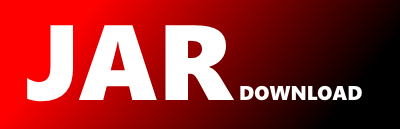
commonMain.aws.sdk.kotlin.services.signer.SignerClient.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of signer-jvm Show documentation
Show all versions of signer-jvm Show documentation
The AWS SDK for Kotlin client for signer
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.signer
import aws.sdk.kotlin.runtime.auth.credentials.DefaultChainCredentialsProvider
import aws.sdk.kotlin.runtime.auth.credentials.internal.borrow
import aws.sdk.kotlin.runtime.endpoint.AwsEndpointResolver
import aws.sdk.kotlin.runtime.region.resolveRegion
import aws.sdk.kotlin.services.signer.internal.DefaultEndpointResolver
import aws.sdk.kotlin.services.signer.model.*
import aws.smithy.kotlin.runtime.SdkClient
import aws.smithy.kotlin.runtime.auth.awscredentials.CredentialsProvider
import aws.smithy.kotlin.runtime.auth.awssigning.AwsSigner
import aws.smithy.kotlin.runtime.auth.awssigning.DefaultAwsSigner
import aws.smithy.kotlin.runtime.client.SdkLogMode
import aws.smithy.kotlin.runtime.config.IdempotencyTokenConfig
import aws.smithy.kotlin.runtime.config.IdempotencyTokenProvider
import aws.smithy.kotlin.runtime.config.SdkClientConfig
import aws.smithy.kotlin.runtime.http.config.HttpClientConfig
import aws.smithy.kotlin.runtime.http.endpoints.EndpointResolver
import aws.smithy.kotlin.runtime.http.engine.HttpClientEngine
import aws.smithy.kotlin.runtime.retries.RetryStrategy
import aws.smithy.kotlin.runtime.retries.StandardRetryStrategy
/**
* AWS Signer is a fully managed code signing service to help you ensure the trust and integrity of your code.
*
* AWS Signer supports the following applications:
*
* With *code signing for AWS Lambda*, you can sign AWS Lambda deployment packages. Integrated support is provided for Amazon S3, Amazon CloudWatch, and AWS CloudTrail. In order to sign code, you create a signing profile and then use Signer to sign Lambda zip files in S3.
*
* With *code signing for IoT*, you can sign code for any IoT device that is supported by AWS. IoT code signing is available for [Amazon FreeRTOS](http://docs.aws.amazon.com/freertos/latest/userguide/) and [AWS IoT Device Management](http://docs.aws.amazon.com/iot/latest/developerguide/), and is integrated with [AWS Certificate Manager (ACM)](http://docs.aws.amazon.com/acm/latest/userguide/). In order to sign code, you import a third-party code signing certificate using ACM, and use that to sign updates in Amazon FreeRTOS and AWS IoT Device Management.
*
* For more information about AWS Signer, see the [AWS Signer Developer Guide](http://docs.aws.amazon.com/signer/latest/developerguide/Welcome.html).
*/
interface SignerClient : SdkClient {
override val serviceName: String
get() = "signer"
/**
* SignerClient's configuration
*/
val config: Config
companion object {
operator fun invoke(block: Config.Builder.() -> Unit): SignerClient {
val config = Config.Builder().apply(block).build()
return DefaultSignerClient(config)
}
operator fun invoke(config: Config): SignerClient = DefaultSignerClient(config)
/**
* Construct a [SignerClient] by resolving the configuration from the current environment.
*/
suspend fun fromEnvironment(block: (Config.Builder.() -> Unit)? = null): SignerClient {
val builder = Config.Builder()
if (block != null) builder.apply(block)
builder.region = builder.region ?: resolveRegion()
return DefaultSignerClient(builder.build())
}
}
class Config private constructor(builder: Builder): HttpClientConfig, IdempotencyTokenConfig, SdkClientConfig {
val credentialsProvider: CredentialsProvider = builder.credentialsProvider?.borrow() ?: DefaultChainCredentialsProvider()
val endpointResolver: AwsEndpointResolver = builder.endpointResolver ?: DefaultEndpointResolver()
override val httpClientEngine: HttpClientEngine? = builder.httpClientEngine
override val idempotencyTokenProvider: IdempotencyTokenProvider? = builder.idempotencyTokenProvider
val region: String = requireNotNull(builder.region) { "region is a required configuration property" }
val retryStrategy: RetryStrategy = StandardRetryStrategy()
override val sdkLogMode: SdkLogMode = builder.sdkLogMode
val signer: AwsSigner = builder.signer ?: DefaultAwsSigner
companion object {
inline operator fun invoke(block: Builder.() -> kotlin.Unit): Config = Builder().apply(block).build()
}
class Builder {
/**
* The AWS credentials provider to use for authenticating requests. If not provided a
* [aws.sdk.kotlin.runtime.auth.credentials.DefaultChainCredentialsProvider] instance will be used.
* NOTE: The caller is responsible for managing the lifetime of the provider when set. The SDK
* client will not close it when the client is closed.
*/
var credentialsProvider: CredentialsProvider? = null
/**
* Determines the endpoint (hostname) to make requests to. When not provided a default
* resolver is configured automatically. This is an advanced client option.
*/
var endpointResolver: AwsEndpointResolver? = null
/**
* Override the default HTTP client engine used to make SDK requests (e.g. configure proxy behavior, timeouts, concurrency, etc).
* NOTE: The caller is responsible for managing the lifetime of the engine when set. The SDK
* client will not close it when the client is closed.
*/
var httpClientEngine: HttpClientEngine? = null
/**
* Override the default idempotency token generator. SDK clients will generate tokens for members
* that represent idempotent tokens when not explicitly set by the caller using this generator.
*/
var idempotencyTokenProvider: IdempotencyTokenProvider? = null
/**
* AWS region to make requests to
*/
var region: String? = null
/**
* Configure events that will be logged. By default clients will not output
* raw requests or responses. Use this setting to opt-in to additional debug logging.
*
* This can be used to configure logging of requests, responses, retries, etc of SDK clients.
*
* **NOTE**: Logging of raw requests or responses may leak sensitive information! It may also have
* performance considerations when dumping the request/response body. This is primarily a tool for
* debug purposes.
*/
var sdkLogMode: SdkLogMode = SdkLogMode.Default
/**
* The implementation of AWS signer to use for signing requests
*/
var signer: AwsSigner? = null
@PublishedApi
internal fun build(): Config = Config(this)
}
}
/**
* Adds cross-account permissions to a signing profile.
*/
suspend fun addProfilePermission(input: AddProfilePermissionRequest): AddProfilePermissionResponse
/**
* Changes the state of an `ACTIVE` signing profile to `CANCELED`. A canceled profile is still viewable with the `ListSigningProfiles` operation, but it cannot perform new signing jobs, and is deleted two years after cancelation.
*/
suspend fun cancelSigningProfile(input: CancelSigningProfileRequest): CancelSigningProfileResponse
/**
* Returns information about a specific code signing job. You specify the job by using the `jobId` value that is returned by the StartSigningJob operation.
*/
suspend fun describeSigningJob(input: DescribeSigningJobRequest): DescribeSigningJobResponse
/**
* Returns information on a specific signing platform.
*/
suspend fun getSigningPlatform(input: GetSigningPlatformRequest): GetSigningPlatformResponse
/**
* Returns information on a specific signing profile.
*/
suspend fun getSigningProfile(input: GetSigningProfileRequest): GetSigningProfileResponse
/**
* Lists the cross-account permissions associated with a signing profile.
*/
suspend fun listProfilePermissions(input: ListProfilePermissionsRequest): ListProfilePermissionsResponse
/**
* Lists all your signing jobs. You can use the `maxResults` parameter to limit the number of signing jobs that are returned in the response. If additional jobs remain to be listed, code signing returns a `nextToken` value. Use this value in subsequent calls to `ListSigningJobs` to fetch the remaining values. You can continue calling `ListSigningJobs` with your `maxResults` parameter and with new values that code signing returns in the `nextToken` parameter until all of your signing jobs have been returned.
*/
suspend fun listSigningJobs(input: ListSigningJobsRequest = ListSigningJobsRequest {}): ListSigningJobsResponse
/**
* Lists all signing platforms available in code signing that match the request parameters. If additional jobs remain to be listed, code signing returns a `nextToken` value. Use this value in subsequent calls to `ListSigningJobs` to fetch the remaining values. You can continue calling `ListSigningJobs` with your `maxResults` parameter and with new values that code signing returns in the `nextToken` parameter until all of your signing jobs have been returned.
*/
suspend fun listSigningPlatforms(input: ListSigningPlatformsRequest = ListSigningPlatformsRequest {}): ListSigningPlatformsResponse
/**
* Lists all available signing profiles in your AWS account. Returns only profiles with an `ACTIVE` status unless the `includeCanceled` request field is set to `true`. If additional jobs remain to be listed, code signing returns a `nextToken` value. Use this value in subsequent calls to `ListSigningJobs` to fetch the remaining values. You can continue calling `ListSigningJobs` with your `maxResults` parameter and with new values that code signing returns in the `nextToken` parameter until all of your signing jobs have been returned.
*/
suspend fun listSigningProfiles(input: ListSigningProfilesRequest = ListSigningProfilesRequest {}): ListSigningProfilesResponse
/**
* Returns a list of the tags associated with a signing profile resource.
*/
suspend fun listTagsForResource(input: ListTagsForResourceRequest): ListTagsForResourceResponse
/**
* Creates a signing profile. A signing profile is a code signing template that can be used to carry out a pre-defined signing job. For more information, see [http://docs.aws.amazon.com/signer/latest/developerguide/gs-profile.html](http://docs.aws.amazon.com/signer/latest/developerguide/gs-profile.html)
*/
suspend fun putSigningProfile(input: PutSigningProfileRequest): PutSigningProfileResponse
/**
* Removes cross-account permissions from a signing profile.
*/
suspend fun removeProfilePermission(input: RemoveProfilePermissionRequest): RemoveProfilePermissionResponse
/**
* Changes the state of a signing job to REVOKED. This indicates that the signature is no longer valid.
*/
suspend fun revokeSignature(input: RevokeSignatureRequest): RevokeSignatureResponse
/**
* Changes the state of a signing profile to REVOKED. This indicates that signatures generated using the signing profile after an effective start date are no longer valid.
*/
suspend fun revokeSigningProfile(input: RevokeSigningProfileRequest): RevokeSigningProfileResponse
/**
* Initiates a signing job to be performed on the code provided. Signing jobs are viewable by the `ListSigningJobs` operation for two years after they are performed. Note the following requirements:
* + You must create an Amazon S3 source bucket. For more information, see [Create a Bucket](http://docs.aws.amazon.com/AmazonS3/latest/gsg/CreatingABucket.html) in the *Amazon S3 Getting Started Guide*.
* + Your S3 source bucket must be version enabled.
* + You must create an S3 destination bucket. Code signing uses your S3 destination bucket to write your signed code.
* + You specify the name of the source and destination buckets when calling the `StartSigningJob` operation.
* + You must also specify a request token that identifies your request to code signing.
*
* You can call the DescribeSigningJob and the ListSigningJobs actions after you call `StartSigningJob`.
*
* For a Java example that shows how to use this action, see [http://docs.aws.amazon.com/acm/latest/userguide/](http://docs.aws.amazon.com/acm/latest/userguide/)
*/
suspend fun startSigningJob(input: StartSigningJobRequest): StartSigningJobResponse
/**
* Adds one or more tags to a signing profile. Tags are labels that you can use to identify and organize your AWS resources. Each tag consists of a key and an optional value. To specify the signing profile, use its Amazon Resource Name (ARN). To specify the tag, use a key-value pair.
*/
suspend fun tagResource(input: TagResourceRequest): TagResourceResponse
/**
* Removes one or more tags from a signing profile. To remove the tags, specify a list of tag keys.
*/
suspend fun untagResource(input: UntagResourceRequest): UntagResourceResponse
}
/**
* Adds cross-account permissions to a signing profile.
*/
suspend inline fun SignerClient.addProfilePermission(crossinline block: AddProfilePermissionRequest.Builder.() -> Unit) = addProfilePermission(AddProfilePermissionRequest.Builder().apply(block).build())
/**
* Changes the state of an `ACTIVE` signing profile to `CANCELED`. A canceled profile is still viewable with the `ListSigningProfiles` operation, but it cannot perform new signing jobs, and is deleted two years after cancelation.
*/
suspend inline fun SignerClient.cancelSigningProfile(crossinline block: CancelSigningProfileRequest.Builder.() -> Unit) = cancelSigningProfile(CancelSigningProfileRequest.Builder().apply(block).build())
/**
* Returns information about a specific code signing job. You specify the job by using the `jobId` value that is returned by the StartSigningJob operation.
*/
suspend inline fun SignerClient.describeSigningJob(crossinline block: DescribeSigningJobRequest.Builder.() -> Unit) = describeSigningJob(DescribeSigningJobRequest.Builder().apply(block).build())
/**
* Returns information on a specific signing platform.
*/
suspend inline fun SignerClient.getSigningPlatform(crossinline block: GetSigningPlatformRequest.Builder.() -> Unit) = getSigningPlatform(GetSigningPlatformRequest.Builder().apply(block).build())
/**
* Returns information on a specific signing profile.
*/
suspend inline fun SignerClient.getSigningProfile(crossinline block: GetSigningProfileRequest.Builder.() -> Unit) = getSigningProfile(GetSigningProfileRequest.Builder().apply(block).build())
/**
* Lists the cross-account permissions associated with a signing profile.
*/
suspend inline fun SignerClient.listProfilePermissions(crossinline block: ListProfilePermissionsRequest.Builder.() -> Unit) = listProfilePermissions(ListProfilePermissionsRequest.Builder().apply(block).build())
/**
* Lists all your signing jobs. You can use the `maxResults` parameter to limit the number of signing jobs that are returned in the response. If additional jobs remain to be listed, code signing returns a `nextToken` value. Use this value in subsequent calls to `ListSigningJobs` to fetch the remaining values. You can continue calling `ListSigningJobs` with your `maxResults` parameter and with new values that code signing returns in the `nextToken` parameter until all of your signing jobs have been returned.
*/
suspend inline fun SignerClient.listSigningJobs(crossinline block: ListSigningJobsRequest.Builder.() -> Unit) = listSigningJobs(ListSigningJobsRequest.Builder().apply(block).build())
/**
* Lists all signing platforms available in code signing that match the request parameters. If additional jobs remain to be listed, code signing returns a `nextToken` value. Use this value in subsequent calls to `ListSigningJobs` to fetch the remaining values. You can continue calling `ListSigningJobs` with your `maxResults` parameter and with new values that code signing returns in the `nextToken` parameter until all of your signing jobs have been returned.
*/
suspend inline fun SignerClient.listSigningPlatforms(crossinline block: ListSigningPlatformsRequest.Builder.() -> Unit) = listSigningPlatforms(ListSigningPlatformsRequest.Builder().apply(block).build())
/**
* Lists all available signing profiles in your AWS account. Returns only profiles with an `ACTIVE` status unless the `includeCanceled` request field is set to `true`. If additional jobs remain to be listed, code signing returns a `nextToken` value. Use this value in subsequent calls to `ListSigningJobs` to fetch the remaining values. You can continue calling `ListSigningJobs` with your `maxResults` parameter and with new values that code signing returns in the `nextToken` parameter until all of your signing jobs have been returned.
*/
suspend inline fun SignerClient.listSigningProfiles(crossinline block: ListSigningProfilesRequest.Builder.() -> Unit) = listSigningProfiles(ListSigningProfilesRequest.Builder().apply(block).build())
/**
* Returns a list of the tags associated with a signing profile resource.
*/
suspend inline fun SignerClient.listTagsForResource(crossinline block: ListTagsForResourceRequest.Builder.() -> Unit) = listTagsForResource(ListTagsForResourceRequest.Builder().apply(block).build())
/**
* Creates a signing profile. A signing profile is a code signing template that can be used to carry out a pre-defined signing job. For more information, see [http://docs.aws.amazon.com/signer/latest/developerguide/gs-profile.html](http://docs.aws.amazon.com/signer/latest/developerguide/gs-profile.html)
*/
suspend inline fun SignerClient.putSigningProfile(crossinline block: PutSigningProfileRequest.Builder.() -> Unit) = putSigningProfile(PutSigningProfileRequest.Builder().apply(block).build())
/**
* Removes cross-account permissions from a signing profile.
*/
suspend inline fun SignerClient.removeProfilePermission(crossinline block: RemoveProfilePermissionRequest.Builder.() -> Unit) = removeProfilePermission(RemoveProfilePermissionRequest.Builder().apply(block).build())
/**
* Changes the state of a signing job to REVOKED. This indicates that the signature is no longer valid.
*/
suspend inline fun SignerClient.revokeSignature(crossinline block: RevokeSignatureRequest.Builder.() -> Unit) = revokeSignature(RevokeSignatureRequest.Builder().apply(block).build())
/**
* Changes the state of a signing profile to REVOKED. This indicates that signatures generated using the signing profile after an effective start date are no longer valid.
*/
suspend inline fun SignerClient.revokeSigningProfile(crossinline block: RevokeSigningProfileRequest.Builder.() -> Unit) = revokeSigningProfile(RevokeSigningProfileRequest.Builder().apply(block).build())
/**
* Initiates a signing job to be performed on the code provided. Signing jobs are viewable by the `ListSigningJobs` operation for two years after they are performed. Note the following requirements:
* + You must create an Amazon S3 source bucket. For more information, see [Create a Bucket](http://docs.aws.amazon.com/AmazonS3/latest/gsg/CreatingABucket.html) in the *Amazon S3 Getting Started Guide*.
* + Your S3 source bucket must be version enabled.
* + You must create an S3 destination bucket. Code signing uses your S3 destination bucket to write your signed code.
* + You specify the name of the source and destination buckets when calling the `StartSigningJob` operation.
* + You must also specify a request token that identifies your request to code signing.
*
* You can call the DescribeSigningJob and the ListSigningJobs actions after you call `StartSigningJob`.
*
* For a Java example that shows how to use this action, see [http://docs.aws.amazon.com/acm/latest/userguide/](http://docs.aws.amazon.com/acm/latest/userguide/)
*/
suspend inline fun SignerClient.startSigningJob(crossinline block: StartSigningJobRequest.Builder.() -> Unit) = startSigningJob(StartSigningJobRequest.Builder().apply(block).build())
/**
* Adds one or more tags to a signing profile. Tags are labels that you can use to identify and organize your AWS resources. Each tag consists of a key and an optional value. To specify the signing profile, use its Amazon Resource Name (ARN). To specify the tag, use a key-value pair.
*/
suspend inline fun SignerClient.tagResource(crossinline block: TagResourceRequest.Builder.() -> Unit) = tagResource(TagResourceRequest.Builder().apply(block).build())
/**
* Removes one or more tags from a signing profile. To remove the tags, specify a list of tag keys.
*/
suspend inline fun SignerClient.untagResource(crossinline block: UntagResourceRequest.Builder.() -> Unit) = untagResource(UntagResourceRequest.Builder().apply(block).build())
© 2015 - 2025 Weber Informatics LLC | Privacy Policy