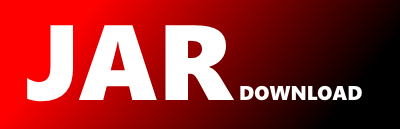
commonMain.aws.sdk.kotlin.services.servicequotas.model.RequestedServiceQuotaChange.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.servicequotas.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* Information about a quota increase request.
*/
public class RequestedServiceQuotaChange private constructor(builder: Builder) {
/**
* The case ID.
*/
public val caseId: kotlin.String? = builder.caseId
/**
* The date and time when the quota increase request was received and the case ID was created.
*/
public val created: aws.smithy.kotlin.runtime.time.Instant? = builder.created
/**
* The new, increased value for the quota.
*/
public val desiredValue: kotlin.Double? = builder.desiredValue
/**
* Indicates whether the quota is global.
*/
public val globalQuota: kotlin.Boolean = builder.globalQuota
/**
* The unique identifier.
*/
public val id: kotlin.String? = builder.id
/**
* The date and time of the most recent change.
*/
public val lastUpdated: aws.smithy.kotlin.runtime.time.Instant? = builder.lastUpdated
/**
* The Amazon Resource Name (ARN) of the quota.
*/
public val quotaArn: kotlin.String? = builder.quotaArn
/**
* Specifies the quota identifier. To find the quota code for a specific quota, use the ListServiceQuotas operation, and look for the `QuotaCode` response in the output for the quota you want.
*/
public val quotaCode: kotlin.String? = builder.quotaCode
/**
* The context for this service quota.
*/
public val quotaContext: aws.sdk.kotlin.services.servicequotas.model.QuotaContextInfo? = builder.quotaContext
/**
* Specifies the quota name.
*/
public val quotaName: kotlin.String? = builder.quotaName
/**
* Specifies at which level within the Amazon Web Services account the quota request applies to.
*/
public val quotaRequestedAtLevel: aws.sdk.kotlin.services.servicequotas.model.AppliedLevelEnum? = builder.quotaRequestedAtLevel
/**
* The IAM identity of the requester.
*/
public val requester: kotlin.String? = builder.requester
/**
* Specifies the service identifier. To find the service code value for an Amazon Web Services service, use the ListServices operation.
*/
public val serviceCode: kotlin.String? = builder.serviceCode
/**
* Specifies the service name.
*/
public val serviceName: kotlin.String? = builder.serviceName
/**
* The state of the quota increase request.
*/
public val status: aws.sdk.kotlin.services.servicequotas.model.RequestStatus? = builder.status
/**
* The unit of measurement.
*/
public val unit: kotlin.String? = builder.unit
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.servicequotas.model.RequestedServiceQuotaChange = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("RequestedServiceQuotaChange(")
append("caseId=$caseId,")
append("created=$created,")
append("desiredValue=$desiredValue,")
append("globalQuota=$globalQuota,")
append("id=$id,")
append("lastUpdated=$lastUpdated,")
append("quotaArn=$quotaArn,")
append("quotaCode=$quotaCode,")
append("quotaContext=$quotaContext,")
append("quotaName=$quotaName,")
append("quotaRequestedAtLevel=$quotaRequestedAtLevel,")
append("requester=$requester,")
append("serviceCode=$serviceCode,")
append("serviceName=$serviceName,")
append("status=$status,")
append("unit=$unit")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = caseId?.hashCode() ?: 0
result = 31 * result + (created?.hashCode() ?: 0)
result = 31 * result + (desiredValue?.hashCode() ?: 0)
result = 31 * result + (globalQuota.hashCode())
result = 31 * result + (id?.hashCode() ?: 0)
result = 31 * result + (lastUpdated?.hashCode() ?: 0)
result = 31 * result + (quotaArn?.hashCode() ?: 0)
result = 31 * result + (quotaCode?.hashCode() ?: 0)
result = 31 * result + (quotaContext?.hashCode() ?: 0)
result = 31 * result + (quotaName?.hashCode() ?: 0)
result = 31 * result + (quotaRequestedAtLevel?.hashCode() ?: 0)
result = 31 * result + (requester?.hashCode() ?: 0)
result = 31 * result + (serviceCode?.hashCode() ?: 0)
result = 31 * result + (serviceName?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
result = 31 * result + (unit?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as RequestedServiceQuotaChange
if (caseId != other.caseId) return false
if (created != other.created) return false
if (!(desiredValue?.equals(other.desiredValue) ?: (other.desiredValue == null))) return false
if (globalQuota != other.globalQuota) return false
if (id != other.id) return false
if (lastUpdated != other.lastUpdated) return false
if (quotaArn != other.quotaArn) return false
if (quotaCode != other.quotaCode) return false
if (quotaContext != other.quotaContext) return false
if (quotaName != other.quotaName) return false
if (quotaRequestedAtLevel != other.quotaRequestedAtLevel) return false
if (requester != other.requester) return false
if (serviceCode != other.serviceCode) return false
if (serviceName != other.serviceName) return false
if (status != other.status) return false
if (unit != other.unit) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.servicequotas.model.RequestedServiceQuotaChange = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The case ID.
*/
public var caseId: kotlin.String? = null
/**
* The date and time when the quota increase request was received and the case ID was created.
*/
public var created: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The new, increased value for the quota.
*/
public var desiredValue: kotlin.Double? = null
/**
* Indicates whether the quota is global.
*/
public var globalQuota: kotlin.Boolean = false
/**
* The unique identifier.
*/
public var id: kotlin.String? = null
/**
* The date and time of the most recent change.
*/
public var lastUpdated: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The Amazon Resource Name (ARN) of the quota.
*/
public var quotaArn: kotlin.String? = null
/**
* Specifies the quota identifier. To find the quota code for a specific quota, use the ListServiceQuotas operation, and look for the `QuotaCode` response in the output for the quota you want.
*/
public var quotaCode: kotlin.String? = null
/**
* The context for this service quota.
*/
public var quotaContext: aws.sdk.kotlin.services.servicequotas.model.QuotaContextInfo? = null
/**
* Specifies the quota name.
*/
public var quotaName: kotlin.String? = null
/**
* Specifies at which level within the Amazon Web Services account the quota request applies to.
*/
public var quotaRequestedAtLevel: aws.sdk.kotlin.services.servicequotas.model.AppliedLevelEnum? = null
/**
* The IAM identity of the requester.
*/
public var requester: kotlin.String? = null
/**
* Specifies the service identifier. To find the service code value for an Amazon Web Services service, use the ListServices operation.
*/
public var serviceCode: kotlin.String? = null
/**
* Specifies the service name.
*/
public var serviceName: kotlin.String? = null
/**
* The state of the quota increase request.
*/
public var status: aws.sdk.kotlin.services.servicequotas.model.RequestStatus? = null
/**
* The unit of measurement.
*/
public var unit: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.servicequotas.model.RequestedServiceQuotaChange) : this() {
this.caseId = x.caseId
this.created = x.created
this.desiredValue = x.desiredValue
this.globalQuota = x.globalQuota
this.id = x.id
this.lastUpdated = x.lastUpdated
this.quotaArn = x.quotaArn
this.quotaCode = x.quotaCode
this.quotaContext = x.quotaContext
this.quotaName = x.quotaName
this.quotaRequestedAtLevel = x.quotaRequestedAtLevel
this.requester = x.requester
this.serviceCode = x.serviceCode
this.serviceName = x.serviceName
this.status = x.status
this.unit = x.unit
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.servicequotas.model.RequestedServiceQuotaChange = RequestedServiceQuotaChange(this)
/**
* construct an [aws.sdk.kotlin.services.servicequotas.model.QuotaContextInfo] inside the given [block]
*/
public fun quotaContext(block: aws.sdk.kotlin.services.servicequotas.model.QuotaContextInfo.Builder.() -> kotlin.Unit) {
this.quotaContext = aws.sdk.kotlin.services.servicequotas.model.QuotaContextInfo.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy