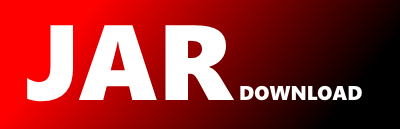
aws.sdk.kotlin.services.transcribe.model.UpdateVocabularyRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of transcribe Show documentation
Show all versions of transcribe Show documentation
Amazon Transcribe Service
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.transcribe.model
class UpdateVocabularyRequest private constructor(builder: Builder) {
/**
* The language code of the vocabulary entries. For a list of languages and their
* corresponding language codes, see Supported languages.
*/
val languageCode: aws.sdk.kotlin.services.transcribe.model.LanguageCode? = builder.languageCode
/**
* An array of strings containing the vocabulary entries.
*/
val phrases: List? = builder.phrases
/**
* The S3 location of the text file that contains the definition of the custom vocabulary.
* The URI must be in the same region as the API endpoint that you are calling. The general form
* is:
*
* https://s3.aws-region.amazonaws.com/bucket-name/keyprefix/objectkey
*
* For example:
*
* https://s3.us-east-1.amazonaws.com/DOC-EXAMPLE-BUCKET/vocab.txt
*
* For more information about S3 object names, see Object Keys in the
* Amazon S3 Developer Guide.
* For more information about custom vocabularies, see Custom
* Vocabularies.
*/
val vocabularyFileUri: kotlin.String? = builder.vocabularyFileUri
/**
* The name of the vocabulary to update. The name is case sensitive. If you try to update
* a vocabulary with the same name as a previous vocabulary you will receive a
* ConflictException error.
*/
val vocabularyName: kotlin.String? = builder.vocabularyName
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.transcribe.model.UpdateVocabularyRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("UpdateVocabularyRequest(")
append("languageCode=$languageCode,")
append("phrases=$phrases,")
append("vocabularyFileUri=$vocabularyFileUri,")
append("vocabularyName=$vocabularyName)")
}
override fun hashCode(): kotlin.Int {
var result = languageCode?.hashCode() ?: 0
result = 31 * result + (phrases?.hashCode() ?: 0)
result = 31 * result + (vocabularyFileUri?.hashCode() ?: 0)
result = 31 * result + (vocabularyName?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as UpdateVocabularyRequest
if (languageCode != other.languageCode) return false
if (phrases != other.phrases) return false
if (vocabularyFileUri != other.vocabularyFileUri) return false
if (vocabularyName != other.vocabularyName) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.transcribe.model.UpdateVocabularyRequest = Builder(this).apply(block).build()
class Builder {
/**
* The language code of the vocabulary entries. For a list of languages and their
* corresponding language codes, see Supported languages.
*/
var languageCode: aws.sdk.kotlin.services.transcribe.model.LanguageCode? = null
/**
* An array of strings containing the vocabulary entries.
*/
var phrases: List? = null
/**
* The S3 location of the text file that contains the definition of the custom vocabulary.
* The URI must be in the same region as the API endpoint that you are calling. The general form
* is:
*
* https://s3.aws-region.amazonaws.com/bucket-name/keyprefix/objectkey
*
* For example:
*
* https://s3.us-east-1.amazonaws.com/DOC-EXAMPLE-BUCKET/vocab.txt
*
* For more information about S3 object names, see Object Keys in the
* Amazon S3 Developer Guide.
* For more information about custom vocabularies, see Custom
* Vocabularies.
*/
var vocabularyFileUri: kotlin.String? = null
/**
* The name of the vocabulary to update. The name is case sensitive. If you try to update
* a vocabulary with the same name as a previous vocabulary you will receive a
* ConflictException error.
*/
var vocabularyName: kotlin.String? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.transcribe.model.UpdateVocabularyRequest) : this() {
this.languageCode = x.languageCode
this.phrases = x.phrases
this.vocabularyFileUri = x.vocabularyFileUri
this.vocabularyName = x.vocabularyName
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.transcribe.model.UpdateVocabularyRequest = UpdateVocabularyRequest(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy