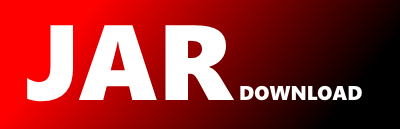
aws.sdk.kotlin.services.sagemaker.model.OutputParameter.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
/**
* An output parameter of a pipeline step.
*/
class OutputParameter private constructor(builder: BuilderImpl) {
/**
* The name of the output parameter.
*/
val name: String? = builder.name
/**
* The value of the output parameter.
*/
val value: String? = builder.value
companion object {
@JvmStatic
fun fluentBuilder(): FluentBuilder = BuilderImpl()
internal fun builder(): DslBuilder = BuilderImpl()
operator fun invoke(block: DslBuilder.() -> kotlin.Unit): OutputParameter = BuilderImpl().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("OutputParameter(")
append("name=$name,")
append("value=$value)")
}
override fun hashCode(): kotlin.Int {
var result = name?.hashCode() ?: 0
result = 31 * result + (value?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as OutputParameter
if (name != other.name) return false
if (value != other.value) return false
return true
}
fun copy(block: DslBuilder.() -> kotlin.Unit = {}): OutputParameter = BuilderImpl(this).apply(block).build()
interface FluentBuilder {
fun build(): OutputParameter
/**
* The name of the output parameter.
*/
fun name(name: String): FluentBuilder
/**
* The value of the output parameter.
*/
fun value(value: String): FluentBuilder
}
interface DslBuilder {
/**
* The name of the output parameter.
*/
var name: String?
/**
* The value of the output parameter.
*/
var value: String?
fun build(): OutputParameter
}
private class BuilderImpl() : FluentBuilder, DslBuilder {
override var name: String? = null
override var value: String? = null
constructor(x: OutputParameter) : this() {
this.name = x.name
this.value = x.value
}
override fun build(): OutputParameter = OutputParameter(this)
override fun name(name: String): FluentBuilder = apply { this.name = name }
override fun value(value: String): FluentBuilder = apply { this.value = value }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy