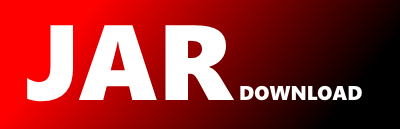
commonMain.aws.sdk.kotlin.services.snowdevicemanagement.model.Instance.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snowdevicemanagement-jvm Show documentation
Show all versions of snowdevicemanagement-jvm Show documentation
The AWS SDK for Kotlin client for Snow Device Management
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.snowdevicemanagement.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* The description of an instance. Currently, Amazon EC2 instances are the only supported instance type.
*/
public class Instance private constructor(builder: Builder) {
/**
* The Amazon Machine Image (AMI) launch index, which you can use to find this instance in the launch group.
*/
public val amiLaunchIndex: kotlin.Int? = builder.amiLaunchIndex
/**
* Any block device mapping entries for the instance.
*/
public val blockDeviceMappings: List? = builder.blockDeviceMappings
/**
* The CPU options for the instance.
*/
public val cpuOptions: aws.sdk.kotlin.services.snowdevicemanagement.model.CpuOptions? = builder.cpuOptions
/**
* When the instance was created.
*/
public val createdAt: aws.smithy.kotlin.runtime.time.Instant? = builder.createdAt
/**
* The ID of the AMI used to launch the instance.
*/
public val imageId: kotlin.String? = builder.imageId
/**
* The ID of the instance.
*/
public val instanceId: kotlin.String? = builder.instanceId
/**
* The instance type.
*/
public val instanceType: kotlin.String? = builder.instanceType
/**
* The private IPv4 address assigned to the instance.
*/
public val privateIpAddress: kotlin.String? = builder.privateIpAddress
/**
* The public IPv4 address assigned to the instance.
*/
public val publicIpAddress: kotlin.String? = builder.publicIpAddress
/**
* The device name of the root device volume (for example, `/dev/sda1`).
*/
public val rootDeviceName: kotlin.String? = builder.rootDeviceName
/**
* The security groups for the instance.
*/
public val securityGroups: List? = builder.securityGroups
/**
* The description of the current state of an instance.
*/
public val state: aws.sdk.kotlin.services.snowdevicemanagement.model.InstanceState? = builder.state
/**
* When the instance was last updated.
*/
public val updatedAt: aws.smithy.kotlin.runtime.time.Instant? = builder.updatedAt
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.snowdevicemanagement.model.Instance = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Instance(")
append("amiLaunchIndex=$amiLaunchIndex,")
append("blockDeviceMappings=$blockDeviceMappings,")
append("cpuOptions=$cpuOptions,")
append("createdAt=$createdAt,")
append("imageId=$imageId,")
append("instanceId=$instanceId,")
append("instanceType=$instanceType,")
append("privateIpAddress=$privateIpAddress,")
append("publicIpAddress=$publicIpAddress,")
append("rootDeviceName=$rootDeviceName,")
append("securityGroups=$securityGroups,")
append("state=$state,")
append("updatedAt=$updatedAt")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = amiLaunchIndex ?: 0
result = 31 * result + (blockDeviceMappings?.hashCode() ?: 0)
result = 31 * result + (cpuOptions?.hashCode() ?: 0)
result = 31 * result + (createdAt?.hashCode() ?: 0)
result = 31 * result + (imageId?.hashCode() ?: 0)
result = 31 * result + (instanceId?.hashCode() ?: 0)
result = 31 * result + (instanceType?.hashCode() ?: 0)
result = 31 * result + (privateIpAddress?.hashCode() ?: 0)
result = 31 * result + (publicIpAddress?.hashCode() ?: 0)
result = 31 * result + (rootDeviceName?.hashCode() ?: 0)
result = 31 * result + (securityGroups?.hashCode() ?: 0)
result = 31 * result + (state?.hashCode() ?: 0)
result = 31 * result + (updatedAt?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Instance
if (amiLaunchIndex != other.amiLaunchIndex) return false
if (blockDeviceMappings != other.blockDeviceMappings) return false
if (cpuOptions != other.cpuOptions) return false
if (createdAt != other.createdAt) return false
if (imageId != other.imageId) return false
if (instanceId != other.instanceId) return false
if (instanceType != other.instanceType) return false
if (privateIpAddress != other.privateIpAddress) return false
if (publicIpAddress != other.publicIpAddress) return false
if (rootDeviceName != other.rootDeviceName) return false
if (securityGroups != other.securityGroups) return false
if (state != other.state) return false
if (updatedAt != other.updatedAt) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.snowdevicemanagement.model.Instance = Builder(this).apply(block).build()
public class Builder {
/**
* The Amazon Machine Image (AMI) launch index, which you can use to find this instance in the launch group.
*/
public var amiLaunchIndex: kotlin.Int? = null
/**
* Any block device mapping entries for the instance.
*/
public var blockDeviceMappings: List? = null
/**
* The CPU options for the instance.
*/
public var cpuOptions: aws.sdk.kotlin.services.snowdevicemanagement.model.CpuOptions? = null
/**
* When the instance was created.
*/
public var createdAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The ID of the AMI used to launch the instance.
*/
public var imageId: kotlin.String? = null
/**
* The ID of the instance.
*/
public var instanceId: kotlin.String? = null
/**
* The instance type.
*/
public var instanceType: kotlin.String? = null
/**
* The private IPv4 address assigned to the instance.
*/
public var privateIpAddress: kotlin.String? = null
/**
* The public IPv4 address assigned to the instance.
*/
public var publicIpAddress: kotlin.String? = null
/**
* The device name of the root device volume (for example, `/dev/sda1`).
*/
public var rootDeviceName: kotlin.String? = null
/**
* The security groups for the instance.
*/
public var securityGroups: List? = null
/**
* The description of the current state of an instance.
*/
public var state: aws.sdk.kotlin.services.snowdevicemanagement.model.InstanceState? = null
/**
* When the instance was last updated.
*/
public var updatedAt: aws.smithy.kotlin.runtime.time.Instant? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.snowdevicemanagement.model.Instance) : this() {
this.amiLaunchIndex = x.amiLaunchIndex
this.blockDeviceMappings = x.blockDeviceMappings
this.cpuOptions = x.cpuOptions
this.createdAt = x.createdAt
this.imageId = x.imageId
this.instanceId = x.instanceId
this.instanceType = x.instanceType
this.privateIpAddress = x.privateIpAddress
this.publicIpAddress = x.publicIpAddress
this.rootDeviceName = x.rootDeviceName
this.securityGroups = x.securityGroups
this.state = x.state
this.updatedAt = x.updatedAt
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.snowdevicemanagement.model.Instance = Instance(this)
/**
* construct an [aws.sdk.kotlin.services.snowdevicemanagement.model.CpuOptions] inside the given [block]
*/
public fun cpuOptions(block: aws.sdk.kotlin.services.snowdevicemanagement.model.CpuOptions.Builder.() -> kotlin.Unit) {
this.cpuOptions = aws.sdk.kotlin.services.snowdevicemanagement.model.CpuOptions.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.snowdevicemanagement.model.InstanceState] inside the given [block]
*/
public fun state(block: aws.sdk.kotlin.services.snowdevicemanagement.model.InstanceState.Builder.() -> kotlin.Unit) {
this.state = aws.sdk.kotlin.services.snowdevicemanagement.model.InstanceState.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy