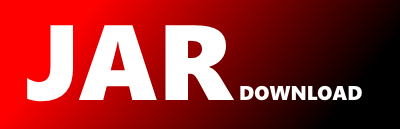
commonMain.aws.sdk.kotlin.services.shield.model.Protection.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.shield.model
/**
* An object that represents a resource that is under DDoS protection.
*/
public class Protection private constructor(builder: Builder) {
/**
* The automatic application layer DDoS mitigation settings for the protection. This configuration determines whether Shield Advanced automatically manages rules in the web ACL in order to respond to application layer events that Shield Advanced determines to be DDoS attacks.
*/
public val applicationLayerAutomaticResponseConfiguration: aws.sdk.kotlin.services.shield.model.ApplicationLayerAutomaticResponseConfiguration? = builder.applicationLayerAutomaticResponseConfiguration
/**
* The unique identifier (ID) for the Route 53 health check that's associated with the protection.
*/
public val healthCheckIds: List? = builder.healthCheckIds
/**
* The unique identifier (ID) of the protection.
*/
public val id: kotlin.String? = builder.id
/**
* The name of the protection. For example, `My CloudFront distributions`.
*/
public val name: kotlin.String? = builder.name
/**
* The ARN (Amazon Resource Name) of the protection.
*/
public val protectionArn: kotlin.String? = builder.protectionArn
/**
* The ARN (Amazon Resource Name) of the Amazon Web Services resource that is protected.
*/
public val resourceArn: kotlin.String? = builder.resourceArn
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.shield.model.Protection = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Protection(")
append("applicationLayerAutomaticResponseConfiguration=$applicationLayerAutomaticResponseConfiguration,")
append("healthCheckIds=$healthCheckIds,")
append("id=$id,")
append("name=$name,")
append("protectionArn=$protectionArn,")
append("resourceArn=$resourceArn")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = applicationLayerAutomaticResponseConfiguration?.hashCode() ?: 0
result = 31 * result + (healthCheckIds?.hashCode() ?: 0)
result = 31 * result + (id?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (protectionArn?.hashCode() ?: 0)
result = 31 * result + (resourceArn?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Protection
if (applicationLayerAutomaticResponseConfiguration != other.applicationLayerAutomaticResponseConfiguration) return false
if (healthCheckIds != other.healthCheckIds) return false
if (id != other.id) return false
if (name != other.name) return false
if (protectionArn != other.protectionArn) return false
if (resourceArn != other.resourceArn) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.shield.model.Protection = Builder(this).apply(block).build()
public class Builder {
/**
* The automatic application layer DDoS mitigation settings for the protection. This configuration determines whether Shield Advanced automatically manages rules in the web ACL in order to respond to application layer events that Shield Advanced determines to be DDoS attacks.
*/
public var applicationLayerAutomaticResponseConfiguration: aws.sdk.kotlin.services.shield.model.ApplicationLayerAutomaticResponseConfiguration? = null
/**
* The unique identifier (ID) for the Route 53 health check that's associated with the protection.
*/
public var healthCheckIds: List? = null
/**
* The unique identifier (ID) of the protection.
*/
public var id: kotlin.String? = null
/**
* The name of the protection. For example, `My CloudFront distributions`.
*/
public var name: kotlin.String? = null
/**
* The ARN (Amazon Resource Name) of the protection.
*/
public var protectionArn: kotlin.String? = null
/**
* The ARN (Amazon Resource Name) of the Amazon Web Services resource that is protected.
*/
public var resourceArn: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.shield.model.Protection) : this() {
this.applicationLayerAutomaticResponseConfiguration = x.applicationLayerAutomaticResponseConfiguration
this.healthCheckIds = x.healthCheckIds
this.id = x.id
this.name = x.name
this.protectionArn = x.protectionArn
this.resourceArn = x.resourceArn
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.shield.model.Protection = Protection(this)
/**
* construct an [aws.sdk.kotlin.services.shield.model.ApplicationLayerAutomaticResponseConfiguration] inside the given [block]
*/
public fun applicationLayerAutomaticResponseConfiguration(block: aws.sdk.kotlin.services.shield.model.ApplicationLayerAutomaticResponseConfiguration.Builder.() -> kotlin.Unit) {
this.applicationLayerAutomaticResponseConfiguration = aws.sdk.kotlin.services.shield.model.ApplicationLayerAutomaticResponseConfiguration.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy