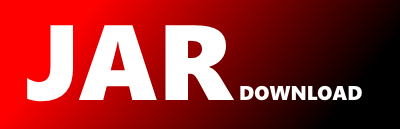
aws.sdk.kotlin.services.sagemaker.model.EdgeModelStat.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
/**
* Status of edge devices with this model.
*/
class EdgeModelStat private constructor(builder: BuilderImpl) {
/**
* The number of devices that have this model version, a heart beat, and are currently running.
*/
val activeDeviceCount: Long = builder.activeDeviceCount
/**
* The number of devices that have this model version and have a heart beat.
*/
val connectedDeviceCount: Long = builder.connectedDeviceCount
/**
* The name of the model.
*/
val modelName: String? = builder.modelName
/**
* The model version.
*/
val modelVersion: String? = builder.modelVersion
/**
* The number of devices that have this model version and do not have a heart beat.
*/
val offlineDeviceCount: Long = builder.offlineDeviceCount
/**
* The number of devices with this model version and are producing sample data.
*/
val samplingDeviceCount: Long = builder.samplingDeviceCount
companion object {
@JvmStatic
fun fluentBuilder(): FluentBuilder = BuilderImpl()
internal fun builder(): DslBuilder = BuilderImpl()
operator fun invoke(block: DslBuilder.() -> kotlin.Unit): EdgeModelStat = BuilderImpl().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("EdgeModelStat(")
append("activeDeviceCount=$activeDeviceCount,")
append("connectedDeviceCount=$connectedDeviceCount,")
append("modelName=$modelName,")
append("modelVersion=$modelVersion,")
append("offlineDeviceCount=$offlineDeviceCount,")
append("samplingDeviceCount=$samplingDeviceCount)")
}
override fun hashCode(): kotlin.Int {
var result = activeDeviceCount.hashCode()
result = 31 * result + (connectedDeviceCount.hashCode())
result = 31 * result + (modelName?.hashCode() ?: 0)
result = 31 * result + (modelVersion?.hashCode() ?: 0)
result = 31 * result + (offlineDeviceCount.hashCode())
result = 31 * result + (samplingDeviceCount.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as EdgeModelStat
if (activeDeviceCount != other.activeDeviceCount) return false
if (connectedDeviceCount != other.connectedDeviceCount) return false
if (modelName != other.modelName) return false
if (modelVersion != other.modelVersion) return false
if (offlineDeviceCount != other.offlineDeviceCount) return false
if (samplingDeviceCount != other.samplingDeviceCount) return false
return true
}
fun copy(block: DslBuilder.() -> kotlin.Unit = {}): EdgeModelStat = BuilderImpl(this).apply(block).build()
interface FluentBuilder {
fun build(): EdgeModelStat
/**
* The number of devices that have this model version, a heart beat, and are currently running.
*/
fun activeDeviceCount(activeDeviceCount: Long): FluentBuilder
/**
* The number of devices that have this model version and have a heart beat.
*/
fun connectedDeviceCount(connectedDeviceCount: Long): FluentBuilder
/**
* The name of the model.
*/
fun modelName(modelName: String): FluentBuilder
/**
* The model version.
*/
fun modelVersion(modelVersion: String): FluentBuilder
/**
* The number of devices that have this model version and do not have a heart beat.
*/
fun offlineDeviceCount(offlineDeviceCount: Long): FluentBuilder
/**
* The number of devices with this model version and are producing sample data.
*/
fun samplingDeviceCount(samplingDeviceCount: Long): FluentBuilder
}
interface DslBuilder {
/**
* The number of devices that have this model version, a heart beat, and are currently running.
*/
var activeDeviceCount: Long
/**
* The number of devices that have this model version and have a heart beat.
*/
var connectedDeviceCount: Long
/**
* The name of the model.
*/
var modelName: String?
/**
* The model version.
*/
var modelVersion: String?
/**
* The number of devices that have this model version and do not have a heart beat.
*/
var offlineDeviceCount: Long
/**
* The number of devices with this model version and are producing sample data.
*/
var samplingDeviceCount: Long
fun build(): EdgeModelStat
}
private class BuilderImpl() : FluentBuilder, DslBuilder {
override var activeDeviceCount: Long = 0L
override var connectedDeviceCount: Long = 0L
override var modelName: String? = null
override var modelVersion: String? = null
override var offlineDeviceCount: Long = 0L
override var samplingDeviceCount: Long = 0L
constructor(x: EdgeModelStat) : this() {
this.activeDeviceCount = x.activeDeviceCount
this.connectedDeviceCount = x.connectedDeviceCount
this.modelName = x.modelName
this.modelVersion = x.modelVersion
this.offlineDeviceCount = x.offlineDeviceCount
this.samplingDeviceCount = x.samplingDeviceCount
}
override fun build(): EdgeModelStat = EdgeModelStat(this)
override fun activeDeviceCount(activeDeviceCount: Long): FluentBuilder = apply { this.activeDeviceCount = activeDeviceCount }
override fun connectedDeviceCount(connectedDeviceCount: Long): FluentBuilder = apply { this.connectedDeviceCount = connectedDeviceCount }
override fun modelName(modelName: String): FluentBuilder = apply { this.modelName = modelName }
override fun modelVersion(modelVersion: String): FluentBuilder = apply { this.modelVersion = modelVersion }
override fun offlineDeviceCount(offlineDeviceCount: Long): FluentBuilder = apply { this.offlineDeviceCount = offlineDeviceCount }
override fun samplingDeviceCount(samplingDeviceCount: Long): FluentBuilder = apply { this.samplingDeviceCount = samplingDeviceCount }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy