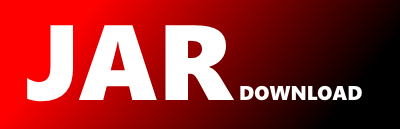
aws.sdk.kotlin.services.sagemaker.model.LabelingJobDataSource.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
/**
* Provides information about the location of input data.
* You must specify at least one of the following: S3DataSource or SnsDataSource.
* Use SnsDataSource to specify an SNS input topic
* for a streaming labeling job. If you do not specify
* and SNS input topic ARN, Ground Truth will create a one-time labeling job.
* Use S3DataSource to specify an input
* manifest file for both streaming and one-time labeling jobs.
* Adding an S3DataSource is optional if you use SnsDataSource to create a streaming labeling job.
*/
class LabelingJobDataSource private constructor(builder: BuilderImpl) {
/**
* The Amazon S3 location of the input data objects.
*/
val s3DataSource: LabelingJobS3DataSource? = builder.s3DataSource
/**
* An Amazon SNS data source used for streaming labeling jobs. To learn more, see Send Data to a Streaming Labeling Job.
*/
val snsDataSource: LabelingJobSnsDataSource? = builder.snsDataSource
companion object {
@JvmStatic
fun fluentBuilder(): FluentBuilder = BuilderImpl()
internal fun builder(): DslBuilder = BuilderImpl()
operator fun invoke(block: DslBuilder.() -> kotlin.Unit): LabelingJobDataSource = BuilderImpl().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("LabelingJobDataSource(")
append("s3DataSource=$s3DataSource,")
append("snsDataSource=$snsDataSource)")
}
override fun hashCode(): kotlin.Int {
var result = s3DataSource?.hashCode() ?: 0
result = 31 * result + (snsDataSource?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as LabelingJobDataSource
if (s3DataSource != other.s3DataSource) return false
if (snsDataSource != other.snsDataSource) return false
return true
}
fun copy(block: DslBuilder.() -> kotlin.Unit = {}): LabelingJobDataSource = BuilderImpl(this).apply(block).build()
interface FluentBuilder {
fun build(): LabelingJobDataSource
/**
* The Amazon S3 location of the input data objects.
*/
fun s3DataSource(s3DataSource: LabelingJobS3DataSource): FluentBuilder
/**
* An Amazon SNS data source used for streaming labeling jobs. To learn more, see Send Data to a Streaming Labeling Job.
*/
fun snsDataSource(snsDataSource: LabelingJobSnsDataSource): FluentBuilder
}
interface DslBuilder {
/**
* The Amazon S3 location of the input data objects.
*/
var s3DataSource: LabelingJobS3DataSource?
/**
* An Amazon SNS data source used for streaming labeling jobs. To learn more, see Send Data to a Streaming Labeling Job.
*/
var snsDataSource: LabelingJobSnsDataSource?
fun build(): LabelingJobDataSource
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.LabelingJobS3DataSource] inside the given [block]
*/
fun s3DataSource(block: LabelingJobS3DataSource.DslBuilder.() -> kotlin.Unit) {
this.s3DataSource = LabelingJobS3DataSource.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.LabelingJobSnsDataSource] inside the given [block]
*/
fun snsDataSource(block: LabelingJobSnsDataSource.DslBuilder.() -> kotlin.Unit) {
this.snsDataSource = LabelingJobSnsDataSource.invoke(block)
}
}
private class BuilderImpl() : FluentBuilder, DslBuilder {
override var s3DataSource: LabelingJobS3DataSource? = null
override var snsDataSource: LabelingJobSnsDataSource? = null
constructor(x: LabelingJobDataSource) : this() {
this.s3DataSource = x.s3DataSource
this.snsDataSource = x.snsDataSource
}
override fun build(): LabelingJobDataSource = LabelingJobDataSource(this)
override fun s3DataSource(s3DataSource: LabelingJobS3DataSource): FluentBuilder = apply { this.s3DataSource = s3DataSource }
override fun snsDataSource(snsDataSource: LabelingJobSnsDataSource): FluentBuilder = apply { this.snsDataSource = snsDataSource }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy