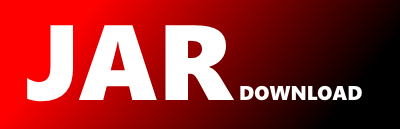
commonMain.aws.sdk.kotlin.services.shield.model.AttackVolume.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.shield.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Information about the volume of attacks during the time period, included in an AttackStatisticsDataItem. If the accompanying `AttackCount` in the statistics object is zero, this setting might be empty.
*/
public class AttackVolume private constructor(builder: Builder) {
/**
* A statistics object that uses bits per second as the unit. This is included for network level attacks.
*/
public val bitsPerSecond: aws.sdk.kotlin.services.shield.model.AttackVolumeStatistics? = builder.bitsPerSecond
/**
* A statistics object that uses packets per second as the unit. This is included for network level attacks.
*/
public val packetsPerSecond: aws.sdk.kotlin.services.shield.model.AttackVolumeStatistics? = builder.packetsPerSecond
/**
* A statistics object that uses requests per second as the unit. This is included for application level attacks, and is only available for accounts that are subscribed to Shield Advanced.
*/
public val requestsPerSecond: aws.sdk.kotlin.services.shield.model.AttackVolumeStatistics? = builder.requestsPerSecond
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.shield.model.AttackVolume = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("AttackVolume(")
append("bitsPerSecond=$bitsPerSecond,")
append("packetsPerSecond=$packetsPerSecond,")
append("requestsPerSecond=$requestsPerSecond")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = bitsPerSecond?.hashCode() ?: 0
result = 31 * result + (packetsPerSecond?.hashCode() ?: 0)
result = 31 * result + (requestsPerSecond?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as AttackVolume
if (bitsPerSecond != other.bitsPerSecond) return false
if (packetsPerSecond != other.packetsPerSecond) return false
if (requestsPerSecond != other.requestsPerSecond) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.shield.model.AttackVolume = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* A statistics object that uses bits per second as the unit. This is included for network level attacks.
*/
public var bitsPerSecond: aws.sdk.kotlin.services.shield.model.AttackVolumeStatistics? = null
/**
* A statistics object that uses packets per second as the unit. This is included for network level attacks.
*/
public var packetsPerSecond: aws.sdk.kotlin.services.shield.model.AttackVolumeStatistics? = null
/**
* A statistics object that uses requests per second as the unit. This is included for application level attacks, and is only available for accounts that are subscribed to Shield Advanced.
*/
public var requestsPerSecond: aws.sdk.kotlin.services.shield.model.AttackVolumeStatistics? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.shield.model.AttackVolume) : this() {
this.bitsPerSecond = x.bitsPerSecond
this.packetsPerSecond = x.packetsPerSecond
this.requestsPerSecond = x.requestsPerSecond
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.shield.model.AttackVolume = AttackVolume(this)
/**
* construct an [aws.sdk.kotlin.services.shield.model.AttackVolumeStatistics] inside the given [block]
*/
public fun bitsPerSecond(block: aws.sdk.kotlin.services.shield.model.AttackVolumeStatistics.Builder.() -> kotlin.Unit) {
this.bitsPerSecond = aws.sdk.kotlin.services.shield.model.AttackVolumeStatistics.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.shield.model.AttackVolumeStatistics] inside the given [block]
*/
public fun packetsPerSecond(block: aws.sdk.kotlin.services.shield.model.AttackVolumeStatistics.Builder.() -> kotlin.Unit) {
this.packetsPerSecond = aws.sdk.kotlin.services.shield.model.AttackVolumeStatistics.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.shield.model.AttackVolumeStatistics] inside the given [block]
*/
public fun requestsPerSecond(block: aws.sdk.kotlin.services.shield.model.AttackVolumeStatistics.Builder.() -> kotlin.Unit) {
this.requestsPerSecond = aws.sdk.kotlin.services.shield.model.AttackVolumeStatistics.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy