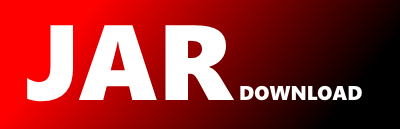
commonMain.aws.sdk.kotlin.services.snowdevicemanagement.DefaultSnowDeviceManagementClient.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snowdevicemanagement Show documentation
Show all versions of snowdevicemanagement Show documentation
The AWS Kotlin client for Snow Device Management
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.snowdevicemanagement
import aws.sdk.kotlin.runtime.client.AwsClientOption
import aws.sdk.kotlin.runtime.http.ApiMetadata
import aws.sdk.kotlin.runtime.http.AwsUserAgentMetadata
import aws.sdk.kotlin.runtime.http.interceptors.AwsSpanInterceptor
import aws.sdk.kotlin.runtime.http.middleware.AwsRetryHeaderMiddleware
import aws.sdk.kotlin.runtime.http.middleware.RecursionDetection
import aws.sdk.kotlin.runtime.http.middleware.UserAgent
import aws.sdk.kotlin.services.snowdevicemanagement.auth.AuthSchemeProviderAdapter
import aws.sdk.kotlin.services.snowdevicemanagement.auth.IdentityProviderConfigAdapter
import aws.sdk.kotlin.services.snowdevicemanagement.endpoints.internal.EndpointResolverAdapter
import aws.sdk.kotlin.services.snowdevicemanagement.model.*
import aws.sdk.kotlin.services.snowdevicemanagement.transform.*
import aws.smithy.kotlin.runtime.auth.AuthSchemeId
import aws.smithy.kotlin.runtime.auth.awssigning.AwsSigningAttributes
import aws.smithy.kotlin.runtime.auth.awssigning.DefaultAwsSigner
import aws.smithy.kotlin.runtime.client.SdkClientOption
import aws.smithy.kotlin.runtime.http.SdkHttpClient
import aws.smithy.kotlin.runtime.http.auth.HttpAuthScheme
import aws.smithy.kotlin.runtime.http.auth.SigV4AuthScheme
import aws.smithy.kotlin.runtime.http.operation.OperationAuthConfig
import aws.smithy.kotlin.runtime.http.operation.OperationMetrics
import aws.smithy.kotlin.runtime.http.operation.SdkHttpOperation
import aws.smithy.kotlin.runtime.http.operation.context
import aws.smithy.kotlin.runtime.http.operation.roundTrip
import aws.smithy.kotlin.runtime.http.operation.telemetry
import aws.smithy.kotlin.runtime.io.SdkManagedGroup
import aws.smithy.kotlin.runtime.io.addIfManaged
import aws.smithy.kotlin.runtime.operation.ExecutionContext
import aws.smithy.kotlin.runtime.util.attributesOf
import aws.smithy.kotlin.runtime.util.putIfAbsent
import aws.smithy.kotlin.runtime.util.putIfAbsentNotNull
public const val ServiceApiVersion: String = "2021-08-04"
internal class DefaultSnowDeviceManagementClient(override val config: SnowDeviceManagementClient.Config) : SnowDeviceManagementClient {
private val managedResources = SdkManagedGroup()
private val client = SdkHttpClient(config.httpClient)
private val identityProviderConfig = IdentityProviderConfigAdapter(config)
private val configuredAuthSchemes = with(config.authSchemes.associateBy(HttpAuthScheme::schemeId).toMutableMap()){
getOrPut(AuthSchemeId.AwsSigV4){
SigV4AuthScheme(DefaultAwsSigner, "snow-device-management")
}
toMap()
}
private val telemetryScope = "aws.sdk.kotlin.services.snowdevicemanagement"
private val opMetrics = OperationMetrics(telemetryScope, config.telemetryProvider)
init {
managedResources.addIfManaged(config.httpClient)
managedResources.addIfManaged(config.credentialsProvider)
}
private val awsUserAgentMetadata = AwsUserAgentMetadata.fromEnvironment(ApiMetadata(ServiceId, SdkVersion))
/**
* Sends a cancel request for a specified task. You can cancel a task only if it's still in a `QUEUED` state. Tasks that are already running can't be cancelled.
*
* A task might still run if it's processed from the queue before the `CancelTask` operation changes the task's state.
*/
override suspend fun cancelTask(input: CancelTaskRequest): CancelTaskResponse {
val op = SdkHttpOperation.build {
serializer = CancelTaskOperationSerializer()
deserializer = CancelTaskOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "CancelTask"
serviceName = ServiceId
}
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Instructs one or more devices to start a task, such as unlocking or rebooting.
*/
override suspend fun createTask(input: CreateTaskRequest): CreateTaskResponse {
val op = SdkHttpOperation.build {
serializer = CreateTaskOperationSerializer()
deserializer = CreateTaskOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "CreateTask"
serviceName = ServiceId
}
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Checks device-specific information, such as the device type, software version, IP addresses, and lock status.
*/
override suspend fun describeDevice(input: DescribeDeviceRequest): DescribeDeviceResponse {
val op = SdkHttpOperation.build {
serializer = DescribeDeviceOperationSerializer()
deserializer = DescribeDeviceOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "DescribeDevice"
serviceName = ServiceId
}
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Checks the current state of the Amazon EC2 instances. The output is similar to `describeDevice`, but the results are sourced from the device cache in the Amazon Web Services Cloud and include a subset of the available fields.
*/
override suspend fun describeDeviceEc2Instances(input: DescribeDeviceEc2InstancesRequest): DescribeDeviceEc2InstancesResponse {
val op = SdkHttpOperation.build {
serializer = DescribeDeviceEc2InstancesOperationSerializer()
deserializer = DescribeDeviceEc2InstancesOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "DescribeDeviceEc2Instances"
serviceName = ServiceId
}
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Checks the status of a remote task running on one or more target devices.
*/
override suspend fun describeExecution(input: DescribeExecutionRequest): DescribeExecutionResponse {
val op = SdkHttpOperation.build {
serializer = DescribeExecutionOperationSerializer()
deserializer = DescribeExecutionOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "DescribeExecution"
serviceName = ServiceId
}
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Checks the metadata for a given task on a device.
*/
override suspend fun describeTask(input: DescribeTaskRequest): DescribeTaskResponse {
val op = SdkHttpOperation.build {
serializer = DescribeTaskOperationSerializer()
deserializer = DescribeTaskOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "DescribeTask"
serviceName = ServiceId
}
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns a list of the Amazon Web Services resources available for a device. Currently, Amazon EC2 instances are the only supported resource type.
*/
override suspend fun listDeviceResources(input: ListDeviceResourcesRequest): ListDeviceResourcesResponse {
val op = SdkHttpOperation.build {
serializer = ListDeviceResourcesOperationSerializer()
deserializer = ListDeviceResourcesOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "ListDeviceResources"
serviceName = ServiceId
}
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns a list of all devices on your Amazon Web Services account that have Amazon Web Services Snow Device Management enabled in the Amazon Web Services Region where the command is run.
*/
override suspend fun listDevices(input: ListDevicesRequest): ListDevicesResponse {
val op = SdkHttpOperation.build {
serializer = ListDevicesOperationSerializer()
deserializer = ListDevicesOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "ListDevices"
serviceName = ServiceId
}
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns the status of tasks for one or more target devices.
*/
override suspend fun listExecutions(input: ListExecutionsRequest): ListExecutionsResponse {
val op = SdkHttpOperation.build {
serializer = ListExecutionsOperationSerializer()
deserializer = ListExecutionsOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "ListExecutions"
serviceName = ServiceId
}
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns a list of tags for a managed device or task.
*/
override suspend fun listTagsForResource(input: ListTagsForResourceRequest): ListTagsForResourceResponse {
val op = SdkHttpOperation.build {
serializer = ListTagsForResourceOperationSerializer()
deserializer = ListTagsForResourceOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "ListTagsForResource"
serviceName = ServiceId
}
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Returns a list of tasks that can be filtered by state.
*/
override suspend fun listTasks(input: ListTasksRequest): ListTasksResponse {
val op = SdkHttpOperation.build {
serializer = ListTasksOperationSerializer()
deserializer = ListTasksOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "ListTasks"
serviceName = ServiceId
}
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Adds or replaces tags on a device or task.
*/
override suspend fun tagResource(input: TagResourceRequest): TagResourceResponse {
val op = SdkHttpOperation.build {
serializer = TagResourceOperationSerializer()
deserializer = TagResourceOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "TagResource"
serviceName = ServiceId
}
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
/**
* Removes a tag from a device or task.
*/
override suspend fun untagResource(input: UntagResourceRequest): UntagResourceResponse {
val op = SdkHttpOperation.build {
serializer = UntagResourceOperationSerializer()
deserializer = UntagResourceOperationDeserializer()
context {
expectedHttpStatus = 200
operationName = "UntagResource"
serviceName = ServiceId
}
telemetry {
provider = config.telemetryProvider
scope = telemetryScope
metrics = opMetrics
attributes = attributesOf {
"rpc.system" to "aws-api"
}
}
execution.auth = OperationAuthConfig(AuthSchemeProviderAdapter, configuredAuthSchemes, identityProviderConfig)
execution.endpointResolver = EndpointResolverAdapter(config)
execution.retryStrategy = config.retryStrategy
}
op.execution.retryPolicy = config.retryPolicy
mergeServiceDefaults(op.context)
op.interceptors.add(AwsSpanInterceptor)
op.install(AwsRetryHeaderMiddleware())
op.install(UserAgent(awsUserAgentMetadata))
op.install(RecursionDetection())
op.interceptors.addAll(config.interceptors)
return op.roundTrip(client, input)
}
override fun close() {
managedResources.unshareAll()
}
/**
* merge the defaults configured for the service into the execution context before firing off a request
*/
private fun mergeServiceDefaults(ctx: ExecutionContext) {
ctx.putIfAbsentNotNull(AwsClientOption.Region, config.region)
ctx.putIfAbsent(SdkClientOption.ClientName, config.clientName)
ctx.putIfAbsent(SdkClientOption.LogMode, config.logMode)
ctx.putIfAbsent(AwsSigningAttributes.SigningService, "snow-device-management")
ctx.putIfAbsentNotNull(AwsSigningAttributes.SigningRegion, config.region)
ctx.putIfAbsent(AwsSigningAttributes.CredentialsProvider, config.credentialsProvider)
ctx.putIfAbsentNotNull(SdkClientOption.IdempotencyTokenProvider, config.idempotencyTokenProvider)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy