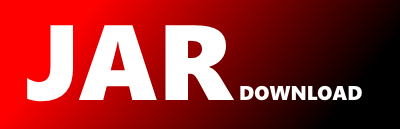
aws.sdk.kotlin.services.transcribe.model.CallAnalyticsJobSummary.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of transcribe Show documentation
Show all versions of transcribe Show documentation
Amazon Transcribe Service
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.transcribe.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* Provides summary information about a call analytics job.
*/
class CallAnalyticsJobSummary private constructor(builder: Builder) {
/**
* The name of the call analytics job.
*/
val callAnalyticsJobName: kotlin.String? = builder.callAnalyticsJobName
/**
* The status of the call analytics job.
*/
val callAnalyticsJobStatus: aws.sdk.kotlin.services.transcribe.model.CallAnalyticsJobStatus? = builder.callAnalyticsJobStatus
/**
* A timestamp that shows when the job was completed.
*/
val completionTime: aws.smithy.kotlin.runtime.time.Instant? = builder.completionTime
/**
* A timestamp that shows when the call analytics job was created.
*/
val creationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.creationTime
/**
* If the CallAnalyticsJobStatus is FAILED, a description of the error.
*/
val failureReason: kotlin.String? = builder.failureReason
/**
* The language of the transcript in the source audio file.
*/
val languageCode: aws.sdk.kotlin.services.transcribe.model.LanguageCode? = builder.languageCode
/**
* A timestamp that shows when the job began processing.
*/
val startTime: aws.smithy.kotlin.runtime.time.Instant? = builder.startTime
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.transcribe.model.CallAnalyticsJobSummary = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CallAnalyticsJobSummary(")
append("callAnalyticsJobName=$callAnalyticsJobName,")
append("callAnalyticsJobStatus=$callAnalyticsJobStatus,")
append("completionTime=$completionTime,")
append("creationTime=$creationTime,")
append("failureReason=$failureReason,")
append("languageCode=$languageCode,")
append("startTime=$startTime)")
}
override fun hashCode(): kotlin.Int {
var result = callAnalyticsJobName?.hashCode() ?: 0
result = 31 * result + (callAnalyticsJobStatus?.hashCode() ?: 0)
result = 31 * result + (completionTime?.hashCode() ?: 0)
result = 31 * result + (creationTime?.hashCode() ?: 0)
result = 31 * result + (failureReason?.hashCode() ?: 0)
result = 31 * result + (languageCode?.hashCode() ?: 0)
result = 31 * result + (startTime?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CallAnalyticsJobSummary
if (callAnalyticsJobName != other.callAnalyticsJobName) return false
if (callAnalyticsJobStatus != other.callAnalyticsJobStatus) return false
if (completionTime != other.completionTime) return false
if (creationTime != other.creationTime) return false
if (failureReason != other.failureReason) return false
if (languageCode != other.languageCode) return false
if (startTime != other.startTime) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.transcribe.model.CallAnalyticsJobSummary = Builder(this).apply(block).build()
class Builder {
/**
* The name of the call analytics job.
*/
var callAnalyticsJobName: kotlin.String? = null
/**
* The status of the call analytics job.
*/
var callAnalyticsJobStatus: aws.sdk.kotlin.services.transcribe.model.CallAnalyticsJobStatus? = null
/**
* A timestamp that shows when the job was completed.
*/
var completionTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* A timestamp that shows when the call analytics job was created.
*/
var creationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* If the CallAnalyticsJobStatus is FAILED, a description of the error.
*/
var failureReason: kotlin.String? = null
/**
* The language of the transcript in the source audio file.
*/
var languageCode: aws.sdk.kotlin.services.transcribe.model.LanguageCode? = null
/**
* A timestamp that shows when the job began processing.
*/
var startTime: aws.smithy.kotlin.runtime.time.Instant? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.transcribe.model.CallAnalyticsJobSummary) : this() {
this.callAnalyticsJobName = x.callAnalyticsJobName
this.callAnalyticsJobStatus = x.callAnalyticsJobStatus
this.completionTime = x.completionTime
this.creationTime = x.creationTime
this.failureReason = x.failureReason
this.languageCode = x.languageCode
this.startTime = x.startTime
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.transcribe.model.CallAnalyticsJobSummary = CallAnalyticsJobSummary(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy