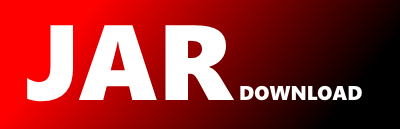
commonMain.aws.sdk.kotlin.services.transcribe.model.TranscriptionJob.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.transcribe.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* Provides detailed information about a transcription job.
*
* To view the status of the specified transcription job, check the `TranscriptionJobStatus` field. If the status is `COMPLETED`, the job is finished and you can find the results at the location specified in `TranscriptFileUri`. If the status is `FAILED`, `FailureReason` provides details on why your transcription job failed.
*
* If you enabled content redaction, the redacted transcript can be found at the location specified in `RedactedTranscriptFileUri`.
*/
public class TranscriptionJob private constructor(builder: Builder) {
/**
* The date and time the specified transcription job finished processing.
*
* Timestamps are in the format `YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC`. For example, `2022-05-04T12:33:13.922000-07:00` represents a transcription job that started processing at 12:33 PM UTC-7 on May 4, 2022.
*/
public val completionTime: aws.smithy.kotlin.runtime.time.Instant? = builder.completionTime
/**
* Redacts or flags specified personally identifiable information (PII) in your transcript.
*/
public val contentRedaction: aws.sdk.kotlin.services.transcribe.model.ContentRedaction? = builder.contentRedaction
/**
* The date and time the specified transcription job request was made.
*
* Timestamps are in the format `YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC`. For example, `2022-05-04T12:32:58.761000-07:00` represents a transcription job that started processing at 12:32 PM UTC-7 on May 4, 2022.
*/
public val creationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTime
/**
* If `TranscriptionJobStatus` is `FAILED`, `FailureReason` contains information about why the transcription job request failed.
*
* The `FailureReason` field contains one of the following values:
* + `Unsupported media format`.The media format specified in `MediaFormat` isn't valid. Refer to **MediaFormat** for a list of supported formats.
* + `The media format provided does not match the detected media format`.The media format specified in `MediaFormat` doesn't match the format of the input file. Check the media format of your media file and correct the specified value.
* + `Invalid sample rate for audio file`.The sample rate specified in `MediaSampleRateHertz` isn't valid. The sample rate must be between 8,000 and 48,000 Hertz.
* + `The sample rate provided does not match the detected sample rate`.The sample rate specified in `MediaSampleRateHertz` doesn't match the sample rate detected in your input media file. Check the sample rate of your media file and correct the specified value.
* + `Invalid file size: file size too large`.The size of your media file is larger than what Amazon Transcribe can process. For more information, refer to [Guidelines and quotas](https://docs.aws.amazon.com/transcribe/latest/dg/limits-guidelines.html#limits).
* + `Invalid number of channels: number of channels too large`.Your audio contains more channels than Amazon Transcribe is able to process. For more information, refer to [Guidelines and quotas](https://docs.aws.amazon.com/transcribe/latest/dg/limits-guidelines.html#limits).
*/
public val failureReason: kotlin.String? = builder.failureReason
/**
* The confidence score associated with the language identified in your media file.
*
* Confidence scores are values between 0 and 1; a larger value indicates a higher probability that the identified language correctly matches the language spoken in your media.
*/
public val identifiedLanguageScore: kotlin.Float? = builder.identifiedLanguageScore
/**
* Indicates whether automatic language identification was enabled (`TRUE`) for the specified transcription job.
*/
public val identifyLanguage: kotlin.Boolean? = builder.identifyLanguage
/**
* Indicates whether automatic multi-language identification was enabled (`TRUE`) for the specified transcription job.
*/
public val identifyMultipleLanguages: kotlin.Boolean? = builder.identifyMultipleLanguages
/**
* Provides information about how your transcription job is being processed. This parameter shows if your request is queued and what data access role is being used.
*/
public val jobExecutionSettings: aws.sdk.kotlin.services.transcribe.model.JobExecutionSettings? = builder.jobExecutionSettings
/**
* The language code used to create your transcription job. For a list of supported languages and their associated language codes, refer to the [Supported languages](https://docs.aws.amazon.com/transcribe/latest/dg/supported-languages.html) table.
*
* Note that you must include one of `LanguageCode`, `IdentifyLanguage`, or `IdentifyMultipleLanguages` in your request. If you include more than one of these parameters, your transcription job fails.
*/
public val languageCode: aws.sdk.kotlin.services.transcribe.model.LanguageCode? = builder.languageCode
/**
* The language codes used to create your transcription job. This parameter is used with multi-language identification. For single-language identification requests, refer to the singular version of this parameter, `LanguageCode`.
*
* For a list of supported languages and their associated language codes, refer to the [Supported languages](https://docs.aws.amazon.com/transcribe/latest/dg/supported-languages.html) table.
*/
public val languageCodes: List? = builder.languageCodes
/**
* If using automatic language identification (`IdentifyLanguage`) in your request and you want to apply a custom language model, a custom vocabulary, or a custom vocabulary filter, include `LanguageIdSettings` with the relevant sub-parameters (`VocabularyName`, `LanguageModelName`, and `VocabularyFilterName`).
*
* You can specify two or more language codes that represent the languages you think may be present in your media; including more than five is not recommended. Each language code you include can have an associated custom language model, custom vocabulary, and custom vocabulary filter. The languages you specify must match the languages of the specified custom language models, custom vocabularies, and custom vocabulary filters.
*
* To include language options using `IdentifyLanguage`**without** including a custom language model, a custom vocabulary, or a custom vocabulary filter, use `LanguageOptions` instead of `LanguageIdSettings`. Including language options can improve the accuracy of automatic language identification.
*
* If you want to include a custom language model with your request but **do not** want to use automatic language identification, use instead the `` parameter with the `LanguageModelName` sub-parameter.
*
* If you want to include a custom vocabulary or a custom vocabulary filter (or both) with your request but **do not** want to use automatic language identification, use instead the `` parameter with the `VocabularyName` or `VocabularyFilterName` (or both) sub-parameter.
*/
public val languageIdSettings: Map? = builder.languageIdSettings
/**
* You can specify two or more language codes that represent the languages you think may be present in your media; including more than five is not recommended. If you're unsure what languages are present, do not include this parameter.
*
* If you include `LanguageOptions` in your request, you must also include `IdentifyLanguage`.
*
* For more information, refer to [Supported languages](https://docs.aws.amazon.com/transcribe/latest/dg/supported-languages.html).
*
* To transcribe speech in Modern Standard Arabic (`ar-SA`), your media file must be encoded at a sample rate of 16,000 Hz or higher.
*/
public val languageOptions: List? = builder.languageOptions
/**
* Describes the Amazon S3 location of the media file you want to use in your request.
*/
public val media: aws.sdk.kotlin.services.transcribe.model.Media? = builder.media
/**
* The format of the input media file.
*/
public val mediaFormat: aws.sdk.kotlin.services.transcribe.model.MediaFormat? = builder.mediaFormat
/**
* The sample rate, in Hertz, of the audio track in your input media file.
*/
public val mediaSampleRateHertz: kotlin.Int? = builder.mediaSampleRateHertz
/**
* The custom language model you want to include with your transcription job. If you include `ModelSettings` in your request, you must include the `LanguageModelName` sub-parameter.
*/
public val modelSettings: aws.sdk.kotlin.services.transcribe.model.ModelSettings? = builder.modelSettings
/**
* Specify additional optional settings in your request, including channel identification, alternative transcriptions, speaker labeling; allows you to apply custom vocabularies and vocabulary filters.
*
* If you want to include a custom vocabulary or a custom vocabulary filter (or both) with your request but **do not** want to use automatic language identification, use `Settings` with the `VocabularyName` or `VocabularyFilterName` (or both) sub-parameter.
*
* If you're using automatic language identification with your request and want to include a custom language model, a custom vocabulary, or a custom vocabulary filter, do not use the `Settings` parameter; use instead the `` parameter with the `LanguageModelName`, `VocabularyName` or `VocabularyFilterName` sub-parameters.
*/
public val settings: aws.sdk.kotlin.services.transcribe.model.Settings? = builder.settings
/**
* The date and time the specified transcription job began processing.
*
* Timestamps are in the format `YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC`. For example, `2022-05-04T12:32:58.789000-07:00` represents a transcription job that started processing at 12:32 PM UTC-7 on May 4, 2022.
*/
public val startTime: aws.smithy.kotlin.runtime.time.Instant? = builder.startTime
/**
* Generate subtitles for your media file with your transcription request.
*/
public val subtitles: aws.sdk.kotlin.services.transcribe.model.SubtitlesOutput? = builder.subtitles
/**
* Adds one or more custom tags, each in the form of a key:value pair, to a new transcription job at the time you start this new job.
*
* To learn more about using tags with Amazon Transcribe, refer to [Tagging resources](https://docs.aws.amazon.com/transcribe/latest/dg/tagging.html).
*/
public val tags: List? = builder.tags
/**
* Provides you with the Amazon S3 URI you can use to access your transcript.
*/
public val transcript: aws.sdk.kotlin.services.transcribe.model.Transcript? = builder.transcript
/**
* The name of the transcription job. Job names are case sensitive and must be unique within an Amazon Web Services account.
*/
public val transcriptionJobName: kotlin.String? = builder.transcriptionJobName
/**
* Provides the status of the specified transcription job.
*
* If the status is `COMPLETED`, the job is finished and you can find the results at the location specified in `TranscriptFileUri` (or `RedactedTranscriptFileUri`, if you requested transcript redaction). If the status is `FAILED`, `FailureReason` provides details on why your transcription job failed.
*/
public val transcriptionJobStatus: aws.sdk.kotlin.services.transcribe.model.TranscriptionJobStatus? = builder.transcriptionJobStatus
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.transcribe.model.TranscriptionJob = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("TranscriptionJob(")
append("completionTime=$completionTime,")
append("contentRedaction=$contentRedaction,")
append("creationTime=$creationTime,")
append("failureReason=$failureReason,")
append("identifiedLanguageScore=$identifiedLanguageScore,")
append("identifyLanguage=$identifyLanguage,")
append("identifyMultipleLanguages=$identifyMultipleLanguages,")
append("jobExecutionSettings=$jobExecutionSettings,")
append("languageCode=$languageCode,")
append("languageCodes=$languageCodes,")
append("languageIdSettings=$languageIdSettings,")
append("languageOptions=$languageOptions,")
append("media=$media,")
append("mediaFormat=$mediaFormat,")
append("mediaSampleRateHertz=$mediaSampleRateHertz,")
append("modelSettings=$modelSettings,")
append("settings=$settings,")
append("startTime=$startTime,")
append("subtitles=$subtitles,")
append("tags=$tags,")
append("transcript=$transcript,")
append("transcriptionJobName=$transcriptionJobName,")
append("transcriptionJobStatus=$transcriptionJobStatus)")
}
override fun hashCode(): kotlin.Int {
var result = completionTime?.hashCode() ?: 0
result = 31 * result + (contentRedaction?.hashCode() ?: 0)
result = 31 * result + (creationTime?.hashCode() ?: 0)
result = 31 * result + (failureReason?.hashCode() ?: 0)
result = 31 * result + (identifiedLanguageScore?.hashCode() ?: 0)
result = 31 * result + (identifyLanguage?.hashCode() ?: 0)
result = 31 * result + (identifyMultipleLanguages?.hashCode() ?: 0)
result = 31 * result + (jobExecutionSettings?.hashCode() ?: 0)
result = 31 * result + (languageCode?.hashCode() ?: 0)
result = 31 * result + (languageCodes?.hashCode() ?: 0)
result = 31 * result + (languageIdSettings?.hashCode() ?: 0)
result = 31 * result + (languageOptions?.hashCode() ?: 0)
result = 31 * result + (media?.hashCode() ?: 0)
result = 31 * result + (mediaFormat?.hashCode() ?: 0)
result = 31 * result + (mediaSampleRateHertz ?: 0)
result = 31 * result + (modelSettings?.hashCode() ?: 0)
result = 31 * result + (settings?.hashCode() ?: 0)
result = 31 * result + (startTime?.hashCode() ?: 0)
result = 31 * result + (subtitles?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (transcript?.hashCode() ?: 0)
result = 31 * result + (transcriptionJobName?.hashCode() ?: 0)
result = 31 * result + (transcriptionJobStatus?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as TranscriptionJob
if (completionTime != other.completionTime) return false
if (contentRedaction != other.contentRedaction) return false
if (creationTime != other.creationTime) return false
if (failureReason != other.failureReason) return false
if (identifiedLanguageScore != other.identifiedLanguageScore) return false
if (identifyLanguage != other.identifyLanguage) return false
if (identifyMultipleLanguages != other.identifyMultipleLanguages) return false
if (jobExecutionSettings != other.jobExecutionSettings) return false
if (languageCode != other.languageCode) return false
if (languageCodes != other.languageCodes) return false
if (languageIdSettings != other.languageIdSettings) return false
if (languageOptions != other.languageOptions) return false
if (media != other.media) return false
if (mediaFormat != other.mediaFormat) return false
if (mediaSampleRateHertz != other.mediaSampleRateHertz) return false
if (modelSettings != other.modelSettings) return false
if (settings != other.settings) return false
if (startTime != other.startTime) return false
if (subtitles != other.subtitles) return false
if (tags != other.tags) return false
if (transcript != other.transcript) return false
if (transcriptionJobName != other.transcriptionJobName) return false
if (transcriptionJobStatus != other.transcriptionJobStatus) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.transcribe.model.TranscriptionJob = Builder(this).apply(block).build()
public class Builder {
/**
* The date and time the specified transcription job finished processing.
*
* Timestamps are in the format `YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC`. For example, `2022-05-04T12:33:13.922000-07:00` represents a transcription job that started processing at 12:33 PM UTC-7 on May 4, 2022.
*/
public var completionTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Redacts or flags specified personally identifiable information (PII) in your transcript.
*/
public var contentRedaction: aws.sdk.kotlin.services.transcribe.model.ContentRedaction? = null
/**
* The date and time the specified transcription job request was made.
*
* Timestamps are in the format `YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC`. For example, `2022-05-04T12:32:58.761000-07:00` represents a transcription job that started processing at 12:32 PM UTC-7 on May 4, 2022.
*/
public var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* If `TranscriptionJobStatus` is `FAILED`, `FailureReason` contains information about why the transcription job request failed.
*
* The `FailureReason` field contains one of the following values:
* + `Unsupported media format`.The media format specified in `MediaFormat` isn't valid. Refer to **MediaFormat** for a list of supported formats.
* + `The media format provided does not match the detected media format`.The media format specified in `MediaFormat` doesn't match the format of the input file. Check the media format of your media file and correct the specified value.
* + `Invalid sample rate for audio file`.The sample rate specified in `MediaSampleRateHertz` isn't valid. The sample rate must be between 8,000 and 48,000 Hertz.
* + `The sample rate provided does not match the detected sample rate`.The sample rate specified in `MediaSampleRateHertz` doesn't match the sample rate detected in your input media file. Check the sample rate of your media file and correct the specified value.
* + `Invalid file size: file size too large`.The size of your media file is larger than what Amazon Transcribe can process. For more information, refer to [Guidelines and quotas](https://docs.aws.amazon.com/transcribe/latest/dg/limits-guidelines.html#limits).
* + `Invalid number of channels: number of channels too large`.Your audio contains more channels than Amazon Transcribe is able to process. For more information, refer to [Guidelines and quotas](https://docs.aws.amazon.com/transcribe/latest/dg/limits-guidelines.html#limits).
*/
public var failureReason: kotlin.String? = null
/**
* The confidence score associated with the language identified in your media file.
*
* Confidence scores are values between 0 and 1; a larger value indicates a higher probability that the identified language correctly matches the language spoken in your media.
*/
public var identifiedLanguageScore: kotlin.Float? = null
/**
* Indicates whether automatic language identification was enabled (`TRUE`) for the specified transcription job.
*/
public var identifyLanguage: kotlin.Boolean? = null
/**
* Indicates whether automatic multi-language identification was enabled (`TRUE`) for the specified transcription job.
*/
public var identifyMultipleLanguages: kotlin.Boolean? = null
/**
* Provides information about how your transcription job is being processed. This parameter shows if your request is queued and what data access role is being used.
*/
public var jobExecutionSettings: aws.sdk.kotlin.services.transcribe.model.JobExecutionSettings? = null
/**
* The language code used to create your transcription job. For a list of supported languages and their associated language codes, refer to the [Supported languages](https://docs.aws.amazon.com/transcribe/latest/dg/supported-languages.html) table.
*
* Note that you must include one of `LanguageCode`, `IdentifyLanguage`, or `IdentifyMultipleLanguages` in your request. If you include more than one of these parameters, your transcription job fails.
*/
public var languageCode: aws.sdk.kotlin.services.transcribe.model.LanguageCode? = null
/**
* The language codes used to create your transcription job. This parameter is used with multi-language identification. For single-language identification requests, refer to the singular version of this parameter, `LanguageCode`.
*
* For a list of supported languages and their associated language codes, refer to the [Supported languages](https://docs.aws.amazon.com/transcribe/latest/dg/supported-languages.html) table.
*/
public var languageCodes: List? = null
/**
* If using automatic language identification (`IdentifyLanguage`) in your request and you want to apply a custom language model, a custom vocabulary, or a custom vocabulary filter, include `LanguageIdSettings` with the relevant sub-parameters (`VocabularyName`, `LanguageModelName`, and `VocabularyFilterName`).
*
* You can specify two or more language codes that represent the languages you think may be present in your media; including more than five is not recommended. Each language code you include can have an associated custom language model, custom vocabulary, and custom vocabulary filter. The languages you specify must match the languages of the specified custom language models, custom vocabularies, and custom vocabulary filters.
*
* To include language options using `IdentifyLanguage`**without** including a custom language model, a custom vocabulary, or a custom vocabulary filter, use `LanguageOptions` instead of `LanguageIdSettings`. Including language options can improve the accuracy of automatic language identification.
*
* If you want to include a custom language model with your request but **do not** want to use automatic language identification, use instead the `` parameter with the `LanguageModelName` sub-parameter.
*
* If you want to include a custom vocabulary or a custom vocabulary filter (or both) with your request but **do not** want to use automatic language identification, use instead the `` parameter with the `VocabularyName` or `VocabularyFilterName` (or both) sub-parameter.
*/
public var languageIdSettings: Map? = null
/**
* You can specify two or more language codes that represent the languages you think may be present in your media; including more than five is not recommended. If you're unsure what languages are present, do not include this parameter.
*
* If you include `LanguageOptions` in your request, you must also include `IdentifyLanguage`.
*
* For more information, refer to [Supported languages](https://docs.aws.amazon.com/transcribe/latest/dg/supported-languages.html).
*
* To transcribe speech in Modern Standard Arabic (`ar-SA`), your media file must be encoded at a sample rate of 16,000 Hz or higher.
*/
public var languageOptions: List? = null
/**
* Describes the Amazon S3 location of the media file you want to use in your request.
*/
public var media: aws.sdk.kotlin.services.transcribe.model.Media? = null
/**
* The format of the input media file.
*/
public var mediaFormat: aws.sdk.kotlin.services.transcribe.model.MediaFormat? = null
/**
* The sample rate, in Hertz, of the audio track in your input media file.
*/
public var mediaSampleRateHertz: kotlin.Int? = null
/**
* The custom language model you want to include with your transcription job. If you include `ModelSettings` in your request, you must include the `LanguageModelName` sub-parameter.
*/
public var modelSettings: aws.sdk.kotlin.services.transcribe.model.ModelSettings? = null
/**
* Specify additional optional settings in your request, including channel identification, alternative transcriptions, speaker labeling; allows you to apply custom vocabularies and vocabulary filters.
*
* If you want to include a custom vocabulary or a custom vocabulary filter (or both) with your request but **do not** want to use automatic language identification, use `Settings` with the `VocabularyName` or `VocabularyFilterName` (or both) sub-parameter.
*
* If you're using automatic language identification with your request and want to include a custom language model, a custom vocabulary, or a custom vocabulary filter, do not use the `Settings` parameter; use instead the `` parameter with the `LanguageModelName`, `VocabularyName` or `VocabularyFilterName` sub-parameters.
*/
public var settings: aws.sdk.kotlin.services.transcribe.model.Settings? = null
/**
* The date and time the specified transcription job began processing.
*
* Timestamps are in the format `YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC`. For example, `2022-05-04T12:32:58.789000-07:00` represents a transcription job that started processing at 12:32 PM UTC-7 on May 4, 2022.
*/
public var startTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Generate subtitles for your media file with your transcription request.
*/
public var subtitles: aws.sdk.kotlin.services.transcribe.model.SubtitlesOutput? = null
/**
* Adds one or more custom tags, each in the form of a key:value pair, to a new transcription job at the time you start this new job.
*
* To learn more about using tags with Amazon Transcribe, refer to [Tagging resources](https://docs.aws.amazon.com/transcribe/latest/dg/tagging.html).
*/
public var tags: List? = null
/**
* Provides you with the Amazon S3 URI you can use to access your transcript.
*/
public var transcript: aws.sdk.kotlin.services.transcribe.model.Transcript? = null
/**
* The name of the transcription job. Job names are case sensitive and must be unique within an Amazon Web Services account.
*/
public var transcriptionJobName: kotlin.String? = null
/**
* Provides the status of the specified transcription job.
*
* If the status is `COMPLETED`, the job is finished and you can find the results at the location specified in `TranscriptFileUri` (or `RedactedTranscriptFileUri`, if you requested transcript redaction). If the status is `FAILED`, `FailureReason` provides details on why your transcription job failed.
*/
public var transcriptionJobStatus: aws.sdk.kotlin.services.transcribe.model.TranscriptionJobStatus? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.transcribe.model.TranscriptionJob) : this() {
this.completionTime = x.completionTime
this.contentRedaction = x.contentRedaction
this.creationTime = x.creationTime
this.failureReason = x.failureReason
this.identifiedLanguageScore = x.identifiedLanguageScore
this.identifyLanguage = x.identifyLanguage
this.identifyMultipleLanguages = x.identifyMultipleLanguages
this.jobExecutionSettings = x.jobExecutionSettings
this.languageCode = x.languageCode
this.languageCodes = x.languageCodes
this.languageIdSettings = x.languageIdSettings
this.languageOptions = x.languageOptions
this.media = x.media
this.mediaFormat = x.mediaFormat
this.mediaSampleRateHertz = x.mediaSampleRateHertz
this.modelSettings = x.modelSettings
this.settings = x.settings
this.startTime = x.startTime
this.subtitles = x.subtitles
this.tags = x.tags
this.transcript = x.transcript
this.transcriptionJobName = x.transcriptionJobName
this.transcriptionJobStatus = x.transcriptionJobStatus
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.transcribe.model.TranscriptionJob = TranscriptionJob(this)
/**
* construct an [aws.sdk.kotlin.services.transcribe.model.ContentRedaction] inside the given [block]
*/
public fun contentRedaction(block: aws.sdk.kotlin.services.transcribe.model.ContentRedaction.Builder.() -> kotlin.Unit) {
this.contentRedaction = aws.sdk.kotlin.services.transcribe.model.ContentRedaction.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.transcribe.model.JobExecutionSettings] inside the given [block]
*/
public fun jobExecutionSettings(block: aws.sdk.kotlin.services.transcribe.model.JobExecutionSettings.Builder.() -> kotlin.Unit) {
this.jobExecutionSettings = aws.sdk.kotlin.services.transcribe.model.JobExecutionSettings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.transcribe.model.Media] inside the given [block]
*/
public fun media(block: aws.sdk.kotlin.services.transcribe.model.Media.Builder.() -> kotlin.Unit) {
this.media = aws.sdk.kotlin.services.transcribe.model.Media.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.transcribe.model.ModelSettings] inside the given [block]
*/
public fun modelSettings(block: aws.sdk.kotlin.services.transcribe.model.ModelSettings.Builder.() -> kotlin.Unit) {
this.modelSettings = aws.sdk.kotlin.services.transcribe.model.ModelSettings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.transcribe.model.Settings] inside the given [block]
*/
public fun settings(block: aws.sdk.kotlin.services.transcribe.model.Settings.Builder.() -> kotlin.Unit) {
this.settings = aws.sdk.kotlin.services.transcribe.model.Settings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.transcribe.model.SubtitlesOutput] inside the given [block]
*/
public fun subtitles(block: aws.sdk.kotlin.services.transcribe.model.SubtitlesOutput.Builder.() -> kotlin.Unit) {
this.subtitles = aws.sdk.kotlin.services.transcribe.model.SubtitlesOutput.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.transcribe.model.Transcript] inside the given [block]
*/
public fun transcript(block: aws.sdk.kotlin.services.transcribe.model.Transcript.Builder.() -> kotlin.Unit) {
this.transcript = aws.sdk.kotlin.services.transcribe.model.Transcript.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy