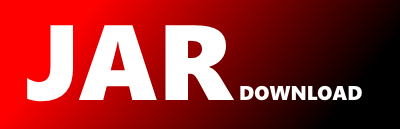
aws.sdk.kotlin.services.sagemaker.model.UpdateArtifactRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.model
class UpdateArtifactRequest private constructor(builder: BuilderImpl) {
/**
* The Amazon Resource Name (ARN) of the artifact to update.
*/
val artifactArn: String? = builder.artifactArn
/**
* The new name for the artifact.
*/
val artifactName: String? = builder.artifactName
/**
* The new list of properties. Overwrites the current property list.
*/
val properties: Map? = builder.properties
/**
* A list of properties to remove.
*/
val propertiesToRemove: List? = builder.propertiesToRemove
companion object {
@JvmStatic
fun fluentBuilder(): FluentBuilder = BuilderImpl()
internal fun builder(): DslBuilder = BuilderImpl()
operator fun invoke(block: DslBuilder.() -> kotlin.Unit): UpdateArtifactRequest = BuilderImpl().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("UpdateArtifactRequest(")
append("artifactArn=$artifactArn,")
append("artifactName=$artifactName,")
append("properties=$properties,")
append("propertiesToRemove=$propertiesToRemove)")
}
override fun hashCode(): kotlin.Int {
var result = artifactArn?.hashCode() ?: 0
result = 31 * result + (artifactName?.hashCode() ?: 0)
result = 31 * result + (properties?.hashCode() ?: 0)
result = 31 * result + (propertiesToRemove?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as UpdateArtifactRequest
if (artifactArn != other.artifactArn) return false
if (artifactName != other.artifactName) return false
if (properties != other.properties) return false
if (propertiesToRemove != other.propertiesToRemove) return false
return true
}
fun copy(block: DslBuilder.() -> kotlin.Unit = {}): UpdateArtifactRequest = BuilderImpl(this).apply(block).build()
interface FluentBuilder {
fun build(): UpdateArtifactRequest
/**
* The Amazon Resource Name (ARN) of the artifact to update.
*/
fun artifactArn(artifactArn: String): FluentBuilder
/**
* The new name for the artifact.
*/
fun artifactName(artifactName: String): FluentBuilder
/**
* The new list of properties. Overwrites the current property list.
*/
fun properties(properties: Map): FluentBuilder
/**
* A list of properties to remove.
*/
fun propertiesToRemove(propertiesToRemove: List): FluentBuilder
}
interface DslBuilder {
/**
* The Amazon Resource Name (ARN) of the artifact to update.
*/
var artifactArn: String?
/**
* The new name for the artifact.
*/
var artifactName: String?
/**
* The new list of properties. Overwrites the current property list.
*/
var properties: Map?
/**
* A list of properties to remove.
*/
var propertiesToRemove: List?
fun build(): UpdateArtifactRequest
}
private class BuilderImpl() : FluentBuilder, DslBuilder {
override var artifactArn: String? = null
override var artifactName: String? = null
override var properties: Map? = null
override var propertiesToRemove: List? = null
constructor(x: UpdateArtifactRequest) : this() {
this.artifactArn = x.artifactArn
this.artifactName = x.artifactName
this.properties = x.properties
this.propertiesToRemove = x.propertiesToRemove
}
override fun build(): UpdateArtifactRequest = UpdateArtifactRequest(this)
override fun artifactArn(artifactArn: String): FluentBuilder = apply { this.artifactArn = artifactArn }
override fun artifactName(artifactName: String): FluentBuilder = apply { this.artifactName = artifactName }
override fun properties(properties: Map): FluentBuilder = apply { this.properties = properties }
override fun propertiesToRemove(propertiesToRemove: List): FluentBuilder = apply { this.propertiesToRemove = propertiesToRemove }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy