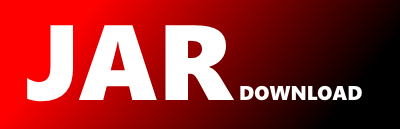
aws.sdk.kotlin.services.sagemaker.model.TransformOutput.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
/**
* Describes the results of a transform job.
*/
class TransformOutput private constructor(builder: BuilderImpl) {
/**
* The MIME type used to specify the output data. Amazon SageMaker uses the MIME type with each http
* call to transfer data from the transform job.
*/
val accept: String? = builder.accept
/**
* Defines how to assemble the results of the transform job as a single S3 object. Choose
* a format that is most convenient to you. To concatenate the results in binary format,
* specify None. To add a newline character at the end of every transformed
* record, specify
* Line.
*/
val assembleWith: AssemblyType? = builder.assembleWith
/**
* The Amazon Web Services Key Management Service (Amazon Web Services KMS) key that Amazon SageMaker uses to encrypt the model artifacts at rest using
* Amazon S3 server-side encryption. The KmsKeyId can be any of the following
* formats:
* Key ID: 1234abcd-12ab-34cd-56ef-1234567890ab
* Key ARN:
* arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab
* Alias name: alias/ExampleAlias
* Alias name ARN:
* arn:aws:kms:us-west-2:111122223333:alias/ExampleAlias
* If you don't provide a KMS key ID, Amazon SageMaker uses the default KMS key for Amazon S3 for your
* role's account. For more information, see KMS-Managed Encryption Keys in the
* Amazon Simple Storage Service
* Developer Guide.
* The KMS key policy must grant permission to the IAM role that you specify in your
* CreateModel request. For more information, see Using
* Key Policies in Amazon Web Services KMS in the Amazon Web Services Key Management Service Developer
* Guide.
*/
val kmsKeyId: String? = builder.kmsKeyId
/**
* The Amazon S3 path where you want Amazon SageMaker to store the results of the transform job. For
* example, s3://bucket-name/key-name-prefix.
* For every S3 object used as input for the transform job, batch transform stores the
* transformed data with an .out suffix in a corresponding subfolder in the
* location in the output prefix. For example, for the input data stored at
* s3://bucket-name/input-name-prefix/dataset01/data.csv, batch transform
* stores the transformed data at
* s3://bucket-name/output-name-prefix/input-name-prefix/data.csv.out.
* Batch transform doesn't upload partially processed objects. For an input S3 object that
* contains multiple records, it creates an .out file only if the transform
* job succeeds on the entire file. When the input contains multiple S3 objects, the batch
* transform job processes the listed S3 objects and uploads only the output for
* successfully processed objects. If any object fails in the transform job batch transform
* marks the job as failed to prompt investigation.
*/
val s3OutputPath: String? = builder.s3OutputPath
companion object {
@JvmStatic
fun fluentBuilder(): FluentBuilder = BuilderImpl()
internal fun builder(): DslBuilder = BuilderImpl()
operator fun invoke(block: DslBuilder.() -> kotlin.Unit): TransformOutput = BuilderImpl().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("TransformOutput(")
append("accept=$accept,")
append("assembleWith=$assembleWith,")
append("kmsKeyId=$kmsKeyId,")
append("s3OutputPath=$s3OutputPath)")
}
override fun hashCode(): kotlin.Int {
var result = accept?.hashCode() ?: 0
result = 31 * result + (assembleWith?.hashCode() ?: 0)
result = 31 * result + (kmsKeyId?.hashCode() ?: 0)
result = 31 * result + (s3OutputPath?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as TransformOutput
if (accept != other.accept) return false
if (assembleWith != other.assembleWith) return false
if (kmsKeyId != other.kmsKeyId) return false
if (s3OutputPath != other.s3OutputPath) return false
return true
}
fun copy(block: DslBuilder.() -> kotlin.Unit = {}): TransformOutput = BuilderImpl(this).apply(block).build()
interface FluentBuilder {
fun build(): TransformOutput
/**
* The MIME type used to specify the output data. Amazon SageMaker uses the MIME type with each http
* call to transfer data from the transform job.
*/
fun accept(accept: String): FluentBuilder
/**
* Defines how to assemble the results of the transform job as a single S3 object. Choose
* a format that is most convenient to you. To concatenate the results in binary format,
* specify None. To add a newline character at the end of every transformed
* record, specify
* Line.
*/
fun assembleWith(assembleWith: AssemblyType): FluentBuilder
/**
* The Amazon Web Services Key Management Service (Amazon Web Services KMS) key that Amazon SageMaker uses to encrypt the model artifacts at rest using
* Amazon S3 server-side encryption. The KmsKeyId can be any of the following
* formats:
* Key ID: 1234abcd-12ab-34cd-56ef-1234567890ab
* Key ARN:
* arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab
* Alias name: alias/ExampleAlias
* Alias name ARN:
* arn:aws:kms:us-west-2:111122223333:alias/ExampleAlias
* If you don't provide a KMS key ID, Amazon SageMaker uses the default KMS key for Amazon S3 for your
* role's account. For more information, see KMS-Managed Encryption Keys in the
* Amazon Simple Storage Service
* Developer Guide.
* The KMS key policy must grant permission to the IAM role that you specify in your
* CreateModel request. For more information, see Using
* Key Policies in Amazon Web Services KMS in the Amazon Web Services Key Management Service Developer
* Guide.
*/
fun kmsKeyId(kmsKeyId: String): FluentBuilder
/**
* The Amazon S3 path where you want Amazon SageMaker to store the results of the transform job. For
* example, s3://bucket-name/key-name-prefix.
* For every S3 object used as input for the transform job, batch transform stores the
* transformed data with an .out suffix in a corresponding subfolder in the
* location in the output prefix. For example, for the input data stored at
* s3://bucket-name/input-name-prefix/dataset01/data.csv, batch transform
* stores the transformed data at
* s3://bucket-name/output-name-prefix/input-name-prefix/data.csv.out.
* Batch transform doesn't upload partially processed objects. For an input S3 object that
* contains multiple records, it creates an .out file only if the transform
* job succeeds on the entire file. When the input contains multiple S3 objects, the batch
* transform job processes the listed S3 objects and uploads only the output for
* successfully processed objects. If any object fails in the transform job batch transform
* marks the job as failed to prompt investigation.
*/
fun s3OutputPath(s3OutputPath: String): FluentBuilder
}
interface DslBuilder {
/**
* The MIME type used to specify the output data. Amazon SageMaker uses the MIME type with each http
* call to transfer data from the transform job.
*/
var accept: String?
/**
* Defines how to assemble the results of the transform job as a single S3 object. Choose
* a format that is most convenient to you. To concatenate the results in binary format,
* specify None. To add a newline character at the end of every transformed
* record, specify
* Line.
*/
var assembleWith: AssemblyType?
/**
* The Amazon Web Services Key Management Service (Amazon Web Services KMS) key that Amazon SageMaker uses to encrypt the model artifacts at rest using
* Amazon S3 server-side encryption. The KmsKeyId can be any of the following
* formats:
* Key ID: 1234abcd-12ab-34cd-56ef-1234567890ab
* Key ARN:
* arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab
* Alias name: alias/ExampleAlias
* Alias name ARN:
* arn:aws:kms:us-west-2:111122223333:alias/ExampleAlias
* If you don't provide a KMS key ID, Amazon SageMaker uses the default KMS key for Amazon S3 for your
* role's account. For more information, see KMS-Managed Encryption Keys in the
* Amazon Simple Storage Service
* Developer Guide.
* The KMS key policy must grant permission to the IAM role that you specify in your
* CreateModel request. For more information, see Using
* Key Policies in Amazon Web Services KMS in the Amazon Web Services Key Management Service Developer
* Guide.
*/
var kmsKeyId: String?
/**
* The Amazon S3 path where you want Amazon SageMaker to store the results of the transform job. For
* example, s3://bucket-name/key-name-prefix.
* For every S3 object used as input for the transform job, batch transform stores the
* transformed data with an .out suffix in a corresponding subfolder in the
* location in the output prefix. For example, for the input data stored at
* s3://bucket-name/input-name-prefix/dataset01/data.csv, batch transform
* stores the transformed data at
* s3://bucket-name/output-name-prefix/input-name-prefix/data.csv.out.
* Batch transform doesn't upload partially processed objects. For an input S3 object that
* contains multiple records, it creates an .out file only if the transform
* job succeeds on the entire file. When the input contains multiple S3 objects, the batch
* transform job processes the listed S3 objects and uploads only the output for
* successfully processed objects. If any object fails in the transform job batch transform
* marks the job as failed to prompt investigation.
*/
var s3OutputPath: String?
fun build(): TransformOutput
}
private class BuilderImpl() : FluentBuilder, DslBuilder {
override var accept: String? = null
override var assembleWith: AssemblyType? = null
override var kmsKeyId: String? = null
override var s3OutputPath: String? = null
constructor(x: TransformOutput) : this() {
this.accept = x.accept
this.assembleWith = x.assembleWith
this.kmsKeyId = x.kmsKeyId
this.s3OutputPath = x.s3OutputPath
}
override fun build(): TransformOutput = TransformOutput(this)
override fun accept(accept: String): FluentBuilder = apply { this.accept = accept }
override fun assembleWith(assembleWith: AssemblyType): FluentBuilder = apply { this.assembleWith = assembleWith }
override fun kmsKeyId(kmsKeyId: String): FluentBuilder = apply { this.kmsKeyId = kmsKeyId }
override fun s3OutputPath(s3OutputPath: String): FluentBuilder = apply { this.s3OutputPath = s3OutputPath }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy