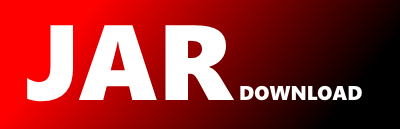
aws.sdk.kotlin.services.sagemaker.transform.TrainingJobDocumentDeserializer.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemaker.transform
import aws.sdk.kotlin.services.sagemaker.model.Channel
import aws.sdk.kotlin.services.sagemaker.model.DebugRuleConfiguration
import aws.sdk.kotlin.services.sagemaker.model.DebugRuleEvaluationStatus
import aws.sdk.kotlin.services.sagemaker.model.MetricData
import aws.sdk.kotlin.services.sagemaker.model.SecondaryStatus
import aws.sdk.kotlin.services.sagemaker.model.SecondaryStatusTransition
import aws.sdk.kotlin.services.sagemaker.model.Tag
import aws.sdk.kotlin.services.sagemaker.model.TrainingJob
import aws.sdk.kotlin.services.sagemaker.model.TrainingJobStatus
import aws.smithy.kotlin.runtime.serde.Deserializer
import aws.smithy.kotlin.runtime.serde.SdkFieldDescriptor
import aws.smithy.kotlin.runtime.serde.SdkObjectDescriptor
import aws.smithy.kotlin.runtime.serde.SerialKind
import aws.smithy.kotlin.runtime.serde.asSdkSerializable
import aws.smithy.kotlin.runtime.serde.deserializeList
import aws.smithy.kotlin.runtime.serde.deserializeMap
import aws.smithy.kotlin.runtime.serde.deserializeStruct
import aws.smithy.kotlin.runtime.serde.field
import aws.smithy.kotlin.runtime.serde.json.JsonDeserializer
import aws.smithy.kotlin.runtime.serde.json.JsonSerialName
import aws.smithy.kotlin.runtime.serde.serializeList
import aws.smithy.kotlin.runtime.serde.serializeMap
import aws.smithy.kotlin.runtime.serde.serializeStruct
import aws.smithy.kotlin.runtime.time.Instant
internal suspend fun deserializeTrainingJobDocument(deserializer: Deserializer): TrainingJob {
val builder = TrainingJob.builder()
val ALGORITHMSPECIFICATION_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Struct, JsonSerialName("AlgorithmSpecification"))
val AUTOMLJOBARN_DESCRIPTOR = SdkFieldDescriptor(SerialKind.String, JsonSerialName("AutoMLJobArn"))
val BILLABLETIMEINSECONDS_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Integer, JsonSerialName("BillableTimeInSeconds"))
val CHECKPOINTCONFIG_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Struct, JsonSerialName("CheckpointConfig"))
val CREATIONTIME_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Timestamp, JsonSerialName("CreationTime"))
val DEBUGHOOKCONFIG_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Struct, JsonSerialName("DebugHookConfig"))
val DEBUGRULECONFIGURATIONS_DESCRIPTOR = SdkFieldDescriptor(SerialKind.List, JsonSerialName("DebugRuleConfigurations"))
val DEBUGRULEEVALUATIONSTATUSES_DESCRIPTOR = SdkFieldDescriptor(SerialKind.List, JsonSerialName("DebugRuleEvaluationStatuses"))
val ENABLEINTERCONTAINERTRAFFICENCRYPTION_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Boolean, JsonSerialName("EnableInterContainerTrafficEncryption"))
val ENABLEMANAGEDSPOTTRAINING_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Boolean, JsonSerialName("EnableManagedSpotTraining"))
val ENABLENETWORKISOLATION_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Boolean, JsonSerialName("EnableNetworkIsolation"))
val ENVIRONMENT_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Map, JsonSerialName("Environment"))
val EXPERIMENTCONFIG_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Struct, JsonSerialName("ExperimentConfig"))
val FAILUREREASON_DESCRIPTOR = SdkFieldDescriptor(SerialKind.String, JsonSerialName("FailureReason"))
val FINALMETRICDATALIST_DESCRIPTOR = SdkFieldDescriptor(SerialKind.List, JsonSerialName("FinalMetricDataList"))
val HYPERPARAMETERS_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Map, JsonSerialName("HyperParameters"))
val INPUTDATACONFIG_DESCRIPTOR = SdkFieldDescriptor(SerialKind.List, JsonSerialName("InputDataConfig"))
val LABELINGJOBARN_DESCRIPTOR = SdkFieldDescriptor(SerialKind.String, JsonSerialName("LabelingJobArn"))
val LASTMODIFIEDTIME_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Timestamp, JsonSerialName("LastModifiedTime"))
val MODELARTIFACTS_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Struct, JsonSerialName("ModelArtifacts"))
val OUTPUTDATACONFIG_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Struct, JsonSerialName("OutputDataConfig"))
val RESOURCECONFIG_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Struct, JsonSerialName("ResourceConfig"))
val RETRYSTRATEGY_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Struct, JsonSerialName("RetryStrategy"))
val ROLEARN_DESCRIPTOR = SdkFieldDescriptor(SerialKind.String, JsonSerialName("RoleArn"))
val SECONDARYSTATUS_DESCRIPTOR = SdkFieldDescriptor(SerialKind.String, JsonSerialName("SecondaryStatus"))
val SECONDARYSTATUSTRANSITIONS_DESCRIPTOR = SdkFieldDescriptor(SerialKind.List, JsonSerialName("SecondaryStatusTransitions"))
val STOPPINGCONDITION_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Struct, JsonSerialName("StoppingCondition"))
val TAGS_DESCRIPTOR = SdkFieldDescriptor(SerialKind.List, JsonSerialName("Tags"))
val TENSORBOARDOUTPUTCONFIG_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Struct, JsonSerialName("TensorBoardOutputConfig"))
val TRAININGENDTIME_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Timestamp, JsonSerialName("TrainingEndTime"))
val TRAININGJOBARN_DESCRIPTOR = SdkFieldDescriptor(SerialKind.String, JsonSerialName("TrainingJobArn"))
val TRAININGJOBNAME_DESCRIPTOR = SdkFieldDescriptor(SerialKind.String, JsonSerialName("TrainingJobName"))
val TRAININGJOBSTATUS_DESCRIPTOR = SdkFieldDescriptor(SerialKind.String, JsonSerialName("TrainingJobStatus"))
val TRAININGSTARTTIME_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Timestamp, JsonSerialName("TrainingStartTime"))
val TRAININGTIMEINSECONDS_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Integer, JsonSerialName("TrainingTimeInSeconds"))
val TUNINGJOBARN_DESCRIPTOR = SdkFieldDescriptor(SerialKind.String, JsonSerialName("TuningJobArn"))
val VPCCONFIG_DESCRIPTOR = SdkFieldDescriptor(SerialKind.Struct, JsonSerialName("VpcConfig"))
val OBJ_DESCRIPTOR = SdkObjectDescriptor.build {
field(ALGORITHMSPECIFICATION_DESCRIPTOR)
field(AUTOMLJOBARN_DESCRIPTOR)
field(BILLABLETIMEINSECONDS_DESCRIPTOR)
field(CHECKPOINTCONFIG_DESCRIPTOR)
field(CREATIONTIME_DESCRIPTOR)
field(DEBUGHOOKCONFIG_DESCRIPTOR)
field(DEBUGRULECONFIGURATIONS_DESCRIPTOR)
field(DEBUGRULEEVALUATIONSTATUSES_DESCRIPTOR)
field(ENABLEINTERCONTAINERTRAFFICENCRYPTION_DESCRIPTOR)
field(ENABLEMANAGEDSPOTTRAINING_DESCRIPTOR)
field(ENABLENETWORKISOLATION_DESCRIPTOR)
field(ENVIRONMENT_DESCRIPTOR)
field(EXPERIMENTCONFIG_DESCRIPTOR)
field(FAILUREREASON_DESCRIPTOR)
field(FINALMETRICDATALIST_DESCRIPTOR)
field(HYPERPARAMETERS_DESCRIPTOR)
field(INPUTDATACONFIG_DESCRIPTOR)
field(LABELINGJOBARN_DESCRIPTOR)
field(LASTMODIFIEDTIME_DESCRIPTOR)
field(MODELARTIFACTS_DESCRIPTOR)
field(OUTPUTDATACONFIG_DESCRIPTOR)
field(RESOURCECONFIG_DESCRIPTOR)
field(RETRYSTRATEGY_DESCRIPTOR)
field(ROLEARN_DESCRIPTOR)
field(SECONDARYSTATUS_DESCRIPTOR)
field(SECONDARYSTATUSTRANSITIONS_DESCRIPTOR)
field(STOPPINGCONDITION_DESCRIPTOR)
field(TAGS_DESCRIPTOR)
field(TENSORBOARDOUTPUTCONFIG_DESCRIPTOR)
field(TRAININGENDTIME_DESCRIPTOR)
field(TRAININGJOBARN_DESCRIPTOR)
field(TRAININGJOBNAME_DESCRIPTOR)
field(TRAININGJOBSTATUS_DESCRIPTOR)
field(TRAININGSTARTTIME_DESCRIPTOR)
field(TRAININGTIMEINSECONDS_DESCRIPTOR)
field(TUNINGJOBARN_DESCRIPTOR)
field(VPCCONFIG_DESCRIPTOR)
}
deserializer.deserializeStruct(OBJ_DESCRIPTOR) {
loop@while (true) {
when (findNextFieldIndex()) {
ALGORITHMSPECIFICATION_DESCRIPTOR.index -> builder.algorithmSpecification = deserializeAlgorithmSpecificationDocument(deserializer)
AUTOMLJOBARN_DESCRIPTOR.index -> builder.autoMlJobArn = deserializeString()
BILLABLETIMEINSECONDS_DESCRIPTOR.index -> builder.billableTimeInSeconds = deserializeInt()
CHECKPOINTCONFIG_DESCRIPTOR.index -> builder.checkpointConfig = deserializeCheckpointConfigDocument(deserializer)
CREATIONTIME_DESCRIPTOR.index -> builder.creationTime = deserializeString().let { Instant.fromEpochSeconds(it) }
DEBUGHOOKCONFIG_DESCRIPTOR.index -> builder.debugHookConfig = deserializeDebugHookConfigDocument(deserializer)
DEBUGRULECONFIGURATIONS_DESCRIPTOR.index -> builder.debugRuleConfigurations =
deserializer.deserializeList(DEBUGRULECONFIGURATIONS_DESCRIPTOR) {
val col0 = mutableListOf()
while (hasNextElement()) {
val el0 = if (nextHasValue()) { deserializeDebugRuleConfigurationDocument(deserializer) } else { deserializeNull(); continue }
col0.add(el0)
}
col0
}
DEBUGRULEEVALUATIONSTATUSES_DESCRIPTOR.index -> builder.debugRuleEvaluationStatuses =
deserializer.deserializeList(DEBUGRULEEVALUATIONSTATUSES_DESCRIPTOR) {
val col0 = mutableListOf()
while (hasNextElement()) {
val el0 = if (nextHasValue()) { deserializeDebugRuleEvaluationStatusDocument(deserializer) } else { deserializeNull(); continue }
col0.add(el0)
}
col0
}
ENABLEINTERCONTAINERTRAFFICENCRYPTION_DESCRIPTOR.index -> builder.enableInterContainerTrafficEncryption = deserializeBoolean()
ENABLEMANAGEDSPOTTRAINING_DESCRIPTOR.index -> builder.enableManagedSpotTraining = deserializeBoolean()
ENABLENETWORKISOLATION_DESCRIPTOR.index -> builder.enableNetworkIsolation = deserializeBoolean()
ENVIRONMENT_DESCRIPTOR.index -> builder.environment =
deserializer.deserializeMap(ENVIRONMENT_DESCRIPTOR) {
val map0 = mutableMapOf()
while (hasNextEntry()) {
val k0 = key()
val v0 = if (nextHasValue()) { deserializeString() } else { deserializeNull(); continue }
map0[k0] = v0
}
map0
}
EXPERIMENTCONFIG_DESCRIPTOR.index -> builder.experimentConfig = deserializeExperimentConfigDocument(deserializer)
FAILUREREASON_DESCRIPTOR.index -> builder.failureReason = deserializeString()
FINALMETRICDATALIST_DESCRIPTOR.index -> builder.finalMetricDataList =
deserializer.deserializeList(FINALMETRICDATALIST_DESCRIPTOR) {
val col0 = mutableListOf()
while (hasNextElement()) {
val el0 = if (nextHasValue()) { deserializeMetricDataDocument(deserializer) } else { deserializeNull(); continue }
col0.add(el0)
}
col0
}
HYPERPARAMETERS_DESCRIPTOR.index -> builder.hyperParameters =
deserializer.deserializeMap(HYPERPARAMETERS_DESCRIPTOR) {
val map0 = mutableMapOf()
while (hasNextEntry()) {
val k0 = key()
val v0 = if (nextHasValue()) { deserializeString() } else { deserializeNull(); continue }
map0[k0] = v0
}
map0
}
INPUTDATACONFIG_DESCRIPTOR.index -> builder.inputDataConfig =
deserializer.deserializeList(INPUTDATACONFIG_DESCRIPTOR) {
val col0 = mutableListOf()
while (hasNextElement()) {
val el0 = if (nextHasValue()) { deserializeChannelDocument(deserializer) } else { deserializeNull(); continue }
col0.add(el0)
}
col0
}
LABELINGJOBARN_DESCRIPTOR.index -> builder.labelingJobArn = deserializeString()
LASTMODIFIEDTIME_DESCRIPTOR.index -> builder.lastModifiedTime = deserializeString().let { Instant.fromEpochSeconds(it) }
MODELARTIFACTS_DESCRIPTOR.index -> builder.modelArtifacts = deserializeModelArtifactsDocument(deserializer)
OUTPUTDATACONFIG_DESCRIPTOR.index -> builder.outputDataConfig = deserializeOutputDataConfigDocument(deserializer)
RESOURCECONFIG_DESCRIPTOR.index -> builder.resourceConfig = deserializeResourceConfigDocument(deserializer)
RETRYSTRATEGY_DESCRIPTOR.index -> builder.retryStrategy = deserializeRetryStrategyDocument(deserializer)
ROLEARN_DESCRIPTOR.index -> builder.roleArn = deserializeString()
SECONDARYSTATUS_DESCRIPTOR.index -> builder.secondaryStatus = deserializeString().let { SecondaryStatus.fromValue(it) }
SECONDARYSTATUSTRANSITIONS_DESCRIPTOR.index -> builder.secondaryStatusTransitions =
deserializer.deserializeList(SECONDARYSTATUSTRANSITIONS_DESCRIPTOR) {
val col0 = mutableListOf()
while (hasNextElement()) {
val el0 = if (nextHasValue()) { deserializeSecondaryStatusTransitionDocument(deserializer) } else { deserializeNull(); continue }
col0.add(el0)
}
col0
}
STOPPINGCONDITION_DESCRIPTOR.index -> builder.stoppingCondition = deserializeStoppingConditionDocument(deserializer)
TAGS_DESCRIPTOR.index -> builder.tags =
deserializer.deserializeList(TAGS_DESCRIPTOR) {
val col0 = mutableListOf()
while (hasNextElement()) {
val el0 = if (nextHasValue()) { deserializeTagDocument(deserializer) } else { deserializeNull(); continue }
col0.add(el0)
}
col0
}
TENSORBOARDOUTPUTCONFIG_DESCRIPTOR.index -> builder.tensorBoardOutputConfig = deserializeTensorBoardOutputConfigDocument(deserializer)
TRAININGENDTIME_DESCRIPTOR.index -> builder.trainingEndTime = deserializeString().let { Instant.fromEpochSeconds(it) }
TRAININGJOBARN_DESCRIPTOR.index -> builder.trainingJobArn = deserializeString()
TRAININGJOBNAME_DESCRIPTOR.index -> builder.trainingJobName = deserializeString()
TRAININGJOBSTATUS_DESCRIPTOR.index -> builder.trainingJobStatus = deserializeString().let { TrainingJobStatus.fromValue(it) }
TRAININGSTARTTIME_DESCRIPTOR.index -> builder.trainingStartTime = deserializeString().let { Instant.fromEpochSeconds(it) }
TRAININGTIMEINSECONDS_DESCRIPTOR.index -> builder.trainingTimeInSeconds = deserializeInt()
TUNINGJOBARN_DESCRIPTOR.index -> builder.tuningJobArn = deserializeString()
VPCCONFIG_DESCRIPTOR.index -> builder.vpcConfig = deserializeVpcConfigDocument(deserializer)
null -> break@loop
else -> skipValue()
}
}
}
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy