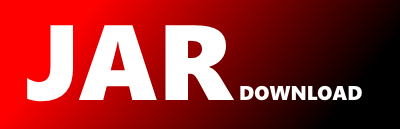
commonMain.aws.sdk.kotlin.services.snowdevicemanagement.model.DescribeExecutionResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snowdevicemanagement Show documentation
Show all versions of snowdevicemanagement Show documentation
The AWS Kotlin client for Snow Device Management
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.snowdevicemanagement.model
import aws.smithy.kotlin.runtime.time.Instant
public class DescribeExecutionResponse private constructor(builder: Builder) {
/**
* The ID of the execution.
*/
public val executionId: kotlin.String? = builder.executionId
/**
* When the status of the execution was last updated.
*/
public val lastUpdatedAt: aws.smithy.kotlin.runtime.time.Instant? = builder.lastUpdatedAt
/**
* The ID of the managed device that the task is being executed on.
*/
public val managedDeviceId: kotlin.String? = builder.managedDeviceId
/**
* When the execution began.
*/
public val startedAt: aws.smithy.kotlin.runtime.time.Instant? = builder.startedAt
/**
* The current state of the execution.
*/
public val state: aws.sdk.kotlin.services.snowdevicemanagement.model.ExecutionState? = builder.state
/**
* The ID of the task being executed on the device.
*/
public val taskId: kotlin.String? = builder.taskId
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.snowdevicemanagement.model.DescribeExecutionResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeExecutionResponse(")
append("executionId=$executionId,")
append("lastUpdatedAt=$lastUpdatedAt,")
append("managedDeviceId=$managedDeviceId,")
append("startedAt=$startedAt,")
append("state=$state,")
append("taskId=$taskId")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = executionId?.hashCode() ?: 0
result = 31 * result + (lastUpdatedAt?.hashCode() ?: 0)
result = 31 * result + (managedDeviceId?.hashCode() ?: 0)
result = 31 * result + (startedAt?.hashCode() ?: 0)
result = 31 * result + (state?.hashCode() ?: 0)
result = 31 * result + (taskId?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribeExecutionResponse
if (executionId != other.executionId) return false
if (lastUpdatedAt != other.lastUpdatedAt) return false
if (managedDeviceId != other.managedDeviceId) return false
if (startedAt != other.startedAt) return false
if (state != other.state) return false
if (taskId != other.taskId) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.snowdevicemanagement.model.DescribeExecutionResponse = Builder(this).apply(block).build()
public class Builder {
/**
* The ID of the execution.
*/
public var executionId: kotlin.String? = null
/**
* When the status of the execution was last updated.
*/
public var lastUpdatedAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The ID of the managed device that the task is being executed on.
*/
public var managedDeviceId: kotlin.String? = null
/**
* When the execution began.
*/
public var startedAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The current state of the execution.
*/
public var state: aws.sdk.kotlin.services.snowdevicemanagement.model.ExecutionState? = null
/**
* The ID of the task being executed on the device.
*/
public var taskId: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.snowdevicemanagement.model.DescribeExecutionResponse) : this() {
this.executionId = x.executionId
this.lastUpdatedAt = x.lastUpdatedAt
this.managedDeviceId = x.managedDeviceId
this.startedAt = x.startedAt
this.state = x.state
this.taskId = x.taskId
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.snowdevicemanagement.model.DescribeExecutionResponse = DescribeExecutionResponse(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy