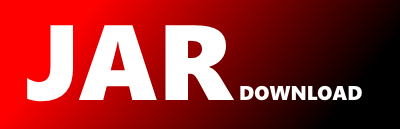
aws.sdk.kotlin.services.sagemaker.model.ProjectSummary.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* Information about a project.
*/
class ProjectSummary private constructor(builder: BuilderImpl) {
/**
* The time that the project was created.
*/
val creationTime: Instant? = builder.creationTime
/**
* The Amazon Resource Name (ARN) of the project.
*/
val projectArn: String? = builder.projectArn
/**
* The description of the project.
*/
val projectDescription: String? = builder.projectDescription
/**
* The ID of the project.
*/
val projectId: String? = builder.projectId
/**
* The name of the project.
*/
val projectName: String? = builder.projectName
/**
* The status of the project.
*/
val projectStatus: ProjectStatus? = builder.projectStatus
companion object {
@JvmStatic
fun fluentBuilder(): FluentBuilder = BuilderImpl()
internal fun builder(): DslBuilder = BuilderImpl()
operator fun invoke(block: DslBuilder.() -> kotlin.Unit): ProjectSummary = BuilderImpl().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ProjectSummary(")
append("creationTime=$creationTime,")
append("projectArn=$projectArn,")
append("projectDescription=$projectDescription,")
append("projectId=$projectId,")
append("projectName=$projectName,")
append("projectStatus=$projectStatus)")
}
override fun hashCode(): kotlin.Int {
var result = creationTime?.hashCode() ?: 0
result = 31 * result + (projectArn?.hashCode() ?: 0)
result = 31 * result + (projectDescription?.hashCode() ?: 0)
result = 31 * result + (projectId?.hashCode() ?: 0)
result = 31 * result + (projectName?.hashCode() ?: 0)
result = 31 * result + (projectStatus?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as ProjectSummary
if (creationTime != other.creationTime) return false
if (projectArn != other.projectArn) return false
if (projectDescription != other.projectDescription) return false
if (projectId != other.projectId) return false
if (projectName != other.projectName) return false
if (projectStatus != other.projectStatus) return false
return true
}
fun copy(block: DslBuilder.() -> kotlin.Unit = {}): ProjectSummary = BuilderImpl(this).apply(block).build()
interface FluentBuilder {
fun build(): ProjectSummary
/**
* The time that the project was created.
*/
fun creationTime(creationTime: Instant): FluentBuilder
/**
* The Amazon Resource Name (ARN) of the project.
*/
fun projectArn(projectArn: String): FluentBuilder
/**
* The description of the project.
*/
fun projectDescription(projectDescription: String): FluentBuilder
/**
* The ID of the project.
*/
fun projectId(projectId: String): FluentBuilder
/**
* The name of the project.
*/
fun projectName(projectName: String): FluentBuilder
/**
* The status of the project.
*/
fun projectStatus(projectStatus: ProjectStatus): FluentBuilder
}
interface DslBuilder {
/**
* The time that the project was created.
*/
var creationTime: Instant?
/**
* The Amazon Resource Name (ARN) of the project.
*/
var projectArn: String?
/**
* The description of the project.
*/
var projectDescription: String?
/**
* The ID of the project.
*/
var projectId: String?
/**
* The name of the project.
*/
var projectName: String?
/**
* The status of the project.
*/
var projectStatus: ProjectStatus?
fun build(): ProjectSummary
}
private class BuilderImpl() : FluentBuilder, DslBuilder {
override var creationTime: Instant? = null
override var projectArn: String? = null
override var projectDescription: String? = null
override var projectId: String? = null
override var projectName: String? = null
override var projectStatus: ProjectStatus? = null
constructor(x: ProjectSummary) : this() {
this.creationTime = x.creationTime
this.projectArn = x.projectArn
this.projectDescription = x.projectDescription
this.projectId = x.projectId
this.projectName = x.projectName
this.projectStatus = x.projectStatus
}
override fun build(): ProjectSummary = ProjectSummary(this)
override fun creationTime(creationTime: Instant): FluentBuilder = apply { this.creationTime = creationTime }
override fun projectArn(projectArn: String): FluentBuilder = apply { this.projectArn = projectArn }
override fun projectDescription(projectDescription: String): FluentBuilder = apply { this.projectDescription = projectDescription }
override fun projectId(projectId: String): FluentBuilder = apply { this.projectId = projectId }
override fun projectName(projectName: String): FluentBuilder = apply { this.projectName = projectName }
override fun projectStatus(projectStatus: ProjectStatus): FluentBuilder = apply { this.projectStatus = projectStatus }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy