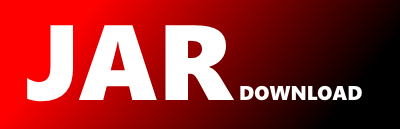
commonMain.aws.sdk.kotlin.services.snowdevicemanagement.model.DescribeTaskResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snowdevicemanagement Show documentation
Show all versions of snowdevicemanagement Show documentation
The AWS Kotlin client for Snow Device Management
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.snowdevicemanagement.model
import aws.smithy.kotlin.runtime.time.Instant
public class DescribeTaskResponse private constructor(builder: Builder) {
/**
* When the task was completed.
*/
public val completedAt: aws.smithy.kotlin.runtime.time.Instant? = builder.completedAt
/**
* When the `CreateTask` operation was called.
*/
public val createdAt: aws.smithy.kotlin.runtime.time.Instant? = builder.createdAt
/**
* The description provided of the task and managed devices.
*/
public val description: kotlin.String? = builder.description
/**
* When the state of the task was last updated.
*/
public val lastUpdatedAt: aws.smithy.kotlin.runtime.time.Instant? = builder.lastUpdatedAt
/**
* The current state of the task.
*/
public val state: aws.sdk.kotlin.services.snowdevicemanagement.model.TaskState? = builder.state
/**
* Optional metadata that you assign to a resource. You can use tags to categorize a resource in different ways, such as by purpose, owner, or environment.
*/
public val tags: Map? = builder.tags
/**
* The managed devices that the task was sent to.
*/
public val targets: List? = builder.targets
/**
* The Amazon Resource Name (ARN) of the task.
*/
public val taskArn: kotlin.String? = builder.taskArn
/**
* The ID of the task.
*/
public val taskId: kotlin.String? = builder.taskId
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.snowdevicemanagement.model.DescribeTaskResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DescribeTaskResponse(")
append("completedAt=$completedAt,")
append("createdAt=$createdAt,")
append("description=$description,")
append("lastUpdatedAt=$lastUpdatedAt,")
append("state=$state,")
append("tags=$tags,")
append("targets=$targets,")
append("taskArn=$taskArn,")
append("taskId=$taskId")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = completedAt?.hashCode() ?: 0
result = 31 * result + (createdAt?.hashCode() ?: 0)
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (lastUpdatedAt?.hashCode() ?: 0)
result = 31 * result + (state?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (targets?.hashCode() ?: 0)
result = 31 * result + (taskArn?.hashCode() ?: 0)
result = 31 * result + (taskId?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DescribeTaskResponse
if (completedAt != other.completedAt) return false
if (createdAt != other.createdAt) return false
if (description != other.description) return false
if (lastUpdatedAt != other.lastUpdatedAt) return false
if (state != other.state) return false
if (tags != other.tags) return false
if (targets != other.targets) return false
if (taskArn != other.taskArn) return false
if (taskId != other.taskId) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.snowdevicemanagement.model.DescribeTaskResponse = Builder(this).apply(block).build()
public class Builder {
/**
* When the task was completed.
*/
public var completedAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* When the `CreateTask` operation was called.
*/
public var createdAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The description provided of the task and managed devices.
*/
public var description: kotlin.String? = null
/**
* When the state of the task was last updated.
*/
public var lastUpdatedAt: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The current state of the task.
*/
public var state: aws.sdk.kotlin.services.snowdevicemanagement.model.TaskState? = null
/**
* Optional metadata that you assign to a resource. You can use tags to categorize a resource in different ways, such as by purpose, owner, or environment.
*/
public var tags: Map? = null
/**
* The managed devices that the task was sent to.
*/
public var targets: List? = null
/**
* The Amazon Resource Name (ARN) of the task.
*/
public var taskArn: kotlin.String? = null
/**
* The ID of the task.
*/
public var taskId: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.snowdevicemanagement.model.DescribeTaskResponse) : this() {
this.completedAt = x.completedAt
this.createdAt = x.createdAt
this.description = x.description
this.lastUpdatedAt = x.lastUpdatedAt
this.state = x.state
this.tags = x.tags
this.targets = x.targets
this.taskArn = x.taskArn
this.taskId = x.taskId
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.snowdevicemanagement.model.DescribeTaskResponse = DescribeTaskResponse(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy