UserSettings): FluentBuilder = apply { this.userSettings = userSettings }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy