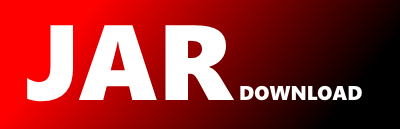
aws.sdk.kotlin.services.transcribe.TranscribeClient.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.transcribe
import aws.sdk.kotlin.runtime.auth.credentials.CredentialsProvider
import aws.sdk.kotlin.runtime.auth.credentials.DefaultChainCredentialsProvider
import aws.sdk.kotlin.runtime.client.AwsClientConfig
import aws.sdk.kotlin.runtime.config.AwsClientConfigLoadOptions
import aws.sdk.kotlin.runtime.config.fromEnvironment
import aws.sdk.kotlin.runtime.endpoint.AwsEndpointResolver
import aws.sdk.kotlin.services.transcribe.internal.DefaultEndpointResolver
import aws.sdk.kotlin.services.transcribe.model.*
import aws.smithy.kotlin.runtime.SdkClient
import aws.smithy.kotlin.runtime.client.SdkLogMode
import aws.smithy.kotlin.runtime.config.SdkClientConfig
import aws.smithy.kotlin.runtime.http.config.HttpClientConfig
import aws.smithy.kotlin.runtime.http.engine.HttpClientEngine
import aws.smithy.kotlin.runtime.http.operation.EndpointResolver
import aws.smithy.kotlin.runtime.retries.RetryStrategy
import aws.smithy.kotlin.runtime.retries.impl.ExponentialBackoffWithJitter
import aws.smithy.kotlin.runtime.retries.impl.ExponentialBackoffWithJitterOptions
import aws.smithy.kotlin.runtime.retries.impl.StandardRetryStrategy
import aws.smithy.kotlin.runtime.retries.impl.StandardRetryStrategyOptions
import aws.smithy.kotlin.runtime.retries.impl.StandardRetryTokenBucket
import aws.smithy.kotlin.runtime.retries.impl.StandardRetryTokenBucketOptions
/**
* Operations and objects for transcribing speech to text.
*/
interface TranscribeClient : SdkClient {
override val serviceName: String
get() = "Transcribe"
/**
* TranscribeClient's configuration
*/
val config: Config
companion object {
operator fun invoke(sharedConfig: AwsClientConfig? = null, block: Config.DslBuilder.() -> Unit = {}): TranscribeClient {
val config = Config.BuilderImpl().apply {
region = sharedConfig?.region
credentialsProvider = sharedConfig?.credentialsProvider
}.apply(block).build()
return DefaultTranscribeClient(config)
}
operator fun invoke(config: Config): TranscribeClient = DefaultTranscribeClient(config)
/**
* Construct a [TranscribeClient] by resolving the configuration from the current environment.
* NOTE: If you are using multiple AWS service clients you may wish to share the configuration among them
* by constructing a [aws.sdk.kotlin.runtime.client.AwsClientConfig] and passing it to each client at construction.
*/
suspend fun fromEnvironment(block: AwsClientConfigLoadOptions.() -> Unit = {}): TranscribeClient {
val sharedConfig = AwsClientConfig.fromEnvironment(block)
return TranscribeClient(sharedConfig)
}
}
class Config private constructor(builder: BuilderImpl): AwsClientConfig, HttpClientConfig, SdkClientConfig {
override val credentialsProvider: CredentialsProvider = builder.credentialsProvider ?: DefaultChainCredentialsProvider()
val endpointResolver: AwsEndpointResolver = builder.endpointResolver ?: DefaultEndpointResolver()
override val httpClientEngine: HttpClientEngine? = builder.httpClientEngine
override val region: String = requireNotNull(builder.region) { "region is a required configuration property" }
val retryStrategy: RetryStrategy = run {
val strategyOptions = StandardRetryStrategyOptions.Default
val tokenBucket = StandardRetryTokenBucket(StandardRetryTokenBucketOptions.Default)
val delayer = ExponentialBackoffWithJitter(ExponentialBackoffWithJitterOptions.Default)
StandardRetryStrategy(strategyOptions, tokenBucket, delayer)
}
override val sdkLogMode: SdkLogMode = builder.sdkLogMode
companion object {
@JvmStatic
fun fluentBuilder(): FluentBuilder = BuilderImpl()
operator fun invoke(block: DslBuilder.() -> kotlin.Unit): Config = BuilderImpl().apply(block).build()
}
interface FluentBuilder {
fun credentialsProvider(credentialsProvider: CredentialsProvider): FluentBuilder
fun endpointResolver(endpointResolver: AwsEndpointResolver): FluentBuilder
fun httpClientEngine(httpClientEngine: HttpClientEngine): FluentBuilder
fun region(region: String): FluentBuilder
fun sdkLogMode(sdkLogMode: SdkLogMode): FluentBuilder
fun build(): Config
}
interface DslBuilder {
/**
* The AWS credentials provider to use for authenticating requests. If not provided a
* [aws.sdk.kotlin.runtime.auth.credentials.DefaultChainCredentialsProvider] instance will be used.
*/
var credentialsProvider: CredentialsProvider?
/**
* Determines the endpoint (hostname) to make requests to. When not provided a default
* resolver is configured automatically. This is an advanced client option.
*/
var endpointResolver: AwsEndpointResolver?
/**
* Override the default HTTP client engine used to make SDK requests (e.g. configure proxy behavior, timeouts, concurrency, etc)
*/
var httpClientEngine: HttpClientEngine?
/**
* AWS region to make requests to
*/
var region: String?
/**
* Configure events that will be logged. By default clients will not output
* raw requests or responses. Use this setting to opt-in to additional debug logging.
* This can be used to configure logging of requests, responses, retries, etc of SDK clients.
* **NOTE**: Logging of raw requests or responses may leak sensitive information! It may also have
* performance considerations when dumping the request/response body. This is primarily a tool for
* debug purposes.
*/
var sdkLogMode: SdkLogMode
}
internal class BuilderImpl() : FluentBuilder, DslBuilder {
override var credentialsProvider: CredentialsProvider? = null
override var endpointResolver: AwsEndpointResolver? = null
override var httpClientEngine: HttpClientEngine? = null
override var region: String? = null
override var sdkLogMode: SdkLogMode = SdkLogMode.Default
override fun build(): Config = Config(this)
override fun credentialsProvider(credentialsProvider: CredentialsProvider): FluentBuilder = apply { this.credentialsProvider = credentialsProvider }
override fun endpointResolver(endpointResolver: AwsEndpointResolver): FluentBuilder = apply { this.endpointResolver = endpointResolver }
override fun httpClientEngine(httpClientEngine: HttpClientEngine): FluentBuilder = apply { this.httpClientEngine = httpClientEngine }
override fun region(region: String): FluentBuilder = apply { this.region = region }
override fun sdkLogMode(sdkLogMode: SdkLogMode): FluentBuilder = apply { this.sdkLogMode = sdkLogMode }
}
}
/**
* Creates an analytics category. Amazon Transcribe applies the conditions specified by your
* analytics categories to your call analytics jobs. For each analytics category, you specify one or
* more rules. For example, you can specify a rule that the customer sentiment was neutral or
* negative within that category. If you start a call analytics job, Amazon Transcribe applies the
* category to the analytics job that you've specified.
*/
suspend fun createCallAnalyticsCategory(input: CreateCallAnalyticsCategoryRequest): CreateCallAnalyticsCategoryResponse
/**
* Creates an analytics category. Amazon Transcribe applies the conditions specified by your
* analytics categories to your call analytics jobs. For each analytics category, you specify one or
* more rules. For example, you can specify a rule that the customer sentiment was neutral or
* negative within that category. If you start a call analytics job, Amazon Transcribe applies the
* category to the analytics job that you've specified.
*/
suspend fun createCallAnalyticsCategory(block: CreateCallAnalyticsCategoryRequest.DslBuilder.() -> Unit) = createCallAnalyticsCategory(CreateCallAnalyticsCategoryRequest.builder().apply(block).build())
/**
* Creates a new custom language model. Use Amazon S3 prefixes to provide the location of your input files. The time it
* takes to create your model depends on the size of your training data.
*/
suspend fun createLanguageModel(input: CreateLanguageModelRequest): CreateLanguageModelResponse
/**
* Creates a new custom language model. Use Amazon S3 prefixes to provide the location of your input files. The time it
* takes to create your model depends on the size of your training data.
*/
suspend fun createLanguageModel(block: CreateLanguageModelRequest.DslBuilder.() -> Unit) = createLanguageModel(CreateLanguageModelRequest.builder().apply(block).build())
/**
* Creates a new custom vocabulary that you can use to modify how Amazon Transcribe Medical transcribes your audio file.
*/
suspend fun createMedicalVocabulary(input: CreateMedicalVocabularyRequest): CreateMedicalVocabularyResponse
/**
* Creates a new custom vocabulary that you can use to modify how Amazon Transcribe Medical transcribes your audio file.
*/
suspend fun createMedicalVocabulary(block: CreateMedicalVocabularyRequest.DslBuilder.() -> Unit) = createMedicalVocabulary(CreateMedicalVocabularyRequest.builder().apply(block).build())
/**
* Creates a new custom vocabulary that you can use to change the way Amazon Transcribe handles transcription of an
* audio file.
*/
suspend fun createVocabulary(input: CreateVocabularyRequest): CreateVocabularyResponse
/**
* Creates a new custom vocabulary that you can use to change the way Amazon Transcribe handles transcription of an
* audio file.
*/
suspend fun createVocabulary(block: CreateVocabularyRequest.DslBuilder.() -> Unit) = createVocabulary(CreateVocabularyRequest.builder().apply(block).build())
/**
* Creates a new vocabulary filter that you can use to filter words, such as profane words, from the output of
* a transcription job.
*/
suspend fun createVocabularyFilter(input: CreateVocabularyFilterRequest): CreateVocabularyFilterResponse
/**
* Creates a new vocabulary filter that you can use to filter words, such as profane words, from the output of
* a transcription job.
*/
suspend fun createVocabularyFilter(block: CreateVocabularyFilterRequest.DslBuilder.() -> Unit) = createVocabularyFilter(CreateVocabularyFilterRequest.builder().apply(block).build())
/**
* Deletes a call analytics category using its name.
*/
suspend fun deleteCallAnalyticsCategory(input: DeleteCallAnalyticsCategoryRequest): DeleteCallAnalyticsCategoryResponse
/**
* Deletes a call analytics category using its name.
*/
suspend fun deleteCallAnalyticsCategory(block: DeleteCallAnalyticsCategoryRequest.DslBuilder.() -> Unit) = deleteCallAnalyticsCategory(DeleteCallAnalyticsCategoryRequest.builder().apply(block).build())
/**
* Deletes a call analytics job using its name.
*/
suspend fun deleteCallAnalyticsJob(input: DeleteCallAnalyticsJobRequest): DeleteCallAnalyticsJobResponse
/**
* Deletes a call analytics job using its name.
*/
suspend fun deleteCallAnalyticsJob(block: DeleteCallAnalyticsJobRequest.DslBuilder.() -> Unit) = deleteCallAnalyticsJob(DeleteCallAnalyticsJobRequest.builder().apply(block).build())
/**
* Deletes a custom language model using its name.
*/
suspend fun deleteLanguageModel(input: DeleteLanguageModelRequest): DeleteLanguageModelResponse
/**
* Deletes a custom language model using its name.
*/
suspend fun deleteLanguageModel(block: DeleteLanguageModelRequest.DslBuilder.() -> Unit) = deleteLanguageModel(DeleteLanguageModelRequest.builder().apply(block).build())
/**
* Deletes a transcription job generated by Amazon Transcribe Medical and any related information.
*/
suspend fun deleteMedicalTranscriptionJob(input: DeleteMedicalTranscriptionJobRequest): DeleteMedicalTranscriptionJobResponse
/**
* Deletes a transcription job generated by Amazon Transcribe Medical and any related information.
*/
suspend fun deleteMedicalTranscriptionJob(block: DeleteMedicalTranscriptionJobRequest.DslBuilder.() -> Unit) = deleteMedicalTranscriptionJob(DeleteMedicalTranscriptionJobRequest.builder().apply(block).build())
/**
* Deletes a vocabulary from Amazon Transcribe Medical.
*/
suspend fun deleteMedicalVocabulary(input: DeleteMedicalVocabularyRequest): DeleteMedicalVocabularyResponse
/**
* Deletes a vocabulary from Amazon Transcribe Medical.
*/
suspend fun deleteMedicalVocabulary(block: DeleteMedicalVocabularyRequest.DslBuilder.() -> Unit) = deleteMedicalVocabulary(DeleteMedicalVocabularyRequest.builder().apply(block).build())
/**
* Deletes a previously submitted transcription job along with any other generated results such as the
* transcription, models, and so on.
*/
suspend fun deleteTranscriptionJob(input: DeleteTranscriptionJobRequest): DeleteTranscriptionJobResponse
/**
* Deletes a previously submitted transcription job along with any other generated results such as the
* transcription, models, and so on.
*/
suspend fun deleteTranscriptionJob(block: DeleteTranscriptionJobRequest.DslBuilder.() -> Unit) = deleteTranscriptionJob(DeleteTranscriptionJobRequest.builder().apply(block).build())
/**
* Deletes a vocabulary from Amazon Transcribe.
*/
suspend fun deleteVocabulary(input: DeleteVocabularyRequest): DeleteVocabularyResponse
/**
* Deletes a vocabulary from Amazon Transcribe.
*/
suspend fun deleteVocabulary(block: DeleteVocabularyRequest.DslBuilder.() -> Unit) = deleteVocabulary(DeleteVocabularyRequest.builder().apply(block).build())
/**
* Removes a vocabulary filter.
*/
suspend fun deleteVocabularyFilter(input: DeleteVocabularyFilterRequest): DeleteVocabularyFilterResponse
/**
* Removes a vocabulary filter.
*/
suspend fun deleteVocabularyFilter(block: DeleteVocabularyFilterRequest.DslBuilder.() -> Unit) = deleteVocabularyFilter(DeleteVocabularyFilterRequest.builder().apply(block).build())
/**
* Gets information about a single custom language model. Use this information to see details about the
* language model in your Amazon Web Services account. You can also see whether the base language model used
* to create your custom language model has been updated. If Amazon Transcribe has updated the base model, you can create a
* new custom language model using the updated base model. If the language model wasn't created, you can use this
* operation to understand why Amazon Transcribe couldn't create it.
*/
suspend fun describeLanguageModel(input: DescribeLanguageModelRequest): DescribeLanguageModelResponse
/**
* Gets information about a single custom language model. Use this information to see details about the
* language model in your Amazon Web Services account. You can also see whether the base language model used
* to create your custom language model has been updated. If Amazon Transcribe has updated the base model, you can create a
* new custom language model using the updated base model. If the language model wasn't created, you can use this
* operation to understand why Amazon Transcribe couldn't create it.
*/
suspend fun describeLanguageModel(block: DescribeLanguageModelRequest.DslBuilder.() -> Unit) = describeLanguageModel(DescribeLanguageModelRequest.builder().apply(block).build())
/**
* Retrieves information about a call analytics category.
*/
suspend fun getCallAnalyticsCategory(input: GetCallAnalyticsCategoryRequest): GetCallAnalyticsCategoryResponse
/**
* Retrieves information about a call analytics category.
*/
suspend fun getCallAnalyticsCategory(block: GetCallAnalyticsCategoryRequest.DslBuilder.() -> Unit) = getCallAnalyticsCategory(GetCallAnalyticsCategoryRequest.builder().apply(block).build())
/**
* Returns information about a call analytics job. To see the status of the job, check the
* CallAnalyticsJobStatus field. If the status is COMPLETED, the job
* is finished and you can find the results at the location specified in the TranscriptFileUri
* field. If you enable personally identifiable information (PII) redaction, the redacted transcript appears
* in the RedactedTranscriptFileUri field.
*/
suspend fun getCallAnalyticsJob(input: GetCallAnalyticsJobRequest): GetCallAnalyticsJobResponse
/**
* Returns information about a call analytics job. To see the status of the job, check the
* CallAnalyticsJobStatus field. If the status is COMPLETED, the job
* is finished and you can find the results at the location specified in the TranscriptFileUri
* field. If you enable personally identifiable information (PII) redaction, the redacted transcript appears
* in the RedactedTranscriptFileUri field.
*/
suspend fun getCallAnalyticsJob(block: GetCallAnalyticsJobRequest.DslBuilder.() -> Unit) = getCallAnalyticsJob(GetCallAnalyticsJobRequest.builder().apply(block).build())
/**
* Returns information about a transcription job from Amazon Transcribe Medical. To see the status of the job, check the
* TranscriptionJobStatus field. If the status is COMPLETED, the job is finished. You
* find the results of the completed job in the TranscriptFileUri field.
*/
suspend fun getMedicalTranscriptionJob(input: GetMedicalTranscriptionJobRequest): GetMedicalTranscriptionJobResponse
/**
* Returns information about a transcription job from Amazon Transcribe Medical. To see the status of the job, check the
* TranscriptionJobStatus field. If the status is COMPLETED, the job is finished. You
* find the results of the completed job in the TranscriptFileUri field.
*/
suspend fun getMedicalTranscriptionJob(block: GetMedicalTranscriptionJobRequest.DslBuilder.() -> Unit) = getMedicalTranscriptionJob(GetMedicalTranscriptionJobRequest.builder().apply(block).build())
/**
* Retrieves information about a medical vocabulary.
*/
suspend fun getMedicalVocabulary(input: GetMedicalVocabularyRequest): GetMedicalVocabularyResponse
/**
* Retrieves information about a medical vocabulary.
*/
suspend fun getMedicalVocabulary(block: GetMedicalVocabularyRequest.DslBuilder.() -> Unit) = getMedicalVocabulary(GetMedicalVocabularyRequest.builder().apply(block).build())
/**
* Returns information about a transcription job. To see the status of the job, check the
* TranscriptionJobStatus field. If the status is COMPLETED, the job is finished and
* you can find the results at the location specified in the TranscriptFileUri field. If you enable content
* redaction, the redacted transcript appears in RedactedTranscriptFileUri.
*/
suspend fun getTranscriptionJob(input: GetTranscriptionJobRequest): GetTranscriptionJobResponse
/**
* Returns information about a transcription job. To see the status of the job, check the
* TranscriptionJobStatus field. If the status is COMPLETED, the job is finished and
* you can find the results at the location specified in the TranscriptFileUri field. If you enable content
* redaction, the redacted transcript appears in RedactedTranscriptFileUri.
*/
suspend fun getTranscriptionJob(block: GetTranscriptionJobRequest.DslBuilder.() -> Unit) = getTranscriptionJob(GetTranscriptionJobRequest.builder().apply(block).build())
/**
* Gets information about a vocabulary.
*/
suspend fun getVocabulary(input: GetVocabularyRequest): GetVocabularyResponse
/**
* Gets information about a vocabulary.
*/
suspend fun getVocabulary(block: GetVocabularyRequest.DslBuilder.() -> Unit) = getVocabulary(GetVocabularyRequest.builder().apply(block).build())
/**
* Returns information about a vocabulary filter.
*/
suspend fun getVocabularyFilter(input: GetVocabularyFilterRequest): GetVocabularyFilterResponse
/**
* Returns information about a vocabulary filter.
*/
suspend fun getVocabularyFilter(block: GetVocabularyFilterRequest.DslBuilder.() -> Unit) = getVocabularyFilter(GetVocabularyFilterRequest.builder().apply(block).build())
/**
* Provides more information about the call analytics categories that you've created. You
* can use the information in this list to find a specific category. You can then use the
* operation to get more information about it.
*/
suspend fun listCallAnalyticsCategories(input: ListCallAnalyticsCategoriesRequest): ListCallAnalyticsCategoriesResponse
/**
* Provides more information about the call analytics categories that you've created. You
* can use the information in this list to find a specific category. You can then use the
* operation to get more information about it.
*/
suspend fun listCallAnalyticsCategories(block: ListCallAnalyticsCategoriesRequest.DslBuilder.() -> Unit) = listCallAnalyticsCategories(ListCallAnalyticsCategoriesRequest.builder().apply(block).build())
/**
* List call analytics jobs with a specified status or substring that matches their names.
*/
suspend fun listCallAnalyticsJobs(input: ListCallAnalyticsJobsRequest): ListCallAnalyticsJobsResponse
/**
* List call analytics jobs with a specified status or substring that matches their names.
*/
suspend fun listCallAnalyticsJobs(block: ListCallAnalyticsJobsRequest.DslBuilder.() -> Unit) = listCallAnalyticsJobs(ListCallAnalyticsJobsRequest.builder().apply(block).build())
/**
* Provides more information about the custom language models you've created. You can use the information in
* this list to find a specific custom language model. You can then use the
* operation to get more information about it.
*/
suspend fun listLanguageModels(input: ListLanguageModelsRequest): ListLanguageModelsResponse
/**
* Provides more information about the custom language models you've created. You can use the information in
* this list to find a specific custom language model. You can then use the
* operation to get more information about it.
*/
suspend fun listLanguageModels(block: ListLanguageModelsRequest.DslBuilder.() -> Unit) = listLanguageModels(ListLanguageModelsRequest.builder().apply(block).build())
/**
* Lists medical transcription jobs with a specified status or substring that matches their names.
*/
suspend fun listMedicalTranscriptionJobs(input: ListMedicalTranscriptionJobsRequest): ListMedicalTranscriptionJobsResponse
/**
* Lists medical transcription jobs with a specified status or substring that matches their names.
*/
suspend fun listMedicalTranscriptionJobs(block: ListMedicalTranscriptionJobsRequest.DslBuilder.() -> Unit) = listMedicalTranscriptionJobs(ListMedicalTranscriptionJobsRequest.builder().apply(block).build())
/**
* Returns a list of vocabularies that match the specified criteria. If you don't enter a value in any of the request
* parameters, returns the entire list of vocabularies.
*/
suspend fun listMedicalVocabularies(input: ListMedicalVocabulariesRequest): ListMedicalVocabulariesResponse
/**
* Returns a list of vocabularies that match the specified criteria. If you don't enter a value in any of the request
* parameters, returns the entire list of vocabularies.
*/
suspend fun listMedicalVocabularies(block: ListMedicalVocabulariesRequest.DslBuilder.() -> Unit) = listMedicalVocabularies(ListMedicalVocabulariesRequest.builder().apply(block).build())
/**
* Lists all tags associated with a given transcription job, vocabulary, or resource.
*/
suspend fun listTagsForResource(input: ListTagsForResourceRequest): ListTagsForResourceResponse
/**
* Lists all tags associated with a given transcription job, vocabulary, or resource.
*/
suspend fun listTagsForResource(block: ListTagsForResourceRequest.DslBuilder.() -> Unit) = listTagsForResource(ListTagsForResourceRequest.builder().apply(block).build())
/**
* Lists transcription jobs with the specified status.
*/
suspend fun listTranscriptionJobs(input: ListTranscriptionJobsRequest): ListTranscriptionJobsResponse
/**
* Lists transcription jobs with the specified status.
*/
suspend fun listTranscriptionJobs(block: ListTranscriptionJobsRequest.DslBuilder.() -> Unit) = listTranscriptionJobs(ListTranscriptionJobsRequest.builder().apply(block).build())
/**
* Returns a list of vocabularies that match the specified criteria. If no criteria are specified, returns the entire list
* of vocabularies.
*/
suspend fun listVocabularies(input: ListVocabulariesRequest): ListVocabulariesResponse
/**
* Returns a list of vocabularies that match the specified criteria. If no criteria are specified, returns the entire list
* of vocabularies.
*/
suspend fun listVocabularies(block: ListVocabulariesRequest.DslBuilder.() -> Unit) = listVocabularies(ListVocabulariesRequest.builder().apply(block).build())
/**
* Gets information about vocabulary filters.
*/
suspend fun listVocabularyFilters(input: ListVocabularyFiltersRequest): ListVocabularyFiltersResponse
/**
* Gets information about vocabulary filters.
*/
suspend fun listVocabularyFilters(block: ListVocabularyFiltersRequest.DslBuilder.() -> Unit) = listVocabularyFilters(ListVocabularyFiltersRequest.builder().apply(block).build())
/**
* Starts an asynchronous analytics job that not only transcribes the audio recording of a caller and agent, but
* also returns additional insights. These insights include how quickly or loudly the caller or agent was speaking. To
* retrieve additional insights with your analytics jobs, create categories. A category is a way to classify analytics jobs
* based on attributes, such as a customer's sentiment or a particular phrase being used during the call. For more
* information, see the operation.
*/
suspend fun startCallAnalyticsJob(input: StartCallAnalyticsJobRequest): StartCallAnalyticsJobResponse
/**
* Starts an asynchronous analytics job that not only transcribes the audio recording of a caller and agent, but
* also returns additional insights. These insights include how quickly or loudly the caller or agent was speaking. To
* retrieve additional insights with your analytics jobs, create categories. A category is a way to classify analytics jobs
* based on attributes, such as a customer's sentiment or a particular phrase being used during the call. For more
* information, see the operation.
*/
suspend fun startCallAnalyticsJob(block: StartCallAnalyticsJobRequest.DslBuilder.() -> Unit) = startCallAnalyticsJob(StartCallAnalyticsJobRequest.builder().apply(block).build())
/**
* Starts a batch job to transcribe medical speech to text.
*/
suspend fun startMedicalTranscriptionJob(input: StartMedicalTranscriptionJobRequest): StartMedicalTranscriptionJobResponse
/**
* Starts a batch job to transcribe medical speech to text.
*/
suspend fun startMedicalTranscriptionJob(block: StartMedicalTranscriptionJobRequest.DslBuilder.() -> Unit) = startMedicalTranscriptionJob(StartMedicalTranscriptionJobRequest.builder().apply(block).build())
/**
* Starts an asynchronous job to transcribe speech to text.
*/
suspend fun startTranscriptionJob(input: StartTranscriptionJobRequest): StartTranscriptionJobResponse
/**
* Starts an asynchronous job to transcribe speech to text.
*/
suspend fun startTranscriptionJob(block: StartTranscriptionJobRequest.DslBuilder.() -> Unit) = startTranscriptionJob(StartTranscriptionJobRequest.builder().apply(block).build())
/**
* Tags a Amazon Transcribe resource with the given list of tags.
*/
suspend fun tagResource(input: TagResourceRequest): TagResourceResponse
/**
* Tags a Amazon Transcribe resource with the given list of tags.
*/
suspend fun tagResource(block: TagResourceRequest.DslBuilder.() -> Unit) = tagResource(TagResourceRequest.builder().apply(block).build())
/**
* Removes specified tags from a specified Amazon Transcribe resource.
*/
suspend fun untagResource(input: UntagResourceRequest): UntagResourceResponse
/**
* Removes specified tags from a specified Amazon Transcribe resource.
*/
suspend fun untagResource(block: UntagResourceRequest.DslBuilder.() -> Unit) = untagResource(UntagResourceRequest.builder().apply(block).build())
/**
* Updates the call analytics category with new values. The UpdateCallAnalyticsCategory
* operation overwrites all of the existing information with the values that you provide in the request.
*/
suspend fun updateCallAnalyticsCategory(input: UpdateCallAnalyticsCategoryRequest): UpdateCallAnalyticsCategoryResponse
/**
* Updates the call analytics category with new values. The UpdateCallAnalyticsCategory
* operation overwrites all of the existing information with the values that you provide in the request.
*/
suspend fun updateCallAnalyticsCategory(block: UpdateCallAnalyticsCategoryRequest.DslBuilder.() -> Unit) = updateCallAnalyticsCategory(UpdateCallAnalyticsCategoryRequest.builder().apply(block).build())
/**
* Updates a vocabulary with new values that you provide in a different text file from the one you used to create
* the vocabulary. The UpdateMedicalVocabulary operation overwrites all of the existing information
* with the values that you provide in the request.
*/
suspend fun updateMedicalVocabulary(input: UpdateMedicalVocabularyRequest): UpdateMedicalVocabularyResponse
/**
* Updates a vocabulary with new values that you provide in a different text file from the one you used to create
* the vocabulary. The UpdateMedicalVocabulary operation overwrites all of the existing information
* with the values that you provide in the request.
*/
suspend fun updateMedicalVocabulary(block: UpdateMedicalVocabularyRequest.DslBuilder.() -> Unit) = updateMedicalVocabulary(UpdateMedicalVocabularyRequest.builder().apply(block).build())
/**
* Updates an existing vocabulary with new values. The UpdateVocabulary operation overwrites
* all of the existing information with the values that you provide in the request.
*/
suspend fun updateVocabulary(input: UpdateVocabularyRequest): UpdateVocabularyResponse
/**
* Updates an existing vocabulary with new values. The UpdateVocabulary operation overwrites
* all of the existing information with the values that you provide in the request.
*/
suspend fun updateVocabulary(block: UpdateVocabularyRequest.DslBuilder.() -> Unit) = updateVocabulary(UpdateVocabularyRequest.builder().apply(block).build())
/**
* Updates a vocabulary filter with a new list of filtered words.
*/
suspend fun updateVocabularyFilter(input: UpdateVocabularyFilterRequest): UpdateVocabularyFilterResponse
/**
* Updates a vocabulary filter with a new list of filtered words.
*/
suspend fun updateVocabularyFilter(block: UpdateVocabularyFilterRequest.DslBuilder.() -> Unit) = updateVocabularyFilter(UpdateVocabularyFilterRequest.builder().apply(block).build())
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy