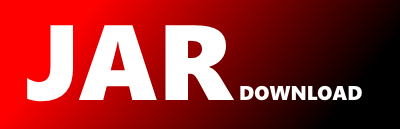
aws.sdk.kotlin.services.transcribe.DefaultTranscribeClient.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.transcribe
import aws.sdk.kotlin.runtime.client.AwsClientOption
import aws.sdk.kotlin.runtime.execution.AuthAttributes
import aws.sdk.kotlin.runtime.http.engine.crt.CrtHttpEngine
import aws.sdk.kotlin.services.transcribe.model.*
import aws.sdk.kotlin.services.transcribe.transform.*
import aws.smithy.kotlin.runtime.client.ExecutionContext
import aws.smithy.kotlin.runtime.client.SdkClientOption
import aws.smithy.kotlin.runtime.http.SdkHttpClient
import aws.smithy.kotlin.runtime.http.operation.SdkHttpOperation
import aws.smithy.kotlin.runtime.http.operation.context
import aws.smithy.kotlin.runtime.http.operation.roundTrip
import aws.smithy.kotlin.runtime.http.sdkHttpClient
import aws.smithy.kotlin.runtime.util.putIfAbsent
const val ServiceId: String = "Transcribe"
const val ServiceApiVersion: String = "2017-10-26"
const val SdkVersion: String = "0.9.1-alpha"
internal class DefaultTranscribeClient(override val config: TranscribeClient.Config) : TranscribeClient {
private val client: SdkHttpClient
init {
val httpClientEngine = config.httpClientEngine ?: CrtHttpEngine()
client = sdkHttpClient(httpClientEngine, manageEngine = config.httpClientEngine == null)
}
/**
* Creates an analytics category. Amazon Transcribe applies the conditions specified by your
* analytics categories to your call analytics jobs. For each analytics category, you specify one or
* more rules. For example, you can specify a rule that the customer sentiment was neutral or
* negative within that category. If you start a call analytics job, Amazon Transcribe applies the
* category to the analytics job that you've specified.
*/
override suspend fun createCallAnalyticsCategory(input: CreateCallAnalyticsCategoryRequest): CreateCallAnalyticsCategoryResponse {
val op = SdkHttpOperation.build {
serializer = CreateCallAnalyticsCategoryOperationSerializer()
deserializer = CreateCallAnalyticsCategoryOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "CreateCallAnalyticsCategory"
}
}
registerCreateCallAnalyticsCategoryMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Creates a new custom language model. Use Amazon S3 prefixes to provide the location of your input files. The time it
* takes to create your model depends on the size of your training data.
*/
override suspend fun createLanguageModel(input: CreateLanguageModelRequest): CreateLanguageModelResponse {
val op = SdkHttpOperation.build {
serializer = CreateLanguageModelOperationSerializer()
deserializer = CreateLanguageModelOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "CreateLanguageModel"
}
}
registerCreateLanguageModelMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Creates a new custom vocabulary that you can use to modify how Amazon Transcribe Medical transcribes your audio file.
*/
override suspend fun createMedicalVocabulary(input: CreateMedicalVocabularyRequest): CreateMedicalVocabularyResponse {
val op = SdkHttpOperation.build {
serializer = CreateMedicalVocabularyOperationSerializer()
deserializer = CreateMedicalVocabularyOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "CreateMedicalVocabulary"
}
}
registerCreateMedicalVocabularyMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Creates a new custom vocabulary that you can use to change the way Amazon Transcribe handles transcription of an
* audio file.
*/
override suspend fun createVocabulary(input: CreateVocabularyRequest): CreateVocabularyResponse {
val op = SdkHttpOperation.build {
serializer = CreateVocabularyOperationSerializer()
deserializer = CreateVocabularyOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "CreateVocabulary"
}
}
registerCreateVocabularyMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Creates a new vocabulary filter that you can use to filter words, such as profane words, from the output of
* a transcription job.
*/
override suspend fun createVocabularyFilter(input: CreateVocabularyFilterRequest): CreateVocabularyFilterResponse {
val op = SdkHttpOperation.build {
serializer = CreateVocabularyFilterOperationSerializer()
deserializer = CreateVocabularyFilterOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "CreateVocabularyFilter"
}
}
registerCreateVocabularyFilterMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Deletes a call analytics category using its name.
*/
override suspend fun deleteCallAnalyticsCategory(input: DeleteCallAnalyticsCategoryRequest): DeleteCallAnalyticsCategoryResponse {
val op = SdkHttpOperation.build {
serializer = DeleteCallAnalyticsCategoryOperationSerializer()
deserializer = DeleteCallAnalyticsCategoryOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "DeleteCallAnalyticsCategory"
}
}
registerDeleteCallAnalyticsCategoryMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Deletes a call analytics job using its name.
*/
override suspend fun deleteCallAnalyticsJob(input: DeleteCallAnalyticsJobRequest): DeleteCallAnalyticsJobResponse {
val op = SdkHttpOperation.build {
serializer = DeleteCallAnalyticsJobOperationSerializer()
deserializer = DeleteCallAnalyticsJobOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "DeleteCallAnalyticsJob"
}
}
registerDeleteCallAnalyticsJobMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Deletes a custom language model using its name.
*/
override suspend fun deleteLanguageModel(input: DeleteLanguageModelRequest): DeleteLanguageModelResponse {
val op = SdkHttpOperation.build {
serializer = DeleteLanguageModelOperationSerializer()
deserializer = DeleteLanguageModelOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "DeleteLanguageModel"
}
}
registerDeleteLanguageModelMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Deletes a transcription job generated by Amazon Transcribe Medical and any related information.
*/
override suspend fun deleteMedicalTranscriptionJob(input: DeleteMedicalTranscriptionJobRequest): DeleteMedicalTranscriptionJobResponse {
val op = SdkHttpOperation.build {
serializer = DeleteMedicalTranscriptionJobOperationSerializer()
deserializer = DeleteMedicalTranscriptionJobOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "DeleteMedicalTranscriptionJob"
}
}
registerDeleteMedicalTranscriptionJobMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Deletes a vocabulary from Amazon Transcribe Medical.
*/
override suspend fun deleteMedicalVocabulary(input: DeleteMedicalVocabularyRequest): DeleteMedicalVocabularyResponse {
val op = SdkHttpOperation.build {
serializer = DeleteMedicalVocabularyOperationSerializer()
deserializer = DeleteMedicalVocabularyOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "DeleteMedicalVocabulary"
}
}
registerDeleteMedicalVocabularyMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Deletes a previously submitted transcription job along with any other generated results such as the
* transcription, models, and so on.
*/
override suspend fun deleteTranscriptionJob(input: DeleteTranscriptionJobRequest): DeleteTranscriptionJobResponse {
val op = SdkHttpOperation.build {
serializer = DeleteTranscriptionJobOperationSerializer()
deserializer = DeleteTranscriptionJobOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "DeleteTranscriptionJob"
}
}
registerDeleteTranscriptionJobMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Deletes a vocabulary from Amazon Transcribe.
*/
override suspend fun deleteVocabulary(input: DeleteVocabularyRequest): DeleteVocabularyResponse {
val op = SdkHttpOperation.build {
serializer = DeleteVocabularyOperationSerializer()
deserializer = DeleteVocabularyOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "DeleteVocabulary"
}
}
registerDeleteVocabularyMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Removes a vocabulary filter.
*/
override suspend fun deleteVocabularyFilter(input: DeleteVocabularyFilterRequest): DeleteVocabularyFilterResponse {
val op = SdkHttpOperation.build {
serializer = DeleteVocabularyFilterOperationSerializer()
deserializer = DeleteVocabularyFilterOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "DeleteVocabularyFilter"
}
}
registerDeleteVocabularyFilterMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Gets information about a single custom language model. Use this information to see details about the
* language model in your Amazon Web Services account. You can also see whether the base language model used
* to create your custom language model has been updated. If Amazon Transcribe has updated the base model, you can create a
* new custom language model using the updated base model. If the language model wasn't created, you can use this
* operation to understand why Amazon Transcribe couldn't create it.
*/
override suspend fun describeLanguageModel(input: DescribeLanguageModelRequest): DescribeLanguageModelResponse {
val op = SdkHttpOperation.build {
serializer = DescribeLanguageModelOperationSerializer()
deserializer = DescribeLanguageModelOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "DescribeLanguageModel"
}
}
registerDescribeLanguageModelMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Retrieves information about a call analytics category.
*/
override suspend fun getCallAnalyticsCategory(input: GetCallAnalyticsCategoryRequest): GetCallAnalyticsCategoryResponse {
val op = SdkHttpOperation.build {
serializer = GetCallAnalyticsCategoryOperationSerializer()
deserializer = GetCallAnalyticsCategoryOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "GetCallAnalyticsCategory"
}
}
registerGetCallAnalyticsCategoryMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Returns information about a call analytics job. To see the status of the job, check the
* CallAnalyticsJobStatus field. If the status is COMPLETED, the job
* is finished and you can find the results at the location specified in the TranscriptFileUri
* field. If you enable personally identifiable information (PII) redaction, the redacted transcript appears
* in the RedactedTranscriptFileUri field.
*/
override suspend fun getCallAnalyticsJob(input: GetCallAnalyticsJobRequest): GetCallAnalyticsJobResponse {
val op = SdkHttpOperation.build {
serializer = GetCallAnalyticsJobOperationSerializer()
deserializer = GetCallAnalyticsJobOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "GetCallAnalyticsJob"
}
}
registerGetCallAnalyticsJobMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Returns information about a transcription job from Amazon Transcribe Medical. To see the status of the job, check the
* TranscriptionJobStatus field. If the status is COMPLETED, the job is finished. You
* find the results of the completed job in the TranscriptFileUri field.
*/
override suspend fun getMedicalTranscriptionJob(input: GetMedicalTranscriptionJobRequest): GetMedicalTranscriptionJobResponse {
val op = SdkHttpOperation.build {
serializer = GetMedicalTranscriptionJobOperationSerializer()
deserializer = GetMedicalTranscriptionJobOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "GetMedicalTranscriptionJob"
}
}
registerGetMedicalTranscriptionJobMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Retrieves information about a medical vocabulary.
*/
override suspend fun getMedicalVocabulary(input: GetMedicalVocabularyRequest): GetMedicalVocabularyResponse {
val op = SdkHttpOperation.build {
serializer = GetMedicalVocabularyOperationSerializer()
deserializer = GetMedicalVocabularyOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "GetMedicalVocabulary"
}
}
registerGetMedicalVocabularyMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Returns information about a transcription job. To see the status of the job, check the
* TranscriptionJobStatus field. If the status is COMPLETED, the job is finished and
* you can find the results at the location specified in the TranscriptFileUri field. If you enable content
* redaction, the redacted transcript appears in RedactedTranscriptFileUri.
*/
override suspend fun getTranscriptionJob(input: GetTranscriptionJobRequest): GetTranscriptionJobResponse {
val op = SdkHttpOperation.build {
serializer = GetTranscriptionJobOperationSerializer()
deserializer = GetTranscriptionJobOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "GetTranscriptionJob"
}
}
registerGetTranscriptionJobMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Gets information about a vocabulary.
*/
override suspend fun getVocabulary(input: GetVocabularyRequest): GetVocabularyResponse {
val op = SdkHttpOperation.build {
serializer = GetVocabularyOperationSerializer()
deserializer = GetVocabularyOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "GetVocabulary"
}
}
registerGetVocabularyMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Returns information about a vocabulary filter.
*/
override suspend fun getVocabularyFilter(input: GetVocabularyFilterRequest): GetVocabularyFilterResponse {
val op = SdkHttpOperation.build {
serializer = GetVocabularyFilterOperationSerializer()
deserializer = GetVocabularyFilterOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "GetVocabularyFilter"
}
}
registerGetVocabularyFilterMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Provides more information about the call analytics categories that you've created. You
* can use the information in this list to find a specific category. You can then use the
* operation to get more information about it.
*/
override suspend fun listCallAnalyticsCategories(input: ListCallAnalyticsCategoriesRequest): ListCallAnalyticsCategoriesResponse {
val op = SdkHttpOperation.build {
serializer = ListCallAnalyticsCategoriesOperationSerializer()
deserializer = ListCallAnalyticsCategoriesOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "ListCallAnalyticsCategories"
}
}
registerListCallAnalyticsCategoriesMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* List call analytics jobs with a specified status or substring that matches their names.
*/
override suspend fun listCallAnalyticsJobs(input: ListCallAnalyticsJobsRequest): ListCallAnalyticsJobsResponse {
val op = SdkHttpOperation.build {
serializer = ListCallAnalyticsJobsOperationSerializer()
deserializer = ListCallAnalyticsJobsOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "ListCallAnalyticsJobs"
}
}
registerListCallAnalyticsJobsMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Provides more information about the custom language models you've created. You can use the information in
* this list to find a specific custom language model. You can then use the
* operation to get more information about it.
*/
override suspend fun listLanguageModels(input: ListLanguageModelsRequest): ListLanguageModelsResponse {
val op = SdkHttpOperation.build {
serializer = ListLanguageModelsOperationSerializer()
deserializer = ListLanguageModelsOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "ListLanguageModels"
}
}
registerListLanguageModelsMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Lists medical transcription jobs with a specified status or substring that matches their names.
*/
override suspend fun listMedicalTranscriptionJobs(input: ListMedicalTranscriptionJobsRequest): ListMedicalTranscriptionJobsResponse {
val op = SdkHttpOperation.build {
serializer = ListMedicalTranscriptionJobsOperationSerializer()
deserializer = ListMedicalTranscriptionJobsOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "ListMedicalTranscriptionJobs"
}
}
registerListMedicalTranscriptionJobsMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Returns a list of vocabularies that match the specified criteria. If you don't enter a value in any of the request
* parameters, returns the entire list of vocabularies.
*/
override suspend fun listMedicalVocabularies(input: ListMedicalVocabulariesRequest): ListMedicalVocabulariesResponse {
val op = SdkHttpOperation.build {
serializer = ListMedicalVocabulariesOperationSerializer()
deserializer = ListMedicalVocabulariesOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "ListMedicalVocabularies"
}
}
registerListMedicalVocabulariesMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Lists all tags associated with a given transcription job, vocabulary, or resource.
*/
override suspend fun listTagsForResource(input: ListTagsForResourceRequest): ListTagsForResourceResponse {
val op = SdkHttpOperation.build {
serializer = ListTagsForResourceOperationSerializer()
deserializer = ListTagsForResourceOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "ListTagsForResource"
}
}
registerListTagsForResourceMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Lists transcription jobs with the specified status.
*/
override suspend fun listTranscriptionJobs(input: ListTranscriptionJobsRequest): ListTranscriptionJobsResponse {
val op = SdkHttpOperation.build {
serializer = ListTranscriptionJobsOperationSerializer()
deserializer = ListTranscriptionJobsOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "ListTranscriptionJobs"
}
}
registerListTranscriptionJobsMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Returns a list of vocabularies that match the specified criteria. If no criteria are specified, returns the entire list
* of vocabularies.
*/
override suspend fun listVocabularies(input: ListVocabulariesRequest): ListVocabulariesResponse {
val op = SdkHttpOperation.build {
serializer = ListVocabulariesOperationSerializer()
deserializer = ListVocabulariesOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "ListVocabularies"
}
}
registerListVocabulariesMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Gets information about vocabulary filters.
*/
override suspend fun listVocabularyFilters(input: ListVocabularyFiltersRequest): ListVocabularyFiltersResponse {
val op = SdkHttpOperation.build {
serializer = ListVocabularyFiltersOperationSerializer()
deserializer = ListVocabularyFiltersOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "ListVocabularyFilters"
}
}
registerListVocabularyFiltersMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Starts an asynchronous analytics job that not only transcribes the audio recording of a caller and agent, but
* also returns additional insights. These insights include how quickly or loudly the caller or agent was speaking. To
* retrieve additional insights with your analytics jobs, create categories. A category is a way to classify analytics jobs
* based on attributes, such as a customer's sentiment or a particular phrase being used during the call. For more
* information, see the operation.
*/
override suspend fun startCallAnalyticsJob(input: StartCallAnalyticsJobRequest): StartCallAnalyticsJobResponse {
val op = SdkHttpOperation.build {
serializer = StartCallAnalyticsJobOperationSerializer()
deserializer = StartCallAnalyticsJobOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "StartCallAnalyticsJob"
}
}
registerStartCallAnalyticsJobMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Starts a batch job to transcribe medical speech to text.
*/
override suspend fun startMedicalTranscriptionJob(input: StartMedicalTranscriptionJobRequest): StartMedicalTranscriptionJobResponse {
val op = SdkHttpOperation.build {
serializer = StartMedicalTranscriptionJobOperationSerializer()
deserializer = StartMedicalTranscriptionJobOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "StartMedicalTranscriptionJob"
}
}
registerStartMedicalTranscriptionJobMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Starts an asynchronous job to transcribe speech to text.
*/
override suspend fun startTranscriptionJob(input: StartTranscriptionJobRequest): StartTranscriptionJobResponse {
val op = SdkHttpOperation.build {
serializer = StartTranscriptionJobOperationSerializer()
deserializer = StartTranscriptionJobOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "StartTranscriptionJob"
}
}
registerStartTranscriptionJobMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Tags a Amazon Transcribe resource with the given list of tags.
*/
override suspend fun tagResource(input: TagResourceRequest): TagResourceResponse {
val op = SdkHttpOperation.build {
serializer = TagResourceOperationSerializer()
deserializer = TagResourceOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "TagResource"
}
}
registerTagResourceMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Removes specified tags from a specified Amazon Transcribe resource.
*/
override suspend fun untagResource(input: UntagResourceRequest): UntagResourceResponse {
val op = SdkHttpOperation.build {
serializer = UntagResourceOperationSerializer()
deserializer = UntagResourceOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "UntagResource"
}
}
registerUntagResourceMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Updates the call analytics category with new values. The UpdateCallAnalyticsCategory
* operation overwrites all of the existing information with the values that you provide in the request.
*/
override suspend fun updateCallAnalyticsCategory(input: UpdateCallAnalyticsCategoryRequest): UpdateCallAnalyticsCategoryResponse {
val op = SdkHttpOperation.build {
serializer = UpdateCallAnalyticsCategoryOperationSerializer()
deserializer = UpdateCallAnalyticsCategoryOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "UpdateCallAnalyticsCategory"
}
}
registerUpdateCallAnalyticsCategoryMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Updates a vocabulary with new values that you provide in a different text file from the one you used to create
* the vocabulary. The UpdateMedicalVocabulary operation overwrites all of the existing information
* with the values that you provide in the request.
*/
override suspend fun updateMedicalVocabulary(input: UpdateMedicalVocabularyRequest): UpdateMedicalVocabularyResponse {
val op = SdkHttpOperation.build {
serializer = UpdateMedicalVocabularyOperationSerializer()
deserializer = UpdateMedicalVocabularyOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "UpdateMedicalVocabulary"
}
}
registerUpdateMedicalVocabularyMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Updates an existing vocabulary with new values. The UpdateVocabulary operation overwrites
* all of the existing information with the values that you provide in the request.
*/
override suspend fun updateVocabulary(input: UpdateVocabularyRequest): UpdateVocabularyResponse {
val op = SdkHttpOperation.build {
serializer = UpdateVocabularyOperationSerializer()
deserializer = UpdateVocabularyOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "UpdateVocabulary"
}
}
registerUpdateVocabularyMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
/**
* Updates a vocabulary filter with a new list of filtered words.
*/
override suspend fun updateVocabularyFilter(input: UpdateVocabularyFilterRequest): UpdateVocabularyFilterResponse {
val op = SdkHttpOperation.build {
serializer = UpdateVocabularyFilterOperationSerializer()
deserializer = UpdateVocabularyFilterOperationDeserializer()
context {
expectedHttpStatus = 200
service = serviceName
operationName = "UpdateVocabularyFilter"
}
}
registerUpdateVocabularyFilterMiddleware(config, op)
mergeServiceDefaults(op.context)
return op.roundTrip(client, input)
}
override fun close() {
client.close()
}
/**
* merge the defaults configured for the service into the execution context before firing off a request
*/
private suspend fun mergeServiceDefaults(ctx: ExecutionContext) {
ctx.putIfAbsent(AwsClientOption.Region, config.region)
ctx.putIfAbsent(AuthAttributes.SigningRegion, config.region)
ctx.putIfAbsent(SdkClientOption.ServiceName, serviceName)
ctx.putIfAbsent(SdkClientOption.LogMode, config.sdkLogMode)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy