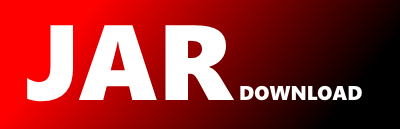
aws.sdk.kotlin.services.transcribe.model.MedicalTranscriptionJobSummary.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of transcribe Show documentation
Show all versions of transcribe Show documentation
Amazon Transcribe Service
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.transcribe.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* Provides summary information about a transcription job.
*/
class MedicalTranscriptionJobSummary private constructor(builder: Builder) {
/**
* A timestamp that shows when the job was completed.
*/
val completionTime: aws.smithy.kotlin.runtime.time.Instant? = builder.completionTime
/**
* Shows the type of information you've configured Amazon Transcribe Medical to identify in a transcription job.
* If the value is PHI, you've configured the transcription job to identify personal
* health information (PHI).
*/
val contentIdentificationType: aws.sdk.kotlin.services.transcribe.model.MedicalContentIdentificationType? = builder.contentIdentificationType
/**
* A timestamp that shows when the medical transcription job was created.
*/
val creationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTime
/**
* If the TranscriptionJobStatus field is FAILED, a description
* of the error.
*/
val failureReason: kotlin.String? = builder.failureReason
/**
* The language of the transcript in the source audio file.
*/
val languageCode: aws.sdk.kotlin.services.transcribe.model.LanguageCode? = builder.languageCode
/**
* The name of a medical transcription job.
*/
val medicalTranscriptionJobName: kotlin.String? = builder.medicalTranscriptionJobName
/**
* Indicates the location of the transcription job's output. This field must be the path of an
* S3 bucket; if you don't already have an S3 bucket, one is created based on the path you
* add.
*/
val outputLocationType: aws.sdk.kotlin.services.transcribe.model.OutputLocationType? = builder.outputLocationType
/**
* The medical specialty of the transcription job. Refer to Transcribing a medical
* conversationfor a list of supported specialties.
*/
val specialty: aws.sdk.kotlin.services.transcribe.model.Specialty? = builder.specialty
/**
* A timestamp that shows when the job began processing.
*/
val startTime: aws.smithy.kotlin.runtime.time.Instant? = builder.startTime
/**
* The status of the medical transcription job.
*/
val transcriptionJobStatus: aws.sdk.kotlin.services.transcribe.model.TranscriptionJobStatus? = builder.transcriptionJobStatus
/**
* The speech of the clinician in the input audio.
*/
val type: aws.sdk.kotlin.services.transcribe.model.Type? = builder.type
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.transcribe.model.MedicalTranscriptionJobSummary = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("MedicalTranscriptionJobSummary(")
append("completionTime=$completionTime,")
append("contentIdentificationType=$contentIdentificationType,")
append("creationTime=$creationTime,")
append("failureReason=$failureReason,")
append("languageCode=$languageCode,")
append("medicalTranscriptionJobName=$medicalTranscriptionJobName,")
append("outputLocationType=$outputLocationType,")
append("specialty=$specialty,")
append("startTime=$startTime,")
append("transcriptionJobStatus=$transcriptionJobStatus,")
append("type=$type)")
}
override fun hashCode(): kotlin.Int {
var result = completionTime?.hashCode() ?: 0
result = 31 * result + (contentIdentificationType?.hashCode() ?: 0)
result = 31 * result + (creationTime?.hashCode() ?: 0)
result = 31 * result + (failureReason?.hashCode() ?: 0)
result = 31 * result + (languageCode?.hashCode() ?: 0)
result = 31 * result + (medicalTranscriptionJobName?.hashCode() ?: 0)
result = 31 * result + (outputLocationType?.hashCode() ?: 0)
result = 31 * result + (specialty?.hashCode() ?: 0)
result = 31 * result + (startTime?.hashCode() ?: 0)
result = 31 * result + (transcriptionJobStatus?.hashCode() ?: 0)
result = 31 * result + (type?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as MedicalTranscriptionJobSummary
if (completionTime != other.completionTime) return false
if (contentIdentificationType != other.contentIdentificationType) return false
if (creationTime != other.creationTime) return false
if (failureReason != other.failureReason) return false
if (languageCode != other.languageCode) return false
if (medicalTranscriptionJobName != other.medicalTranscriptionJobName) return false
if (outputLocationType != other.outputLocationType) return false
if (specialty != other.specialty) return false
if (startTime != other.startTime) return false
if (transcriptionJobStatus != other.transcriptionJobStatus) return false
if (type != other.type) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.transcribe.model.MedicalTranscriptionJobSummary = Builder(this).apply(block).build()
class Builder {
/**
* A timestamp that shows when the job was completed.
*/
var completionTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Shows the type of information you've configured Amazon Transcribe Medical to identify in a transcription job.
* If the value is PHI, you've configured the transcription job to identify personal
* health information (PHI).
*/
var contentIdentificationType: aws.sdk.kotlin.services.transcribe.model.MedicalContentIdentificationType? = null
/**
* A timestamp that shows when the medical transcription job was created.
*/
var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* If the TranscriptionJobStatus field is FAILED, a description
* of the error.
*/
var failureReason: kotlin.String? = null
/**
* The language of the transcript in the source audio file.
*/
var languageCode: aws.sdk.kotlin.services.transcribe.model.LanguageCode? = null
/**
* The name of a medical transcription job.
*/
var medicalTranscriptionJobName: kotlin.String? = null
/**
* Indicates the location of the transcription job's output. This field must be the path of an
* S3 bucket; if you don't already have an S3 bucket, one is created based on the path you
* add.
*/
var outputLocationType: aws.sdk.kotlin.services.transcribe.model.OutputLocationType? = null
/**
* The medical specialty of the transcription job. Refer to Transcribing a medical
* conversationfor a list of supported specialties.
*/
var specialty: aws.sdk.kotlin.services.transcribe.model.Specialty? = null
/**
* A timestamp that shows when the job began processing.
*/
var startTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The status of the medical transcription job.
*/
var transcriptionJobStatus: aws.sdk.kotlin.services.transcribe.model.TranscriptionJobStatus? = null
/**
* The speech of the clinician in the input audio.
*/
var type: aws.sdk.kotlin.services.transcribe.model.Type? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.transcribe.model.MedicalTranscriptionJobSummary) : this() {
this.completionTime = x.completionTime
this.contentIdentificationType = x.contentIdentificationType
this.creationTime = x.creationTime
this.failureReason = x.failureReason
this.languageCode = x.languageCode
this.medicalTranscriptionJobName = x.medicalTranscriptionJobName
this.outputLocationType = x.outputLocationType
this.specialty = x.specialty
this.startTime = x.startTime
this.transcriptionJobStatus = x.transcriptionJobStatus
this.type = x.type
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.transcribe.model.MedicalTranscriptionJobSummary = MedicalTranscriptionJobSummary(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy