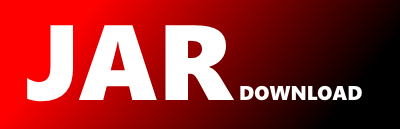
commonMain.aws.sdk.kotlin.services.transcribe.model.MedicalTranscriptionJobSummary.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of transcribe Show documentation
Show all versions of transcribe Show documentation
Amazon Transcribe Service
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.transcribe.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* Provides detailed information about a specific medical transcription job.
*/
public class MedicalTranscriptionJobSummary private constructor(builder: Builder) {
/**
* The date and time the specified medical transcription job finished processing.
*
* Timestamps are in the format `YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC`. For example, `2022-05-04T12:33:13.922000-07:00` represents a transcription job that started processing at 12:33 PM UTC-7 on May 4, 2022.
*/
public val completionTime: aws.smithy.kotlin.runtime.time.Instant? = builder.completionTime
/**
* Labels all personal health information (PHI) identified in your transcript. For more information, see [Identifying personal health information (PHI) in a transcription](https://docs.aws.amazon.com/transcribe/latest/dg/phi-id.html).
*/
public val contentIdentificationType: aws.sdk.kotlin.services.transcribe.model.MedicalContentIdentificationType? = builder.contentIdentificationType
/**
* The date and time the specified medical transcription job request was made.
*
* Timestamps are in the format `YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC`. For example, `2022-05-04T12:32:58.761000-07:00` represents a transcription job that started processing at 12:32 PM UTC-7 on May 4, 2022.
*/
public val creationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTime
/**
* If `TranscriptionJobStatus` is `FAILED`, `FailureReason` contains information about why the transcription job failed. See also: [Common Errors](https://docs.aws.amazon.com/transcribe/latest/APIReference/CommonErrors.html).
*/
public val failureReason: kotlin.String? = builder.failureReason
/**
* The language code used to create your medical transcription. US English (`en-US`) is the only supported language for medical transcriptions.
*/
public val languageCode: aws.sdk.kotlin.services.transcribe.model.LanguageCode? = builder.languageCode
/**
* The name of the medical transcription job. Job names are case sensitive and must be unique within an Amazon Web Services account.
*/
public val medicalTranscriptionJobName: kotlin.String? = builder.medicalTranscriptionJobName
/**
* Indicates where the specified medical transcription output is stored.
*
* If the value is `CUSTOMER_BUCKET`, the location is the Amazon S3 bucket you specified using the `OutputBucketName` parameter in your request. If you also included `OutputKey` in your request, your output is located in the path you specified in your request.
*
* If the value is `SERVICE_BUCKET`, the location is a service-managed Amazon S3 bucket. To access a transcript stored in a service-managed bucket, use the URI shown in the `TranscriptFileUri` field.
*/
public val outputLocationType: aws.sdk.kotlin.services.transcribe.model.OutputLocationType? = builder.outputLocationType
/**
* Provides the medical specialty represented in your media.
*/
public val specialty: aws.sdk.kotlin.services.transcribe.model.Specialty? = builder.specialty
/**
* The date and time your medical transcription job began processing.
*
* Timestamps are in the format `YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC`. For example, `2022-05-04T12:32:58.789000-07:00` represents a transcription job that started processing at 12:32 PM UTC-7 on May 4, 2022.
*/
public val startTime: aws.smithy.kotlin.runtime.time.Instant? = builder.startTime
/**
* Provides the status of your medical transcription job.
*
* If the status is `COMPLETED`, the job is finished and you can find the results at the location specified in `TranscriptFileUri`. If the status is `FAILED`, `FailureReason` provides details on why your transcription job failed.
*/
public val transcriptionJobStatus: aws.sdk.kotlin.services.transcribe.model.TranscriptionJobStatus? = builder.transcriptionJobStatus
/**
* Indicates whether the input media is a dictation or a conversation, as specified in the `StartMedicalTranscriptionJob` request.
*/
public val type: aws.sdk.kotlin.services.transcribe.model.Type? = builder.type
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.transcribe.model.MedicalTranscriptionJobSummary = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("MedicalTranscriptionJobSummary(")
append("completionTime=$completionTime,")
append("contentIdentificationType=$contentIdentificationType,")
append("creationTime=$creationTime,")
append("failureReason=$failureReason,")
append("languageCode=$languageCode,")
append("medicalTranscriptionJobName=$medicalTranscriptionJobName,")
append("outputLocationType=$outputLocationType,")
append("specialty=$specialty,")
append("startTime=$startTime,")
append("transcriptionJobStatus=$transcriptionJobStatus,")
append("type=$type)")
}
override fun hashCode(): kotlin.Int {
var result = completionTime?.hashCode() ?: 0
result = 31 * result + (contentIdentificationType?.hashCode() ?: 0)
result = 31 * result + (creationTime?.hashCode() ?: 0)
result = 31 * result + (failureReason?.hashCode() ?: 0)
result = 31 * result + (languageCode?.hashCode() ?: 0)
result = 31 * result + (medicalTranscriptionJobName?.hashCode() ?: 0)
result = 31 * result + (outputLocationType?.hashCode() ?: 0)
result = 31 * result + (specialty?.hashCode() ?: 0)
result = 31 * result + (startTime?.hashCode() ?: 0)
result = 31 * result + (transcriptionJobStatus?.hashCode() ?: 0)
result = 31 * result + (type?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as MedicalTranscriptionJobSummary
if (completionTime != other.completionTime) return false
if (contentIdentificationType != other.contentIdentificationType) return false
if (creationTime != other.creationTime) return false
if (failureReason != other.failureReason) return false
if (languageCode != other.languageCode) return false
if (medicalTranscriptionJobName != other.medicalTranscriptionJobName) return false
if (outputLocationType != other.outputLocationType) return false
if (specialty != other.specialty) return false
if (startTime != other.startTime) return false
if (transcriptionJobStatus != other.transcriptionJobStatus) return false
if (type != other.type) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.transcribe.model.MedicalTranscriptionJobSummary = Builder(this).apply(block).build()
public class Builder {
/**
* The date and time the specified medical transcription job finished processing.
*
* Timestamps are in the format `YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC`. For example, `2022-05-04T12:33:13.922000-07:00` represents a transcription job that started processing at 12:33 PM UTC-7 on May 4, 2022.
*/
public var completionTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Labels all personal health information (PHI) identified in your transcript. For more information, see [Identifying personal health information (PHI) in a transcription](https://docs.aws.amazon.com/transcribe/latest/dg/phi-id.html).
*/
public var contentIdentificationType: aws.sdk.kotlin.services.transcribe.model.MedicalContentIdentificationType? = null
/**
* The date and time the specified medical transcription job request was made.
*
* Timestamps are in the format `YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC`. For example, `2022-05-04T12:32:58.761000-07:00` represents a transcription job that started processing at 12:32 PM UTC-7 on May 4, 2022.
*/
public var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* If `TranscriptionJobStatus` is `FAILED`, `FailureReason` contains information about why the transcription job failed. See also: [Common Errors](https://docs.aws.amazon.com/transcribe/latest/APIReference/CommonErrors.html).
*/
public var failureReason: kotlin.String? = null
/**
* The language code used to create your medical transcription. US English (`en-US`) is the only supported language for medical transcriptions.
*/
public var languageCode: aws.sdk.kotlin.services.transcribe.model.LanguageCode? = null
/**
* The name of the medical transcription job. Job names are case sensitive and must be unique within an Amazon Web Services account.
*/
public var medicalTranscriptionJobName: kotlin.String? = null
/**
* Indicates where the specified medical transcription output is stored.
*
* If the value is `CUSTOMER_BUCKET`, the location is the Amazon S3 bucket you specified using the `OutputBucketName` parameter in your request. If you also included `OutputKey` in your request, your output is located in the path you specified in your request.
*
* If the value is `SERVICE_BUCKET`, the location is a service-managed Amazon S3 bucket. To access a transcript stored in a service-managed bucket, use the URI shown in the `TranscriptFileUri` field.
*/
public var outputLocationType: aws.sdk.kotlin.services.transcribe.model.OutputLocationType? = null
/**
* Provides the medical specialty represented in your media.
*/
public var specialty: aws.sdk.kotlin.services.transcribe.model.Specialty? = null
/**
* The date and time your medical transcription job began processing.
*
* Timestamps are in the format `YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC`. For example, `2022-05-04T12:32:58.789000-07:00` represents a transcription job that started processing at 12:32 PM UTC-7 on May 4, 2022.
*/
public var startTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Provides the status of your medical transcription job.
*
* If the status is `COMPLETED`, the job is finished and you can find the results at the location specified in `TranscriptFileUri`. If the status is `FAILED`, `FailureReason` provides details on why your transcription job failed.
*/
public var transcriptionJobStatus: aws.sdk.kotlin.services.transcribe.model.TranscriptionJobStatus? = null
/**
* Indicates whether the input media is a dictation or a conversation, as specified in the `StartMedicalTranscriptionJob` request.
*/
public var type: aws.sdk.kotlin.services.transcribe.model.Type? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.transcribe.model.MedicalTranscriptionJobSummary) : this() {
this.completionTime = x.completionTime
this.contentIdentificationType = x.contentIdentificationType
this.creationTime = x.creationTime
this.failureReason = x.failureReason
this.languageCode = x.languageCode
this.medicalTranscriptionJobName = x.medicalTranscriptionJobName
this.outputLocationType = x.outputLocationType
this.specialty = x.specialty
this.startTime = x.startTime
this.transcriptionJobStatus = x.transcriptionJobStatus
this.type = x.type
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.transcribe.model.MedicalTranscriptionJobSummary = MedicalTranscriptionJobSummary(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy