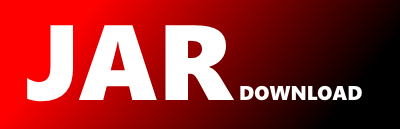
aws.sdk.kotlin.services.sagemaker.model.ServiceCatalogProvisioningDetails.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
/**
* Details that you specify to provision a service catalog product. For information about
* service catalog, see What is Amazon Web Services Service
* Catalog.
*/
class ServiceCatalogProvisioningDetails private constructor(builder: BuilderImpl) {
/**
* The path identifier of the product. This value is optional if the product has a default path, and required if the product has more than one path.
*/
val pathId: String? = builder.pathId
/**
* The ID of the product to provision.
*/
val productId: String? = builder.productId
/**
* The ID of the provisioning artifact.
*/
val provisioningArtifactId: String? = builder.provisioningArtifactId
/**
* A list of key value pairs that you specify when you provision a product.
*/
val provisioningParameters: List? = builder.provisioningParameters
companion object {
@JvmStatic
fun fluentBuilder(): FluentBuilder = BuilderImpl()
internal fun builder(): DslBuilder = BuilderImpl()
operator fun invoke(block: DslBuilder.() -> kotlin.Unit): ServiceCatalogProvisioningDetails = BuilderImpl().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ServiceCatalogProvisioningDetails(")
append("pathId=$pathId,")
append("productId=$productId,")
append("provisioningArtifactId=$provisioningArtifactId,")
append("provisioningParameters=$provisioningParameters)")
}
override fun hashCode(): kotlin.Int {
var result = pathId?.hashCode() ?: 0
result = 31 * result + (productId?.hashCode() ?: 0)
result = 31 * result + (provisioningArtifactId?.hashCode() ?: 0)
result = 31 * result + (provisioningParameters?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as ServiceCatalogProvisioningDetails
if (pathId != other.pathId) return false
if (productId != other.productId) return false
if (provisioningArtifactId != other.provisioningArtifactId) return false
if (provisioningParameters != other.provisioningParameters) return false
return true
}
fun copy(block: DslBuilder.() -> kotlin.Unit = {}): ServiceCatalogProvisioningDetails = BuilderImpl(this).apply(block).build()
interface FluentBuilder {
fun build(): ServiceCatalogProvisioningDetails
/**
* The path identifier of the product. This value is optional if the product has a default path, and required if the product has more than one path.
*/
fun pathId(pathId: String): FluentBuilder
/**
* The ID of the product to provision.
*/
fun productId(productId: String): FluentBuilder
/**
* The ID of the provisioning artifact.
*/
fun provisioningArtifactId(provisioningArtifactId: String): FluentBuilder
/**
* A list of key value pairs that you specify when you provision a product.
*/
fun provisioningParameters(provisioningParameters: List): FluentBuilder
}
interface DslBuilder {
/**
* The path identifier of the product. This value is optional if the product has a default path, and required if the product has more than one path.
*/
var pathId: String?
/**
* The ID of the product to provision.
*/
var productId: String?
/**
* The ID of the provisioning artifact.
*/
var provisioningArtifactId: String?
/**
* A list of key value pairs that you specify when you provision a product.
*/
var provisioningParameters: List?
fun build(): ServiceCatalogProvisioningDetails
}
private class BuilderImpl() : FluentBuilder, DslBuilder {
override var pathId: String? = null
override var productId: String? = null
override var provisioningArtifactId: String? = null
override var provisioningParameters: List? = null
constructor(x: ServiceCatalogProvisioningDetails) : this() {
this.pathId = x.pathId
this.productId = x.productId
this.provisioningArtifactId = x.provisioningArtifactId
this.provisioningParameters = x.provisioningParameters
}
override fun build(): ServiceCatalogProvisioningDetails = ServiceCatalogProvisioningDetails(this)
override fun pathId(pathId: String): FluentBuilder = apply { this.pathId = pathId }
override fun productId(productId: String): FluentBuilder = apply { this.productId = productId }
override fun provisioningArtifactId(provisioningArtifactId: String): FluentBuilder = apply { this.provisioningArtifactId = provisioningArtifactId }
override fun provisioningParameters(provisioningParameters: List): FluentBuilder = apply { this.provisioningParameters = provisioningParameters }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy