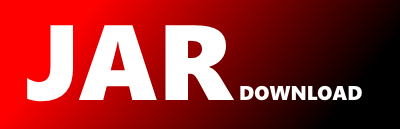
aws.sdk.kotlin.services.sagemaker.model.FeatureDefinition.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
/**
* A list of features. You must include FeatureName and
* FeatureType. Valid feature FeatureTypes are
* Integral, Fractional and String.
*/
class FeatureDefinition private constructor(builder: BuilderImpl) {
/**
* The name of a feature. The type must be a string. FeatureName cannot be any
* of the following: is_deleted, write_time,
* api_invocation_time.
*/
val featureName: String? = builder.featureName
/**
* The value type of a feature. Valid values are Integral, Fractional, or String.
*/
val featureType: FeatureType? = builder.featureType
companion object {
@JvmStatic
fun fluentBuilder(): FluentBuilder = BuilderImpl()
internal fun builder(): DslBuilder = BuilderImpl()
operator fun invoke(block: DslBuilder.() -> kotlin.Unit): FeatureDefinition = BuilderImpl().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("FeatureDefinition(")
append("featureName=$featureName,")
append("featureType=$featureType)")
}
override fun hashCode(): kotlin.Int {
var result = featureName?.hashCode() ?: 0
result = 31 * result + (featureType?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as FeatureDefinition
if (featureName != other.featureName) return false
if (featureType != other.featureType) return false
return true
}
fun copy(block: DslBuilder.() -> kotlin.Unit = {}): FeatureDefinition = BuilderImpl(this).apply(block).build()
interface FluentBuilder {
fun build(): FeatureDefinition
/**
* The name of a feature. The type must be a string. FeatureName cannot be any
* of the following: is_deleted, write_time,
* api_invocation_time.
*/
fun featureName(featureName: String): FluentBuilder
/**
* The value type of a feature. Valid values are Integral, Fractional, or String.
*/
fun featureType(featureType: FeatureType): FluentBuilder
}
interface DslBuilder {
/**
* The name of a feature. The type must be a string. FeatureName cannot be any
* of the following: is_deleted, write_time,
* api_invocation_time.
*/
var featureName: String?
/**
* The value type of a feature. Valid values are Integral, Fractional, or String.
*/
var featureType: FeatureType?
fun build(): FeatureDefinition
}
private class BuilderImpl() : FluentBuilder, DslBuilder {
override var featureName: String? = null
override var featureType: FeatureType? = null
constructor(x: FeatureDefinition) : this() {
this.featureName = x.featureName
this.featureType = x.featureType
}
override fun build(): FeatureDefinition = FeatureDefinition(this)
override fun featureName(featureName: String): FluentBuilder = apply { this.featureName = featureName }
override fun featureType(featureType: FeatureType): FluentBuilder = apply { this.featureType = featureType }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy