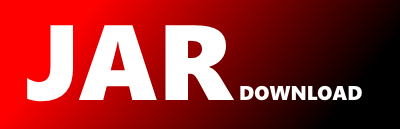
commonMain.aws.sdk.kotlin.services.shield.model.SummarizedCounter.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of shield Show documentation
Show all versions of shield Show documentation
The AWS SDK for Kotlin client for Shield
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.shield.model
/**
* The counter that describes a DDoS attack.
*/
public class SummarizedCounter private constructor(builder: Builder) {
/**
* The average value of the counter for a specified time period.
*/
public val average: kotlin.Double = builder.average
/**
* The maximum value of the counter for a specified time period.
*/
public val max: kotlin.Double = builder.max
/**
* The number of counters for a specified time period.
*/
public val n: kotlin.Int = builder.n
/**
* The counter name.
*/
public val name: kotlin.String? = builder.name
/**
* The total of counter values for a specified time period.
*/
public val sum: kotlin.Double = builder.sum
/**
* The unit of the counters.
*/
public val unit: kotlin.String? = builder.unit
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.shield.model.SummarizedCounter = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("SummarizedCounter(")
append("average=$average,")
append("max=$max,")
append("n=$n,")
append("name=$name,")
append("sum=$sum,")
append("unit=$unit)")
}
override fun hashCode(): kotlin.Int {
var result = average.hashCode()
result = 31 * result + (max.hashCode())
result = 31 * result + (n)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (sum.hashCode())
result = 31 * result + (unit?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as SummarizedCounter
if (average != other.average) return false
if (max != other.max) return false
if (n != other.n) return false
if (name != other.name) return false
if (sum != other.sum) return false
if (unit != other.unit) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.shield.model.SummarizedCounter = Builder(this).apply(block).build()
public class Builder {
/**
* The average value of the counter for a specified time period.
*/
public var average: kotlin.Double = 0.0
/**
* The maximum value of the counter for a specified time period.
*/
public var max: kotlin.Double = 0.0
/**
* The number of counters for a specified time period.
*/
public var n: kotlin.Int = 0
/**
* The counter name.
*/
public var name: kotlin.String? = null
/**
* The total of counter values for a specified time period.
*/
public var sum: kotlin.Double = 0.0
/**
* The unit of the counters.
*/
public var unit: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.shield.model.SummarizedCounter) : this() {
this.average = x.average
this.max = x.max
this.n = x.n
this.name = x.name
this.sum = x.sum
this.unit = x.unit
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.shield.model.SummarizedCounter = SummarizedCounter(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy