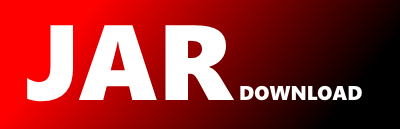
commonMain.aws.sdk.kotlin.services.shield.model.UpdateProtectionGroupRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.shield.model
public class UpdateProtectionGroupRequest private constructor(builder: Builder) {
/**
* Defines how Shield combines resource data for the group in order to detect, mitigate, and report events.
* + Sum - Use the total traffic across the group. This is a good choice for most cases. Examples include Elastic IP addresses for EC2 instances that scale manually or automatically.
* + Mean - Use the average of the traffic across the group. This is a good choice for resources that share traffic uniformly. Examples include accelerators and load balancers.
* + Max - Use the highest traffic from each resource. This is useful for resources that don't share traffic and for resources that share that traffic in a non-uniform way. Examples include Amazon CloudFront distributions and origin resources for CloudFront distributions.
*/
public val aggregation: aws.sdk.kotlin.services.shield.model.ProtectionGroupAggregation? = builder.aggregation
/**
* The Amazon Resource Names (ARNs) of the resources to include in the protection group. You must set this when you set `Pattern` to `ARBITRARY` and you must not set it for any other `Pattern` setting.
*/
public val members: List? = builder.members
/**
* The criteria to use to choose the protected resources for inclusion in the group. You can include all resources that have protections, provide a list of resource Amazon Resource Names (ARNs), or include all resources of a specified resource type.
*/
public val pattern: aws.sdk.kotlin.services.shield.model.ProtectionGroupPattern? = builder.pattern
/**
* The name of the protection group. You use this to identify the protection group in lists and to manage the protection group, for example to update, delete, or describe it.
*/
public val protectionGroupId: kotlin.String? = builder.protectionGroupId
/**
* The resource type to include in the protection group. All protected resources of this type are included in the protection group. You must set this when you set `Pattern` to `BY_RESOURCE_TYPE` and you must not set it for any other `Pattern` setting.
*/
public val resourceType: aws.sdk.kotlin.services.shield.model.ProtectedResourceType? = builder.resourceType
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.shield.model.UpdateProtectionGroupRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("UpdateProtectionGroupRequest(")
append("aggregation=$aggregation,")
append("members=$members,")
append("pattern=$pattern,")
append("protectionGroupId=$protectionGroupId,")
append("resourceType=$resourceType)")
}
override fun hashCode(): kotlin.Int {
var result = aggregation?.hashCode() ?: 0
result = 31 * result + (members?.hashCode() ?: 0)
result = 31 * result + (pattern?.hashCode() ?: 0)
result = 31 * result + (protectionGroupId?.hashCode() ?: 0)
result = 31 * result + (resourceType?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as UpdateProtectionGroupRequest
if (aggregation != other.aggregation) return false
if (members != other.members) return false
if (pattern != other.pattern) return false
if (protectionGroupId != other.protectionGroupId) return false
if (resourceType != other.resourceType) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.shield.model.UpdateProtectionGroupRequest = Builder(this).apply(block).build()
public class Builder {
/**
* Defines how Shield combines resource data for the group in order to detect, mitigate, and report events.
* + Sum - Use the total traffic across the group. This is a good choice for most cases. Examples include Elastic IP addresses for EC2 instances that scale manually or automatically.
* + Mean - Use the average of the traffic across the group. This is a good choice for resources that share traffic uniformly. Examples include accelerators and load balancers.
* + Max - Use the highest traffic from each resource. This is useful for resources that don't share traffic and for resources that share that traffic in a non-uniform way. Examples include Amazon CloudFront distributions and origin resources for CloudFront distributions.
*/
public var aggregation: aws.sdk.kotlin.services.shield.model.ProtectionGroupAggregation? = null
/**
* The Amazon Resource Names (ARNs) of the resources to include in the protection group. You must set this when you set `Pattern` to `ARBITRARY` and you must not set it for any other `Pattern` setting.
*/
public var members: List? = null
/**
* The criteria to use to choose the protected resources for inclusion in the group. You can include all resources that have protections, provide a list of resource Amazon Resource Names (ARNs), or include all resources of a specified resource type.
*/
public var pattern: aws.sdk.kotlin.services.shield.model.ProtectionGroupPattern? = null
/**
* The name of the protection group. You use this to identify the protection group in lists and to manage the protection group, for example to update, delete, or describe it.
*/
public var protectionGroupId: kotlin.String? = null
/**
* The resource type to include in the protection group. All protected resources of this type are included in the protection group. You must set this when you set `Pattern` to `BY_RESOURCE_TYPE` and you must not set it for any other `Pattern` setting.
*/
public var resourceType: aws.sdk.kotlin.services.shield.model.ProtectedResourceType? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.shield.model.UpdateProtectionGroupRequest) : this() {
this.aggregation = x.aggregation
this.members = x.members
this.pattern = x.pattern
this.protectionGroupId = x.protectionGroupId
this.resourceType = x.resourceType
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.shield.model.UpdateProtectionGroupRequest = UpdateProtectionGroupRequest(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy