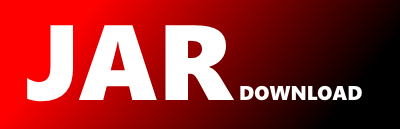
aws.sdk.kotlin.services.transcribe.model.ListMedicalTranscriptionJobsRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of transcribe Show documentation
Show all versions of transcribe Show documentation
Amazon Transcribe Service
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.transcribe.model
class ListMedicalTranscriptionJobsRequest private constructor(builder: BuilderImpl) {
/**
* When specified, the jobs returned in the list are limited to jobs whose name contains the specified string.
*/
val jobNameContains: String? = builder.jobNameContains
/**
* The maximum number of medical transcription jobs to return in each page of results. If there are fewer
* results than the value you specify, only the actual results are returned. If you do not specify a value, the default of
* 5 is used.
*/
val maxResults: Int? = builder.maxResults
/**
* If you a receive a truncated result in the previous request of ListMedicalTranscriptionJobs,
* include NextToken to fetch the next set of jobs.
*/
val nextToken: String? = builder.nextToken
/**
* When specified, returns only medical transcription jobs with the specified status. Jobs are ordered by creation
* date, with the newest jobs returned first. If you don't specify a status, Amazon Transcribe Medical returns all transcription jobs ordered
* by creation date.
*/
val status: TranscriptionJobStatus? = builder.status
companion object {
@JvmStatic
fun fluentBuilder(): FluentBuilder = BuilderImpl()
internal fun builder(): DslBuilder = BuilderImpl()
operator fun invoke(block: DslBuilder.() -> kotlin.Unit): ListMedicalTranscriptionJobsRequest = BuilderImpl().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ListMedicalTranscriptionJobsRequest(")
append("jobNameContains=$jobNameContains,")
append("maxResults=$maxResults,")
append("nextToken=$nextToken,")
append("status=$status)")
}
override fun hashCode(): kotlin.Int {
var result = jobNameContains?.hashCode() ?: 0
result = 31 * result + (maxResults ?: 0)
result = 31 * result + (nextToken?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as ListMedicalTranscriptionJobsRequest
if (jobNameContains != other.jobNameContains) return false
if (maxResults != other.maxResults) return false
if (nextToken != other.nextToken) return false
if (status != other.status) return false
return true
}
fun copy(block: DslBuilder.() -> kotlin.Unit = {}): ListMedicalTranscriptionJobsRequest = BuilderImpl(this).apply(block).build()
interface FluentBuilder {
fun build(): ListMedicalTranscriptionJobsRequest
/**
* When specified, the jobs returned in the list are limited to jobs whose name contains the specified string.
*/
fun jobNameContains(jobNameContains: String): FluentBuilder
/**
* The maximum number of medical transcription jobs to return in each page of results. If there are fewer
* results than the value you specify, only the actual results are returned. If you do not specify a value, the default of
* 5 is used.
*/
fun maxResults(maxResults: Int): FluentBuilder
/**
* If you a receive a truncated result in the previous request of ListMedicalTranscriptionJobs,
* include NextToken to fetch the next set of jobs.
*/
fun nextToken(nextToken: String): FluentBuilder
/**
* When specified, returns only medical transcription jobs with the specified status. Jobs are ordered by creation
* date, with the newest jobs returned first. If you don't specify a status, Amazon Transcribe Medical returns all transcription jobs ordered
* by creation date.
*/
fun status(status: TranscriptionJobStatus): FluentBuilder
}
interface DslBuilder {
/**
* When specified, the jobs returned in the list are limited to jobs whose name contains the specified string.
*/
var jobNameContains: String?
/**
* The maximum number of medical transcription jobs to return in each page of results. If there are fewer
* results than the value you specify, only the actual results are returned. If you do not specify a value, the default of
* 5 is used.
*/
var maxResults: Int?
/**
* If you a receive a truncated result in the previous request of ListMedicalTranscriptionJobs,
* include NextToken to fetch the next set of jobs.
*/
var nextToken: String?
/**
* When specified, returns only medical transcription jobs with the specified status. Jobs are ordered by creation
* date, with the newest jobs returned first. If you don't specify a status, Amazon Transcribe Medical returns all transcription jobs ordered
* by creation date.
*/
var status: TranscriptionJobStatus?
fun build(): ListMedicalTranscriptionJobsRequest
}
private class BuilderImpl() : FluentBuilder, DslBuilder {
override var jobNameContains: String? = null
override var maxResults: Int? = null
override var nextToken: String? = null
override var status: TranscriptionJobStatus? = null
constructor(x: ListMedicalTranscriptionJobsRequest) : this() {
this.jobNameContains = x.jobNameContains
this.maxResults = x.maxResults
this.nextToken = x.nextToken
this.status = x.status
}
override fun build(): ListMedicalTranscriptionJobsRequest = ListMedicalTranscriptionJobsRequest(this)
override fun jobNameContains(jobNameContains: String): FluentBuilder = apply { this.jobNameContains = jobNameContains }
override fun maxResults(maxResults: Int): FluentBuilder = apply { this.maxResults = maxResults }
override fun nextToken(nextToken: String): FluentBuilder = apply { this.nextToken = nextToken }
override fun status(status: TranscriptionJobStatus): FluentBuilder = apply { this.status = status }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy