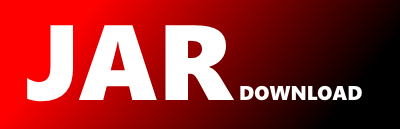
commonMain.aws.sdk.kotlin.services.shield.model.Subscription.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of shield Show documentation
Show all versions of shield Show documentation
The AWS SDK for Kotlin client for Shield
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.shield.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* Information about the Shield Advanced subscription for an account.
*/
public class Subscription private constructor(builder: Builder) {
/**
* If `ENABLED`, the subscription will be automatically renewed at the end of the existing subscription period.
*
* When you initally create a subscription, `AutoRenew` is set to `ENABLED`. You can change this by submitting an `UpdateSubscription` request. If the `UpdateSubscription` request does not included a value for `AutoRenew`, the existing value for `AutoRenew` remains unchanged.
*/
public val autoRenew: aws.sdk.kotlin.services.shield.model.AutoRenew? = builder.autoRenew
/**
* The date and time your subscription will end.
*/
public val endTime: aws.smithy.kotlin.runtime.time.Instant? = builder.endTime
/**
* Specifies how many protections of a given type you can create.
*/
public val limits: List? = builder.limits
/**
* If `ENABLED`, the Shield Response Team (SRT) will use email and phone to notify contacts about escalations to the SRT and to initiate proactive customer support.
*
* If `PENDING`, you have requested proactive engagement and the request is pending. The status changes to `ENABLED` when your request is fully processed.
*
* If `DISABLED`, the SRT will not proactively notify contacts about escalations or to initiate proactive customer support.
*/
public val proactiveEngagementStatus: aws.sdk.kotlin.services.shield.model.ProactiveEngagementStatus? = builder.proactiveEngagementStatus
/**
* The start time of the subscription, in Unix time in seconds.
*/
public val startTime: aws.smithy.kotlin.runtime.time.Instant? = builder.startTime
/**
* The ARN (Amazon Resource Name) of the subscription.
*/
public val subscriptionArn: kotlin.String? = builder.subscriptionArn
/**
* Limits settings for your subscription.
*/
public val subscriptionLimits: aws.sdk.kotlin.services.shield.model.SubscriptionLimits? = builder.subscriptionLimits
/**
* The length, in seconds, of the Shield Advanced subscription for the account.
*/
public val timeCommitmentInSeconds: kotlin.Long = builder.timeCommitmentInSeconds
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.shield.model.Subscription = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Subscription(")
append("autoRenew=$autoRenew,")
append("endTime=$endTime,")
append("limits=$limits,")
append("proactiveEngagementStatus=$proactiveEngagementStatus,")
append("startTime=$startTime,")
append("subscriptionArn=$subscriptionArn,")
append("subscriptionLimits=$subscriptionLimits,")
append("timeCommitmentInSeconds=$timeCommitmentInSeconds")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = autoRenew?.hashCode() ?: 0
result = 31 * result + (endTime?.hashCode() ?: 0)
result = 31 * result + (limits?.hashCode() ?: 0)
result = 31 * result + (proactiveEngagementStatus?.hashCode() ?: 0)
result = 31 * result + (startTime?.hashCode() ?: 0)
result = 31 * result + (subscriptionArn?.hashCode() ?: 0)
result = 31 * result + (subscriptionLimits?.hashCode() ?: 0)
result = 31 * result + (timeCommitmentInSeconds.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Subscription
if (autoRenew != other.autoRenew) return false
if (endTime != other.endTime) return false
if (limits != other.limits) return false
if (proactiveEngagementStatus != other.proactiveEngagementStatus) return false
if (startTime != other.startTime) return false
if (subscriptionArn != other.subscriptionArn) return false
if (subscriptionLimits != other.subscriptionLimits) return false
if (timeCommitmentInSeconds != other.timeCommitmentInSeconds) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.shield.model.Subscription = Builder(this).apply(block).build()
public class Builder {
/**
* If `ENABLED`, the subscription will be automatically renewed at the end of the existing subscription period.
*
* When you initally create a subscription, `AutoRenew` is set to `ENABLED`. You can change this by submitting an `UpdateSubscription` request. If the `UpdateSubscription` request does not included a value for `AutoRenew`, the existing value for `AutoRenew` remains unchanged.
*/
public var autoRenew: aws.sdk.kotlin.services.shield.model.AutoRenew? = null
/**
* The date and time your subscription will end.
*/
public var endTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Specifies how many protections of a given type you can create.
*/
public var limits: List? = null
/**
* If `ENABLED`, the Shield Response Team (SRT) will use email and phone to notify contacts about escalations to the SRT and to initiate proactive customer support.
*
* If `PENDING`, you have requested proactive engagement and the request is pending. The status changes to `ENABLED` when your request is fully processed.
*
* If `DISABLED`, the SRT will not proactively notify contacts about escalations or to initiate proactive customer support.
*/
public var proactiveEngagementStatus: aws.sdk.kotlin.services.shield.model.ProactiveEngagementStatus? = null
/**
* The start time of the subscription, in Unix time in seconds.
*/
public var startTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The ARN (Amazon Resource Name) of the subscription.
*/
public var subscriptionArn: kotlin.String? = null
/**
* Limits settings for your subscription.
*/
public var subscriptionLimits: aws.sdk.kotlin.services.shield.model.SubscriptionLimits? = null
/**
* The length, in seconds, of the Shield Advanced subscription for the account.
*/
public var timeCommitmentInSeconds: kotlin.Long = 0L
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.shield.model.Subscription) : this() {
this.autoRenew = x.autoRenew
this.endTime = x.endTime
this.limits = x.limits
this.proactiveEngagementStatus = x.proactiveEngagementStatus
this.startTime = x.startTime
this.subscriptionArn = x.subscriptionArn
this.subscriptionLimits = x.subscriptionLimits
this.timeCommitmentInSeconds = x.timeCommitmentInSeconds
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.shield.model.Subscription = Subscription(this)
/**
* construct an [aws.sdk.kotlin.services.shield.model.SubscriptionLimits] inside the given [block]
*/
public fun subscriptionLimits(block: aws.sdk.kotlin.services.shield.model.SubscriptionLimits.Builder.() -> kotlin.Unit) {
this.subscriptionLimits = aws.sdk.kotlin.services.shield.model.SubscriptionLimits.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy