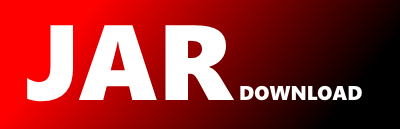
commonMain.aws.sdk.kotlin.services.snowdevicemanagement.model.DescribeDeviceResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snowdevicemanagement-jvm Show documentation
Show all versions of snowdevicemanagement-jvm Show documentation
The AWS SDK for Kotlin client for Snow Device Management
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.snowdevicemanagement.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
public class DescribeDeviceResponse private constructor(builder: Builder) {
/**
* The ID of the job used when ordering the device.
*/
public val associatedWithJob: kotlin.String? = builder.associatedWithJob
/**
* The hardware specifications of the device.
*/
public val deviceCapacities: List? = builder.deviceCapacities
/**
* The current state of the device.
*/
public val deviceState: aws.sdk.kotlin.services.snowdevicemanagement.model.UnlockState? = builder.deviceState
/**
* The type of Amazon Web Services Snow Family device.
*/
public val deviceType: kotlin.String? = builder.deviceType
/**
* When the device last contacted the Amazon Web Services Cloud. Indicates that the device is online.
*/
public val lastReachedOutAt: aws.smithy.kotlin.runtime.time.Instant? = builder.lastReachedOutAt
/**
* When the device last pushed an update to the Amazon Web Services Cloud. Indicates when the device cache was refreshed.
*/
public val lastUpdatedAt: aws.smithy.kotlin.runtime.time.Instant? = builder.lastUpdatedAt
/**
* The Amazon Resource Name (ARN) of the device.
*/
public val managedDeviceArn: kotlin.String? = builder.managedDeviceArn
/**
* The ID of the device that you checked the information for.
*/
public val managedDeviceId: kotlin.String? = builder.managedDeviceId
/**
* The network interfaces available on the device.
*/
public val physicalNetworkInterfaces: List? = builder.physicalNetworkInterfaces
/**
* The software installed on the device.
*/
public val software: aws.sdk.kotlin.services.snowdevicemanagement.model.SoftwareInformation? = builder.software
/**
* Optional metadata that you assign to a resource. You can use tags to categorize a resource in different ways, such as by purpose, owner, or environment.
*/
public val tags: Map? = builder.tags
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.snowdevicemanagement.model.DescribeDeviceResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeDeviceResponse(")
append("associatedWithJob=$associatedWithJob,")
append("deviceCapacities=$deviceCapacities,")
append("deviceState=$deviceState,")
append("deviceType=$deviceType,")
append("lastReachedOutAt=$lastReachedOutAt,")
append("lastUpdatedAt=$lastUpdatedAt,")
append("managedDeviceArn=$managedDeviceArn,")
append("managedDeviceId=$managedDeviceId,")
append("physicalNetworkInterfaces=$physicalNetworkInterfaces,")
append("software=$software,")
append("tags=$tags")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = associatedWithJob?.hashCode() ?: 0
result = 31 * result + (deviceCapacities?.hashCode() ?: 0)
result = 31 * result + (deviceState?.hashCode() ?: 0)
result = 31 * result + (deviceType?.hashCode() ?: 0)
result = 31 * result + (lastReachedOutAt?.hashCode() ?: 0)
result = 31 * result + (lastUpdatedAt?.hashCode() ?: 0)
result = 31 * result + (managedDeviceArn?.hashCode() ?: 0)
result = 31 * result + (managedDeviceId?.hashCode() ?: 0)
result = 31 * result + (physicalNetworkInterfaces?.hashCode() ?: 0)
result = 31 * result + (software?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribeDeviceResponse
if (associatedWithJob != other.associatedWithJob) return false
if (deviceCapacities != other.deviceCapacities) return false
if (deviceState != other.deviceState) return false
if (deviceType != other.deviceType) return false
if (lastReachedOutAt != other.lastReachedOutAt) return false
if (lastUpdatedAt != other.lastUpdatedAt) return false
if (managedDeviceArn != other.managedDeviceArn) return false
if (managedDeviceId != other.managedDeviceId) return false
if (physicalNetworkInterfaces != other.physicalNetworkInterfaces) return false
if (software != other.software) return false
if (tags != other.tags) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.snowdevicemanagement.model.DescribeDeviceResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The ID of the job used when ordering the device.
*/
public var associatedWithJob: kotlin.String? = null
/**
* The hardware specifications of the device.
*/
public var deviceCapacities: List? = null
/**
* The current state of the device.
*/
public var deviceState: aws.sdk.kotlin.services.snowdevicemanagement.model.UnlockState? = null
/**
* The type of Amazon Web Services Snow Family device.
*/
public var deviceType: kotlin.String? = null
/**
* When the device last contacted the Amazon Web Services Cloud. Indicates that the device is online.
*/
public var lastReachedOutAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* When the device last pushed an update to the Amazon Web Services Cloud. Indicates when the device cache was refreshed.
*/
public var lastUpdatedAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The Amazon Resource Name (ARN) of the device.
*/
public var managedDeviceArn: kotlin.String? = null
/**
* The ID of the device that you checked the information for.
*/
public var managedDeviceId: kotlin.String? = null
/**
* The network interfaces available on the device.
*/
public var physicalNetworkInterfaces: List? = null
/**
* The software installed on the device.
*/
public var software: aws.sdk.kotlin.services.snowdevicemanagement.model.SoftwareInformation? = null
/**
* Optional metadata that you assign to a resource. You can use tags to categorize a resource in different ways, such as by purpose, owner, or environment.
*/
public var tags: Map? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.snowdevicemanagement.model.DescribeDeviceResponse) : this() {
this.associatedWithJob = x.associatedWithJob
this.deviceCapacities = x.deviceCapacities
this.deviceState = x.deviceState
this.deviceType = x.deviceType
this.lastReachedOutAt = x.lastReachedOutAt
this.lastUpdatedAt = x.lastUpdatedAt
this.managedDeviceArn = x.managedDeviceArn
this.managedDeviceId = x.managedDeviceId
this.physicalNetworkInterfaces = x.physicalNetworkInterfaces
this.software = x.software
this.tags = x.tags
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.snowdevicemanagement.model.DescribeDeviceResponse = DescribeDeviceResponse(this)
/**
* construct an [aws.sdk.kotlin.services.snowdevicemanagement.model.SoftwareInformation] inside the given [block]
*/
public fun software(block: aws.sdk.kotlin.services.snowdevicemanagement.model.SoftwareInformation.Builder.() -> kotlin.Unit) {
this.software = aws.sdk.kotlin.services.snowdevicemanagement.model.SoftwareInformation.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy