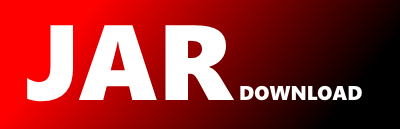
aws.sdk.kotlin.services.transcribe.model.MedicalTranscriptionJob.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of transcribe Show documentation
Show all versions of transcribe Show documentation
Amazon Transcribe Service
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.transcribe.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* The data structure that contains the information for a medical transcription job.
*/
class MedicalTranscriptionJob private constructor(builder: Builder) {
/**
* A timestamp that shows when the job was completed.
*/
val completionTime: aws.smithy.kotlin.runtime.time.Instant? = builder.completionTime
/**
* Shows the type of content that you've configured Amazon Transcribe Medical to identify in a transcription
* job. If the value is PHI, you've configured the job to identify personal health
* information (PHI) in the transcription output.
*/
val contentIdentificationType: aws.sdk.kotlin.services.transcribe.model.MedicalContentIdentificationType? = builder.contentIdentificationType
/**
* A timestamp that shows when the job was created.
*/
val creationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTime
/**
* If the TranscriptionJobStatus field is FAILED, this field
* contains information about why the job failed.
* The FailureReason field contains one of the following values:
*
*
*
* Unsupported media format- The media format specified in the
* MediaFormat field of the request isn't valid. See the description of the
* MediaFormat field for a list of valid values.
*
*
* The media format provided does not match the detected media
* format- The media format of the audio file doesn't match the format specified
* in the MediaFormat field in the request. Check the media format of
* your media file and make sure the two values match.
*
*
* Invalid sample rate for audio file- The sample rate specified in the
* MediaSampleRateHertz of the request isn't valid. The sample rate must
* be between 8,000 and 48,000 Hertz.
*
*
* The sample rate provided does not match the detected sample
* rate- The sample rate in the audio file doesn't match the sample rate specified
* in the MediaSampleRateHertz field in the request. Check the sample rate
* of your media file and make sure that the two values match.
*
*
* Invalid file size: file size too large- The size of your audio file is
* larger than what Amazon Transcribe Medical can process. For more information, see
* Guidelines
* and Quotas in the Amazon Transcribe Medical Guide.
*
*
* Invalid number of channels: number of channels too large- Your
* audio contains more channels than Amazon Transcribe Medical is configured to process. To request
* additional channels, see Amazon Transcribe Medical Endpoints and
* Quotas in the Amazon Web Services General
* Reference.
*/
val failureReason: kotlin.String? = builder.failureReason
/**
* The language code for the language spoken in the source audio file. US English (en-US)
* is the only supported language for medical transcriptions. Any other value you enter for
* language code results in a BadRequestException error.
*/
val languageCode: aws.sdk.kotlin.services.transcribe.model.LanguageCode? = builder.languageCode
/**
* Describes the input media file in a transcription request.
*/
val media: aws.sdk.kotlin.services.transcribe.model.Media? = builder.media
/**
* The format of the input media file.
*/
val mediaFormat: aws.sdk.kotlin.services.transcribe.model.MediaFormat? = builder.mediaFormat
/**
* The sample rate, in Hertz, of the source audio containing medical information.
* If you don't specify the sample rate, Amazon Transcribe Medical determines it for you. If you choose to
* specify the sample rate, it must match the rate detected by Amazon Transcribe Medical.
*/
val mediaSampleRateHertz: kotlin.Int? = builder.mediaSampleRateHertz
/**
* The name for a given medical transcription job.
*/
val medicalTranscriptionJobName: kotlin.String? = builder.medicalTranscriptionJobName
/**
* Object that contains object.
*/
val settings: aws.sdk.kotlin.services.transcribe.model.MedicalTranscriptionSetting? = builder.settings
/**
* The medical specialty of any clinicians providing a dictation or having a conversation.
* Refer to Transcribing a medical
* conversationfor a list of supported specialties.
*/
val specialty: aws.sdk.kotlin.services.transcribe.model.Specialty? = builder.specialty
/**
* A timestamp that shows when the job started processing.
*/
val startTime: aws.smithy.kotlin.runtime.time.Instant? = builder.startTime
/**
* A key:value pair assigned to a given medical transcription job.
*/
val tags: List? = builder.tags
/**
* An object that contains the MedicalTranscript. The
* MedicalTranscript contains the TranscriptFileUri.
*/
val transcript: aws.sdk.kotlin.services.transcribe.model.MedicalTranscript? = builder.transcript
/**
* The completion status of a medical transcription job.
*/
val transcriptionJobStatus: aws.sdk.kotlin.services.transcribe.model.TranscriptionJobStatus? = builder.transcriptionJobStatus
/**
* The type of speech in the transcription job. CONVERSATION is generally
* used for patient-physician dialogues. DICTATION is the setting for physicians
* speaking their notes after seeing a patient. For more information, see
* What
* is Amazon Transcribe Medical?.
*/
val type: aws.sdk.kotlin.services.transcribe.model.Type? = builder.type
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.transcribe.model.MedicalTranscriptionJob = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("MedicalTranscriptionJob(")
append("completionTime=$completionTime,")
append("contentIdentificationType=$contentIdentificationType,")
append("creationTime=$creationTime,")
append("failureReason=$failureReason,")
append("languageCode=$languageCode,")
append("media=$media,")
append("mediaFormat=$mediaFormat,")
append("mediaSampleRateHertz=$mediaSampleRateHertz,")
append("medicalTranscriptionJobName=$medicalTranscriptionJobName,")
append("settings=$settings,")
append("specialty=$specialty,")
append("startTime=$startTime,")
append("tags=$tags,")
append("transcript=$transcript,")
append("transcriptionJobStatus=$transcriptionJobStatus,")
append("type=$type)")
}
override fun hashCode(): kotlin.Int {
var result = completionTime?.hashCode() ?: 0
result = 31 * result + (contentIdentificationType?.hashCode() ?: 0)
result = 31 * result + (creationTime?.hashCode() ?: 0)
result = 31 * result + (failureReason?.hashCode() ?: 0)
result = 31 * result + (languageCode?.hashCode() ?: 0)
result = 31 * result + (media?.hashCode() ?: 0)
result = 31 * result + (mediaFormat?.hashCode() ?: 0)
result = 31 * result + (mediaSampleRateHertz ?: 0)
result = 31 * result + (medicalTranscriptionJobName?.hashCode() ?: 0)
result = 31 * result + (settings?.hashCode() ?: 0)
result = 31 * result + (specialty?.hashCode() ?: 0)
result = 31 * result + (startTime?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (transcript?.hashCode() ?: 0)
result = 31 * result + (transcriptionJobStatus?.hashCode() ?: 0)
result = 31 * result + (type?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as MedicalTranscriptionJob
if (completionTime != other.completionTime) return false
if (contentIdentificationType != other.contentIdentificationType) return false
if (creationTime != other.creationTime) return false
if (failureReason != other.failureReason) return false
if (languageCode != other.languageCode) return false
if (media != other.media) return false
if (mediaFormat != other.mediaFormat) return false
if (mediaSampleRateHertz != other.mediaSampleRateHertz) return false
if (medicalTranscriptionJobName != other.medicalTranscriptionJobName) return false
if (settings != other.settings) return false
if (specialty != other.specialty) return false
if (startTime != other.startTime) return false
if (tags != other.tags) return false
if (transcript != other.transcript) return false
if (transcriptionJobStatus != other.transcriptionJobStatus) return false
if (type != other.type) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.transcribe.model.MedicalTranscriptionJob = Builder(this).apply(block).build()
class Builder {
/**
* A timestamp that shows when the job was completed.
*/
var completionTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Shows the type of content that you've configured Amazon Transcribe Medical to identify in a transcription
* job. If the value is PHI, you've configured the job to identify personal health
* information (PHI) in the transcription output.
*/
var contentIdentificationType: aws.sdk.kotlin.services.transcribe.model.MedicalContentIdentificationType? = null
/**
* A timestamp that shows when the job was created.
*/
var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* If the TranscriptionJobStatus field is FAILED, this field
* contains information about why the job failed.
* The FailureReason field contains one of the following values:
*
*
*
* Unsupported media format- The media format specified in the
* MediaFormat field of the request isn't valid. See the description of the
* MediaFormat field for a list of valid values.
*
*
* The media format provided does not match the detected media
* format- The media format of the audio file doesn't match the format specified
* in the MediaFormat field in the request. Check the media format of
* your media file and make sure the two values match.
*
*
* Invalid sample rate for audio file- The sample rate specified in the
* MediaSampleRateHertz of the request isn't valid. The sample rate must
* be between 8,000 and 48,000 Hertz.
*
*
* The sample rate provided does not match the detected sample
* rate- The sample rate in the audio file doesn't match the sample rate specified
* in the MediaSampleRateHertz field in the request. Check the sample rate
* of your media file and make sure that the two values match.
*
*
* Invalid file size: file size too large- The size of your audio file is
* larger than what Amazon Transcribe Medical can process. For more information, see
* Guidelines
* and Quotas in the Amazon Transcribe Medical Guide.
*
*
* Invalid number of channels: number of channels too large- Your
* audio contains more channels than Amazon Transcribe Medical is configured to process. To request
* additional channels, see Amazon Transcribe Medical Endpoints and
* Quotas in the Amazon Web Services General
* Reference.
*/
var failureReason: kotlin.String? = null
/**
* The language code for the language spoken in the source audio file. US English (en-US)
* is the only supported language for medical transcriptions. Any other value you enter for
* language code results in a BadRequestException error.
*/
var languageCode: aws.sdk.kotlin.services.transcribe.model.LanguageCode? = null
/**
* Describes the input media file in a transcription request.
*/
var media: aws.sdk.kotlin.services.transcribe.model.Media? = null
/**
* The format of the input media file.
*/
var mediaFormat: aws.sdk.kotlin.services.transcribe.model.MediaFormat? = null
/**
* The sample rate, in Hertz, of the source audio containing medical information.
* If you don't specify the sample rate, Amazon Transcribe Medical determines it for you. If you choose to
* specify the sample rate, it must match the rate detected by Amazon Transcribe Medical.
*/
var mediaSampleRateHertz: kotlin.Int? = null
/**
* The name for a given medical transcription job.
*/
var medicalTranscriptionJobName: kotlin.String? = null
/**
* Object that contains object.
*/
var settings: aws.sdk.kotlin.services.transcribe.model.MedicalTranscriptionSetting? = null
/**
* The medical specialty of any clinicians providing a dictation or having a conversation.
* Refer to Transcribing a medical
* conversationfor a list of supported specialties.
*/
var specialty: aws.sdk.kotlin.services.transcribe.model.Specialty? = null
/**
* A timestamp that shows when the job started processing.
*/
var startTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* A key:value pair assigned to a given medical transcription job.
*/
var tags: List? = null
/**
* An object that contains the MedicalTranscript. The
* MedicalTranscript contains the TranscriptFileUri.
*/
var transcript: aws.sdk.kotlin.services.transcribe.model.MedicalTranscript? = null
/**
* The completion status of a medical transcription job.
*/
var transcriptionJobStatus: aws.sdk.kotlin.services.transcribe.model.TranscriptionJobStatus? = null
/**
* The type of speech in the transcription job. CONVERSATION is generally
* used for patient-physician dialogues. DICTATION is the setting for physicians
* speaking their notes after seeing a patient. For more information, see
* What
* is Amazon Transcribe Medical?.
*/
var type: aws.sdk.kotlin.services.transcribe.model.Type? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.transcribe.model.MedicalTranscriptionJob) : this() {
this.completionTime = x.completionTime
this.contentIdentificationType = x.contentIdentificationType
this.creationTime = x.creationTime
this.failureReason = x.failureReason
this.languageCode = x.languageCode
this.media = x.media
this.mediaFormat = x.mediaFormat
this.mediaSampleRateHertz = x.mediaSampleRateHertz
this.medicalTranscriptionJobName = x.medicalTranscriptionJobName
this.settings = x.settings
this.specialty = x.specialty
this.startTime = x.startTime
this.tags = x.tags
this.transcript = x.transcript
this.transcriptionJobStatus = x.transcriptionJobStatus
this.type = x.type
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.transcribe.model.MedicalTranscriptionJob = MedicalTranscriptionJob(this)
/**
* construct an [aws.sdk.kotlin.services.transcribe.model.Media] inside the given [block]
*/
fun media(block: aws.sdk.kotlin.services.transcribe.model.Media.Builder.() -> kotlin.Unit) {
this.media = aws.sdk.kotlin.services.transcribe.model.Media.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.transcribe.model.MedicalTranscriptionSetting] inside the given [block]
*/
fun settings(block: aws.sdk.kotlin.services.transcribe.model.MedicalTranscriptionSetting.Builder.() -> kotlin.Unit) {
this.settings = aws.sdk.kotlin.services.transcribe.model.MedicalTranscriptionSetting.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.transcribe.model.MedicalTranscript] inside the given [block]
*/
fun transcript(block: aws.sdk.kotlin.services.transcribe.model.MedicalTranscript.Builder.() -> kotlin.Unit) {
this.transcript = aws.sdk.kotlin.services.transcribe.model.MedicalTranscript.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy