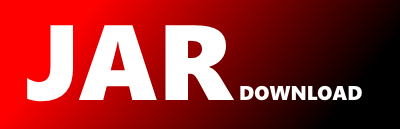
commonMain.aws.sdk.kotlin.services.transcribe.model.RelativeTimeRange.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of transcribe Show documentation
Show all versions of transcribe Show documentation
Amazon Transcribe Service
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.transcribe.model
/**
* A time range, in percentage, between two points in your media file.
*
* You can use `StartPercentage` and `EndPercentage` to search a custom segment. For example, setting `StartPercentage` to 10 and `EndPercentage` to 50 only searches for your specified criteria in the audio contained between the 10 percent mark and the 50 percent mark of your media file.
*
* You can use also `First` to search from the start of the media file until the time you specify, or `Last` to search from the time you specify until the end of the media file. For example, setting `First` to 10 only searches for your specified criteria in the audio contained in the first 10 percent of the media file.
*
* If you prefer to use milliseconds instead of percentage, see .
*/
public class RelativeTimeRange private constructor(builder: Builder) {
/**
* The time, in percentage, when Amazon Transcribe stops searching for the specified criteria in your media file. If you include `EndPercentage` in your request, you must also include `StartPercentage`.
*/
public val endPercentage: kotlin.Int? = builder.endPercentage
/**
* The time, in percentage, from the start of your media file until the value you specify in which Amazon Transcribe searches for your specified criteria.
*/
public val first: kotlin.Int? = builder.first
/**
* The time, in percentage, from the value you specify until the end of your media file in which Amazon Transcribe searches for your specified criteria.
*/
public val last: kotlin.Int? = builder.last
/**
* The time, in percentage, when Amazon Transcribe starts searching for the specified criteria in your media file. If you include `StartPercentage` in your request, you must also include `EndPercentage`.
*/
public val startPercentage: kotlin.Int? = builder.startPercentage
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.transcribe.model.RelativeTimeRange = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("RelativeTimeRange(")
append("endPercentage=$endPercentage,")
append("first=$first,")
append("last=$last,")
append("startPercentage=$startPercentage)")
}
override fun hashCode(): kotlin.Int {
var result = endPercentage ?: 0
result = 31 * result + (first ?: 0)
result = 31 * result + (last ?: 0)
result = 31 * result + (startPercentage ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as RelativeTimeRange
if (endPercentage != other.endPercentage) return false
if (first != other.first) return false
if (last != other.last) return false
if (startPercentage != other.startPercentage) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.transcribe.model.RelativeTimeRange = Builder(this).apply(block).build()
public class Builder {
/**
* The time, in percentage, when Amazon Transcribe stops searching for the specified criteria in your media file. If you include `EndPercentage` in your request, you must also include `StartPercentage`.
*/
public var endPercentage: kotlin.Int? = null
/**
* The time, in percentage, from the start of your media file until the value you specify in which Amazon Transcribe searches for your specified criteria.
*/
public var first: kotlin.Int? = null
/**
* The time, in percentage, from the value you specify until the end of your media file in which Amazon Transcribe searches for your specified criteria.
*/
public var last: kotlin.Int? = null
/**
* The time, in percentage, when Amazon Transcribe starts searching for the specified criteria in your media file. If you include `StartPercentage` in your request, you must also include `EndPercentage`.
*/
public var startPercentage: kotlin.Int? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.transcribe.model.RelativeTimeRange) : this() {
this.endPercentage = x.endPercentage
this.first = x.first
this.last = x.last
this.startPercentage = x.startPercentage
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.transcribe.model.RelativeTimeRange = RelativeTimeRange(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy