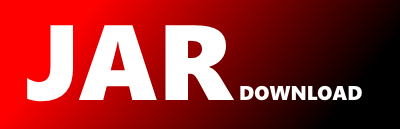
commonMain.aws.sdk.kotlin.services.transcribe.model.UpdateVocabularyResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of transcribe Show documentation
Show all versions of transcribe Show documentation
Amazon Transcribe Service
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.transcribe.model
import aws.smithy.kotlin.runtime.time.Instant
public class UpdateVocabularyResponse private constructor(builder: Builder) {
/**
* The language code you selected for your custom vocabulary.
*/
public val languageCode: aws.sdk.kotlin.services.transcribe.model.LanguageCode? = builder.languageCode
/**
* The date and time the specified vocabulary was last updated.
*
* Timestamps are in the format `YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC`. For example, `2022-05-04T12:32:58.761000-07:00` represents 12:32 PM UTC-7 on May 4, 2022.
*/
public val lastModifiedTime: aws.smithy.kotlin.runtime.time.Instant? = builder.lastModifiedTime
/**
* The name of the updated custom vocabulary.
*/
public val vocabularyName: kotlin.String? = builder.vocabularyName
/**
* The processing state of your custom vocabulary. If the state is `READY`, you can use the vocabulary in a `StartTranscriptionJob` request.
*/
public val vocabularyState: aws.sdk.kotlin.services.transcribe.model.VocabularyState? = builder.vocabularyState
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.transcribe.model.UpdateVocabularyResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("UpdateVocabularyResponse(")
append("languageCode=$languageCode,")
append("lastModifiedTime=$lastModifiedTime,")
append("vocabularyName=$vocabularyName,")
append("vocabularyState=$vocabularyState)")
}
override fun hashCode(): kotlin.Int {
var result = languageCode?.hashCode() ?: 0
result = 31 * result + (lastModifiedTime?.hashCode() ?: 0)
result = 31 * result + (vocabularyName?.hashCode() ?: 0)
result = 31 * result + (vocabularyState?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as UpdateVocabularyResponse
if (languageCode != other.languageCode) return false
if (lastModifiedTime != other.lastModifiedTime) return false
if (vocabularyName != other.vocabularyName) return false
if (vocabularyState != other.vocabularyState) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.transcribe.model.UpdateVocabularyResponse = Builder(this).apply(block).build()
public class Builder {
/**
* The language code you selected for your custom vocabulary.
*/
public var languageCode: aws.sdk.kotlin.services.transcribe.model.LanguageCode? = null
/**
* The date and time the specified vocabulary was last updated.
*
* Timestamps are in the format `YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC`. For example, `2022-05-04T12:32:58.761000-07:00` represents 12:32 PM UTC-7 on May 4, 2022.
*/
public var lastModifiedTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The name of the updated custom vocabulary.
*/
public var vocabularyName: kotlin.String? = null
/**
* The processing state of your custom vocabulary. If the state is `READY`, you can use the vocabulary in a `StartTranscriptionJob` request.
*/
public var vocabularyState: aws.sdk.kotlin.services.transcribe.model.VocabularyState? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.transcribe.model.UpdateVocabularyResponse) : this() {
this.languageCode = x.languageCode
this.lastModifiedTime = x.lastModifiedTime
this.vocabularyName = x.vocabularyName
this.vocabularyState = x.vocabularyState
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.transcribe.model.UpdateVocabularyResponse = UpdateVocabularyResponse(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy