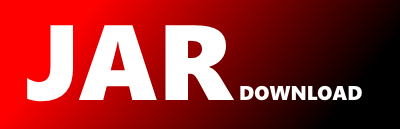
commonMain.aws.sdk.kotlin.services.sagemakeredge.DefaultSagemakerEdgeClient.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sagemakeredge Show documentation
Show all versions of sagemakeredge Show documentation
The AWS SDK for Kotlin client for Sagemaker Edge
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.sagemakeredge
import aws.sdk.kotlin.runtime.client.AwsClientOption
import aws.sdk.kotlin.runtime.http.ApiMetadata
import aws.sdk.kotlin.runtime.http.AwsUserAgentMetadata
import aws.sdk.kotlin.runtime.http.middleware.AwsRetryHeaderMiddleware
import aws.sdk.kotlin.runtime.http.middleware.RecursionDetection
import aws.sdk.kotlin.runtime.http.middleware.UserAgent
import aws.sdk.kotlin.services.sagemakeredge.auth.AuthSchemeProviderAdapter
import aws.sdk.kotlin.services.sagemakeredge.auth.IdentityProviderConfigAdapter
import aws.sdk.kotlin.services.sagemakeredge.endpoints.internal.EndpointResolverAdapter
import aws.sdk.kotlin.services.sagemakeredge.model.*
import aws.sdk.kotlin.services.sagemakeredge.transform.*
import aws.smithy.kotlin.runtime.auth.AuthSchemeId
import aws.smithy.kotlin.runtime.auth.awssigning.AwsSigningAttributes
import aws.smithy.kotlin.runtime.auth.awssigning.DefaultAwsSigner
import aws.smithy.kotlin.runtime.client.SdkClientOption
import aws.smithy.kotlin.runtime.http.SdkHttpClient
import aws.smithy.kotlin.runtime.http.auth.HttpAuthScheme
import aws.smithy.kotlin.runtime.http.auth.SigV4AuthScheme
import aws.smithy.kotlin.runtime.http.operation.OperationAuthConfig
import aws.smithy.kotlin.runtime.http.operation.SdkHttpOperation
import aws.smithy.kotlin.runtime.http.operation.context
import aws.smithy.kotlin.runtime.http.operation.roundTrip
import aws.smithy.kotlin.runtime.http.operation.sdkRequestId
import aws.smithy.kotlin.runtime.io.SdkManagedGroup
import aws.smithy.kotlin.runtime.io.addIfManaged
import aws.smithy.kotlin.runtime.operation.ExecutionContext
import aws.smithy.kotlin.runtime.tracing.withRootTraceSpan
import aws.smithy.kotlin.runtime.util.putIfAbsent
import kotlin.coroutines.coroutineContext
public const val ServiceId: String = "Sagemaker Edge"
public const val ServiceApiVersion: String = "2020-09-23"
public const val SdkVersion: String = "0.28.0-beta"
internal class DefaultSagemakerEdgeClient(override val config: SagemakerEdgeClient.Config) : SagemakerEdgeClient {
private val managedResources = SdkManagedGroup()
private val client = SdkHttpClient(config.httpClient)
private val identityProviderConfig = IdentityProviderConfigAdapter(config)
private val configuredAuthSchemes = with(config.authSchemes.associateBy(HttpAuthScheme::schemeId).toMutableMap()){
getOrPut(AuthSchemeId.AwsSigV4){
SigV4AuthScheme(DefaultAwsSigner, "sagemaker")
}
toMap()
}
init {
managedResources.addIfManaged(config.httpClient)
managedResources.addIfManaged(config.credentialsProvider)
}
private val awsUserAgentMetadata = AwsUserAgentMetadata.fromEnvironment(ApiMetadata(ServiceId, SdkVersion))
/**
* Use to get the active deployments from a device.
*/
override suspend fun getDeployments(input: GetDeploymentsRequest): GetDeploymentsResponse {
val op = SdkHttpOperation.build {
serializer = GetDeploymentsOperationSerializer()
deserializer = GetDeploymentsOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "GetDeployments"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("GetDeployments-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Use to check if a device is registered with SageMaker Edge Manager.
*/
override suspend fun getDeviceRegistration(input: GetDeviceRegistrationRequest): GetDeviceRegistrationResponse {
val op = SdkHttpOperation.build {
serializer = GetDeviceRegistrationOperationSerializer()
deserializer = GetDeviceRegistrationOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "GetDeviceRegistration"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("GetDeviceRegistration-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
/**
* Use to get the current status of devices registered on SageMaker Edge Manager.
*/
override suspend fun sendHeartbeat(input: SendHeartbeatRequest): SendHeartbeatResponse {
val op = SdkHttpOperation.build {
serializer = SendHeartbeatOperationSerializer()
deserializer = SendHeartbeatOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "SendHeartbeat"
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
val rootSpan = config.tracer.createRootSpan("SendHeartbeat-${op.context.sdkRequestId}")
return coroutineContext.withRootTraceSpan(rootSpan) {
op.roundTrip(client, input)
}
}
override fun close() {
managedResources.unshareAll()
}
/**
* merge the defaults configured for the service into the execution context before firing off a request
*/
private suspend fun mergeServiceDefaults(ctx: ExecutionContext) {
ctx.putIfAbsent(AwsClientOption.Region, config.region)
ctx.putIfAbsent(SdkClientOption.ClientName, config.clientName)
ctx.putIfAbsent(SdkClientOption.LogMode, config.logMode)
ctx.putIfAbsent(AwsSigningAttributes.SigningService, "sagemaker")
ctx.putIfAbsent(AwsSigningAttributes.SigningRegion, config.region)
ctx.putIfAbsent(AwsSigningAttributes.CredentialsProvider, config.credentialsProvider)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy