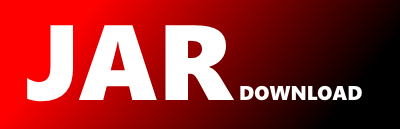
aws.sdk.kotlin.services.sagemaker.model.OnlineStoreConfig.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
/**
* Use this to specify the Amazon Web Services Key Management Service (KMS) Key ID, or
* KMSKeyId, for at rest data encryption. You can turn
* OnlineStore on or off by specifying the EnableOnlineStore flag
* at General Assembly; the default value is False.
*/
class OnlineStoreConfig private constructor(builder: BuilderImpl) {
/**
* Turn OnlineStore off by specifying False
* for the EnableOnlineStore flag. Turn OnlineStore
* on by specifying True
* for the EnableOnlineStore flag.
* The default value is False.
*/
val enableOnlineStore: Boolean = builder.enableOnlineStore
/**
* Use to specify KMS Key ID (KMSKeyId) for at-rest encryption of your
* OnlineStore.
*/
val securityConfig: OnlineStoreSecurityConfig? = builder.securityConfig
companion object {
@JvmStatic
fun fluentBuilder(): FluentBuilder = BuilderImpl()
internal fun builder(): DslBuilder = BuilderImpl()
operator fun invoke(block: DslBuilder.() -> kotlin.Unit): OnlineStoreConfig = BuilderImpl().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("OnlineStoreConfig(")
append("enableOnlineStore=$enableOnlineStore,")
append("securityConfig=$securityConfig)")
}
override fun hashCode(): kotlin.Int {
var result = enableOnlineStore.hashCode()
result = 31 * result + (securityConfig?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as OnlineStoreConfig
if (enableOnlineStore != other.enableOnlineStore) return false
if (securityConfig != other.securityConfig) return false
return true
}
fun copy(block: DslBuilder.() -> kotlin.Unit = {}): OnlineStoreConfig = BuilderImpl(this).apply(block).build()
interface FluentBuilder {
fun build(): OnlineStoreConfig
/**
* Turn OnlineStore off by specifying False
* for the EnableOnlineStore flag. Turn OnlineStore
* on by specifying True
* for the EnableOnlineStore flag.
* The default value is False.
*/
fun enableOnlineStore(enableOnlineStore: Boolean): FluentBuilder
/**
* Use to specify KMS Key ID (KMSKeyId) for at-rest encryption of your
* OnlineStore.
*/
fun securityConfig(securityConfig: OnlineStoreSecurityConfig): FluentBuilder
}
interface DslBuilder {
/**
* Turn OnlineStore off by specifying False
* for the EnableOnlineStore flag. Turn OnlineStore
* on by specifying True
* for the EnableOnlineStore flag.
* The default value is False.
*/
var enableOnlineStore: Boolean
/**
* Use to specify KMS Key ID (KMSKeyId) for at-rest encryption of your
* OnlineStore.
*/
var securityConfig: OnlineStoreSecurityConfig?
fun build(): OnlineStoreConfig
/**
* construct an [aws.sdk.kotlin.services.sagemaker.model.OnlineStoreSecurityConfig] inside the given [block]
*/
fun securityConfig(block: OnlineStoreSecurityConfig.DslBuilder.() -> kotlin.Unit) {
this.securityConfig = OnlineStoreSecurityConfig.invoke(block)
}
}
private class BuilderImpl() : FluentBuilder, DslBuilder {
override var enableOnlineStore: Boolean = false
override var securityConfig: OnlineStoreSecurityConfig? = null
constructor(x: OnlineStoreConfig) : this() {
this.enableOnlineStore = x.enableOnlineStore
this.securityConfig = x.securityConfig
}
override fun build(): OnlineStoreConfig = OnlineStoreConfig(this)
override fun enableOnlineStore(enableOnlineStore: Boolean): FluentBuilder = apply { this.enableOnlineStore = enableOnlineStore }
override fun securityConfig(securityConfig: OnlineStoreSecurityConfig): FluentBuilder = apply { this.securityConfig = securityConfig }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy