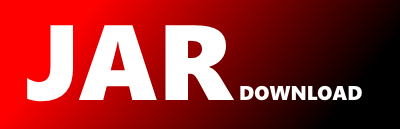
aws.sdk.kotlin.services.sagemaker.model.ListModelsRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
import aws.smithy.kotlin.runtime.time.Instant
class ListModelsRequest private constructor(builder: BuilderImpl) {
/**
* A filter that returns only models with a creation time greater than or equal to the
* specified time (timestamp).
*/
val creationTimeAfter: Instant? = builder.creationTimeAfter
/**
* A filter that returns only models created before the specified time
* (timestamp).
*/
val creationTimeBefore: Instant? = builder.creationTimeBefore
/**
* The maximum number of models to return in the response.
*/
val maxResults: Int? = builder.maxResults
/**
* A string in the model name. This filter returns only models whose
* name contains the specified string.
*/
val nameContains: String? = builder.nameContains
/**
* If the response to a previous ListModels request was truncated, the
* response includes a NextToken. To retrieve the next set of models, use the
* token in the next request.
*/
val nextToken: String? = builder.nextToken
/**
* Sorts the list of results. The default is CreationTime.
*/
val sortBy: ModelSortKey? = builder.sortBy
/**
* The sort order for results. The default is Descending.
*/
val sortOrder: OrderKey? = builder.sortOrder
companion object {
@JvmStatic
fun fluentBuilder(): FluentBuilder = BuilderImpl()
internal fun builder(): DslBuilder = BuilderImpl()
operator fun invoke(block: DslBuilder.() -> kotlin.Unit): ListModelsRequest = BuilderImpl().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ListModelsRequest(")
append("creationTimeAfter=$creationTimeAfter,")
append("creationTimeBefore=$creationTimeBefore,")
append("maxResults=$maxResults,")
append("nameContains=$nameContains,")
append("nextToken=$nextToken,")
append("sortBy=$sortBy,")
append("sortOrder=$sortOrder)")
}
override fun hashCode(): kotlin.Int {
var result = creationTimeAfter?.hashCode() ?: 0
result = 31 * result + (creationTimeBefore?.hashCode() ?: 0)
result = 31 * result + (maxResults ?: 0)
result = 31 * result + (nameContains?.hashCode() ?: 0)
result = 31 * result + (nextToken?.hashCode() ?: 0)
result = 31 * result + (sortBy?.hashCode() ?: 0)
result = 31 * result + (sortOrder?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as ListModelsRequest
if (creationTimeAfter != other.creationTimeAfter) return false
if (creationTimeBefore != other.creationTimeBefore) return false
if (maxResults != other.maxResults) return false
if (nameContains != other.nameContains) return false
if (nextToken != other.nextToken) return false
if (sortBy != other.sortBy) return false
if (sortOrder != other.sortOrder) return false
return true
}
fun copy(block: DslBuilder.() -> kotlin.Unit = {}): ListModelsRequest = BuilderImpl(this).apply(block).build()
interface FluentBuilder {
fun build(): ListModelsRequest
/**
* A filter that returns only models with a creation time greater than or equal to the
* specified time (timestamp).
*/
fun creationTimeAfter(creationTimeAfter: Instant): FluentBuilder
/**
* A filter that returns only models created before the specified time
* (timestamp).
*/
fun creationTimeBefore(creationTimeBefore: Instant): FluentBuilder
/**
* The maximum number of models to return in the response.
*/
fun maxResults(maxResults: Int): FluentBuilder
/**
* A string in the model name. This filter returns only models whose
* name contains the specified string.
*/
fun nameContains(nameContains: String): FluentBuilder
/**
* If the response to a previous ListModels request was truncated, the
* response includes a NextToken. To retrieve the next set of models, use the
* token in the next request.
*/
fun nextToken(nextToken: String): FluentBuilder
/**
* Sorts the list of results. The default is CreationTime.
*/
fun sortBy(sortBy: ModelSortKey): FluentBuilder
/**
* The sort order for results. The default is Descending.
*/
fun sortOrder(sortOrder: OrderKey): FluentBuilder
}
interface DslBuilder {
/**
* A filter that returns only models with a creation time greater than or equal to the
* specified time (timestamp).
*/
var creationTimeAfter: Instant?
/**
* A filter that returns only models created before the specified time
* (timestamp).
*/
var creationTimeBefore: Instant?
/**
* The maximum number of models to return in the response.
*/
var maxResults: Int?
/**
* A string in the model name. This filter returns only models whose
* name contains the specified string.
*/
var nameContains: String?
/**
* If the response to a previous ListModels request was truncated, the
* response includes a NextToken. To retrieve the next set of models, use the
* token in the next request.
*/
var nextToken: String?
/**
* Sorts the list of results. The default is CreationTime.
*/
var sortBy: ModelSortKey?
/**
* The sort order for results. The default is Descending.
*/
var sortOrder: OrderKey?
fun build(): ListModelsRequest
}
private class BuilderImpl() : FluentBuilder, DslBuilder {
override var creationTimeAfter: Instant? = null
override var creationTimeBefore: Instant? = null
override var maxResults: Int? = null
override var nameContains: String? = null
override var nextToken: String? = null
override var sortBy: ModelSortKey? = null
override var sortOrder: OrderKey? = null
constructor(x: ListModelsRequest) : this() {
this.creationTimeAfter = x.creationTimeAfter
this.creationTimeBefore = x.creationTimeBefore
this.maxResults = x.maxResults
this.nameContains = x.nameContains
this.nextToken = x.nextToken
this.sortBy = x.sortBy
this.sortOrder = x.sortOrder
}
override fun build(): ListModelsRequest = ListModelsRequest(this)
override fun creationTimeAfter(creationTimeAfter: Instant): FluentBuilder = apply { this.creationTimeAfter = creationTimeAfter }
override fun creationTimeBefore(creationTimeBefore: Instant): FluentBuilder = apply { this.creationTimeBefore = creationTimeBefore }
override fun maxResults(maxResults: Int): FluentBuilder = apply { this.maxResults = maxResults }
override fun nameContains(nameContains: String): FluentBuilder = apply { this.nameContains = nameContains }
override fun nextToken(nextToken: String): FluentBuilder = apply { this.nextToken = nextToken }
override fun sortBy(sortBy: ModelSortKey): FluentBuilder = apply { this.sortBy = sortBy }
override fun sortOrder(sortOrder: OrderKey): FluentBuilder = apply { this.sortOrder = sortOrder }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy