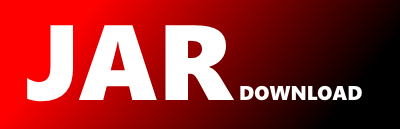
aws.sdk.kotlin.services.sagemaker.model.ListProcessingJobsRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
import aws.smithy.kotlin.runtime.time.Instant
class ListProcessingJobsRequest private constructor(builder: BuilderImpl) {
/**
* A filter that returns only processing jobs created after the specified time.
*/
val creationTimeAfter: Instant? = builder.creationTimeAfter
/**
* A filter that returns only processing jobs created after the specified time.
*/
val creationTimeBefore: Instant? = builder.creationTimeBefore
/**
* A filter that returns only processing jobs modified after the specified time.
*/
val lastModifiedTimeAfter: Instant? = builder.lastModifiedTimeAfter
/**
* A filter that returns only processing jobs modified before the specified time.
*/
val lastModifiedTimeBefore: Instant? = builder.lastModifiedTimeBefore
/**
* The maximum number of processing jobs to return in the response.
*/
val maxResults: Int? = builder.maxResults
/**
* A string in the processing job name. This filter returns only processing jobs whose
* name contains the specified string.
*/
val nameContains: String? = builder.nameContains
/**
* If the result of the previous ListProcessingJobs request was truncated,
* the response includes a NextToken. To retrieve the next set of processing
* jobs, use the token in the next request.
*/
val nextToken: String? = builder.nextToken
/**
* The field to sort results by. The default is CreationTime.
*/
val sortBy: SortBy? = builder.sortBy
/**
* The sort order for results. The default is Ascending.
*/
val sortOrder: SortOrder? = builder.sortOrder
/**
* A filter that retrieves only processing jobs with a specific status.
*/
val statusEquals: ProcessingJobStatus? = builder.statusEquals
companion object {
@JvmStatic
fun fluentBuilder(): FluentBuilder = BuilderImpl()
internal fun builder(): DslBuilder = BuilderImpl()
operator fun invoke(block: DslBuilder.() -> kotlin.Unit): ListProcessingJobsRequest = BuilderImpl().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ListProcessingJobsRequest(")
append("creationTimeAfter=$creationTimeAfter,")
append("creationTimeBefore=$creationTimeBefore,")
append("lastModifiedTimeAfter=$lastModifiedTimeAfter,")
append("lastModifiedTimeBefore=$lastModifiedTimeBefore,")
append("maxResults=$maxResults,")
append("nameContains=$nameContains,")
append("nextToken=$nextToken,")
append("sortBy=$sortBy,")
append("sortOrder=$sortOrder,")
append("statusEquals=$statusEquals)")
}
override fun hashCode(): kotlin.Int {
var result = creationTimeAfter?.hashCode() ?: 0
result = 31 * result + (creationTimeBefore?.hashCode() ?: 0)
result = 31 * result + (lastModifiedTimeAfter?.hashCode() ?: 0)
result = 31 * result + (lastModifiedTimeBefore?.hashCode() ?: 0)
result = 31 * result + (maxResults ?: 0)
result = 31 * result + (nameContains?.hashCode() ?: 0)
result = 31 * result + (nextToken?.hashCode() ?: 0)
result = 31 * result + (sortBy?.hashCode() ?: 0)
result = 31 * result + (sortOrder?.hashCode() ?: 0)
result = 31 * result + (statusEquals?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as ListProcessingJobsRequest
if (creationTimeAfter != other.creationTimeAfter) return false
if (creationTimeBefore != other.creationTimeBefore) return false
if (lastModifiedTimeAfter != other.lastModifiedTimeAfter) return false
if (lastModifiedTimeBefore != other.lastModifiedTimeBefore) return false
if (maxResults != other.maxResults) return false
if (nameContains != other.nameContains) return false
if (nextToken != other.nextToken) return false
if (sortBy != other.sortBy) return false
if (sortOrder != other.sortOrder) return false
if (statusEquals != other.statusEquals) return false
return true
}
fun copy(block: DslBuilder.() -> kotlin.Unit = {}): ListProcessingJobsRequest = BuilderImpl(this).apply(block).build()
interface FluentBuilder {
fun build(): ListProcessingJobsRequest
/**
* A filter that returns only processing jobs created after the specified time.
*/
fun creationTimeAfter(creationTimeAfter: Instant): FluentBuilder
/**
* A filter that returns only processing jobs created after the specified time.
*/
fun creationTimeBefore(creationTimeBefore: Instant): FluentBuilder
/**
* A filter that returns only processing jobs modified after the specified time.
*/
fun lastModifiedTimeAfter(lastModifiedTimeAfter: Instant): FluentBuilder
/**
* A filter that returns only processing jobs modified before the specified time.
*/
fun lastModifiedTimeBefore(lastModifiedTimeBefore: Instant): FluentBuilder
/**
* The maximum number of processing jobs to return in the response.
*/
fun maxResults(maxResults: Int): FluentBuilder
/**
* A string in the processing job name. This filter returns only processing jobs whose
* name contains the specified string.
*/
fun nameContains(nameContains: String): FluentBuilder
/**
* If the result of the previous ListProcessingJobs request was truncated,
* the response includes a NextToken. To retrieve the next set of processing
* jobs, use the token in the next request.
*/
fun nextToken(nextToken: String): FluentBuilder
/**
* The field to sort results by. The default is CreationTime.
*/
fun sortBy(sortBy: SortBy): FluentBuilder
/**
* The sort order for results. The default is Ascending.
*/
fun sortOrder(sortOrder: SortOrder): FluentBuilder
/**
* A filter that retrieves only processing jobs with a specific status.
*/
fun statusEquals(statusEquals: ProcessingJobStatus): FluentBuilder
}
interface DslBuilder {
/**
* A filter that returns only processing jobs created after the specified time.
*/
var creationTimeAfter: Instant?
/**
* A filter that returns only processing jobs created after the specified time.
*/
var creationTimeBefore: Instant?
/**
* A filter that returns only processing jobs modified after the specified time.
*/
var lastModifiedTimeAfter: Instant?
/**
* A filter that returns only processing jobs modified before the specified time.
*/
var lastModifiedTimeBefore: Instant?
/**
* The maximum number of processing jobs to return in the response.
*/
var maxResults: Int?
/**
* A string in the processing job name. This filter returns only processing jobs whose
* name contains the specified string.
*/
var nameContains: String?
/**
* If the result of the previous ListProcessingJobs request was truncated,
* the response includes a NextToken. To retrieve the next set of processing
* jobs, use the token in the next request.
*/
var nextToken: String?
/**
* The field to sort results by. The default is CreationTime.
*/
var sortBy: SortBy?
/**
* The sort order for results. The default is Ascending.
*/
var sortOrder: SortOrder?
/**
* A filter that retrieves only processing jobs with a specific status.
*/
var statusEquals: ProcessingJobStatus?
fun build(): ListProcessingJobsRequest
}
private class BuilderImpl() : FluentBuilder, DslBuilder {
override var creationTimeAfter: Instant? = null
override var creationTimeBefore: Instant? = null
override var lastModifiedTimeAfter: Instant? = null
override var lastModifiedTimeBefore: Instant? = null
override var maxResults: Int? = null
override var nameContains: String? = null
override var nextToken: String? = null
override var sortBy: SortBy? = null
override var sortOrder: SortOrder? = null
override var statusEquals: ProcessingJobStatus? = null
constructor(x: ListProcessingJobsRequest) : this() {
this.creationTimeAfter = x.creationTimeAfter
this.creationTimeBefore = x.creationTimeBefore
this.lastModifiedTimeAfter = x.lastModifiedTimeAfter
this.lastModifiedTimeBefore = x.lastModifiedTimeBefore
this.maxResults = x.maxResults
this.nameContains = x.nameContains
this.nextToken = x.nextToken
this.sortBy = x.sortBy
this.sortOrder = x.sortOrder
this.statusEquals = x.statusEquals
}
override fun build(): ListProcessingJobsRequest = ListProcessingJobsRequest(this)
override fun creationTimeAfter(creationTimeAfter: Instant): FluentBuilder = apply { this.creationTimeAfter = creationTimeAfter }
override fun creationTimeBefore(creationTimeBefore: Instant): FluentBuilder = apply { this.creationTimeBefore = creationTimeBefore }
override fun lastModifiedTimeAfter(lastModifiedTimeAfter: Instant): FluentBuilder = apply { this.lastModifiedTimeAfter = lastModifiedTimeAfter }
override fun lastModifiedTimeBefore(lastModifiedTimeBefore: Instant): FluentBuilder = apply { this.lastModifiedTimeBefore = lastModifiedTimeBefore }
override fun maxResults(maxResults: Int): FluentBuilder = apply { this.maxResults = maxResults }
override fun nameContains(nameContains: String): FluentBuilder = apply { this.nameContains = nameContains }
override fun nextToken(nextToken: String): FluentBuilder = apply { this.nextToken = nextToken }
override fun sortBy(sortBy: SortBy): FluentBuilder = apply { this.sortBy = sortBy }
override fun sortOrder(sortOrder: SortOrder): FluentBuilder = apply { this.sortOrder = sortOrder }
override fun statusEquals(statusEquals: ProcessingJobStatus): FluentBuilder = apply { this.statusEquals = statusEquals }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy