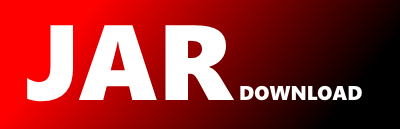
aws.sdk.kotlin.services.transcribe.model.CreateLanguageModelResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of transcribe Show documentation
Show all versions of transcribe Show documentation
Amazon Transcribe Service
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.transcribe.model
class CreateLanguageModelResponse private constructor(builder: Builder) {
/**
* The Amazon Transcribe standard language model, or base model, you used when creating your
* custom language model.
* If your audio has a sample rate of 16,000 Hz or greater, this value should be
* WideBand. If your audio has a sample rate of less than
* 16,000 Hz, this value should be NarrowBand.
*/
val baseModelName: aws.sdk.kotlin.services.transcribe.model.BaseModelName? = builder.baseModelName
/**
* Lists your data access role ARN (Amazon Resource Name) and the Amazon S3
* locations your provided for your training (S3Uri) and tuning
* (TuningDataS3Uri) data.
*/
val inputDataConfig: aws.sdk.kotlin.services.transcribe.model.InputDataConfig? = builder.inputDataConfig
/**
* The language code you selected for your custom language model.
*/
val languageCode: aws.sdk.kotlin.services.transcribe.model.ClmLanguageCode? = builder.languageCode
/**
* The unique name you chose for your custom language model.
*/
val modelName: kotlin.String? = builder.modelName
/**
* The status of your custom language model. When the status shows as
* COMPLETED, your model is ready to use.
*/
val modelStatus: aws.sdk.kotlin.services.transcribe.model.ModelStatus? = builder.modelStatus
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.transcribe.model.CreateLanguageModelResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateLanguageModelResponse(")
append("baseModelName=$baseModelName,")
append("inputDataConfig=$inputDataConfig,")
append("languageCode=$languageCode,")
append("modelName=$modelName,")
append("modelStatus=$modelStatus)")
}
override fun hashCode(): kotlin.Int {
var result = baseModelName?.hashCode() ?: 0
result = 31 * result + (inputDataConfig?.hashCode() ?: 0)
result = 31 * result + (languageCode?.hashCode() ?: 0)
result = 31 * result + (modelName?.hashCode() ?: 0)
result = 31 * result + (modelStatus?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateLanguageModelResponse
if (baseModelName != other.baseModelName) return false
if (inputDataConfig != other.inputDataConfig) return false
if (languageCode != other.languageCode) return false
if (modelName != other.modelName) return false
if (modelStatus != other.modelStatus) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.transcribe.model.CreateLanguageModelResponse = Builder(this).apply(block).build()
class Builder {
/**
* The Amazon Transcribe standard language model, or base model, you used when creating your
* custom language model.
* If your audio has a sample rate of 16,000 Hz or greater, this value should be
* WideBand. If your audio has a sample rate of less than
* 16,000 Hz, this value should be NarrowBand.
*/
var baseModelName: aws.sdk.kotlin.services.transcribe.model.BaseModelName? = null
/**
* Lists your data access role ARN (Amazon Resource Name) and the Amazon S3
* locations your provided for your training (S3Uri) and tuning
* (TuningDataS3Uri) data.
*/
var inputDataConfig: aws.sdk.kotlin.services.transcribe.model.InputDataConfig? = null
/**
* The language code you selected for your custom language model.
*/
var languageCode: aws.sdk.kotlin.services.transcribe.model.ClmLanguageCode? = null
/**
* The unique name you chose for your custom language model.
*/
var modelName: kotlin.String? = null
/**
* The status of your custom language model. When the status shows as
* COMPLETED, your model is ready to use.
*/
var modelStatus: aws.sdk.kotlin.services.transcribe.model.ModelStatus? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.transcribe.model.CreateLanguageModelResponse) : this() {
this.baseModelName = x.baseModelName
this.inputDataConfig = x.inputDataConfig
this.languageCode = x.languageCode
this.modelName = x.modelName
this.modelStatus = x.modelStatus
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.transcribe.model.CreateLanguageModelResponse = CreateLanguageModelResponse(this)
/**
* construct an [aws.sdk.kotlin.services.transcribe.model.InputDataConfig] inside the given [block]
*/
fun inputDataConfig(block: aws.sdk.kotlin.services.transcribe.model.InputDataConfig.Builder.() -> kotlin.Unit) {
this.inputDataConfig = aws.sdk.kotlin.services.transcribe.model.InputDataConfig.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy