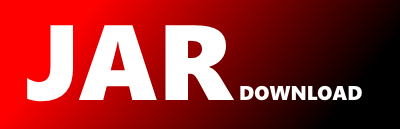
aws.sdk.kotlin.services.transcribe.model.ListVocabulariesRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of transcribe Show documentation
Show all versions of transcribe Show documentation
Amazon Transcribe Service
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.transcribe.model
class ListVocabulariesRequest private constructor(builder: Builder) {
/**
* The maximum number of vocabularies to return in each page of results. If there are fewer results than the
* value you specify, only the actual results are returned. If you do not specify a value, the default of 5 is used.
*/
val maxResults: kotlin.Int? = builder.maxResults
/**
* When specified, the vocabularies returned in the list are limited to vocabularies whose name contains the
* specified string. The search is not case sensitive, ListVocabularies returns both "vocabularyname"
* and "VocabularyName" in the response list.
*/
val nameContains: kotlin.String? = builder.nameContains
/**
* If the result of the previous request to ListVocabularies was truncated, include the
* NextToken to fetch the next set of jobs.
*/
val nextToken: kotlin.String? = builder.nextToken
/**
* When specified, only returns vocabularies with the VocabularyState field equal to the
* specified state.
*/
val stateEquals: aws.sdk.kotlin.services.transcribe.model.VocabularyState? = builder.stateEquals
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.transcribe.model.ListVocabulariesRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ListVocabulariesRequest(")
append("maxResults=$maxResults,")
append("nameContains=$nameContains,")
append("nextToken=$nextToken,")
append("stateEquals=$stateEquals)")
}
override fun hashCode(): kotlin.Int {
var result = maxResults ?: 0
result = 31 * result + (nameContains?.hashCode() ?: 0)
result = 31 * result + (nextToken?.hashCode() ?: 0)
result = 31 * result + (stateEquals?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ListVocabulariesRequest
if (maxResults != other.maxResults) return false
if (nameContains != other.nameContains) return false
if (nextToken != other.nextToken) return false
if (stateEquals != other.stateEquals) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.transcribe.model.ListVocabulariesRequest = Builder(this).apply(block).build()
class Builder {
/**
* The maximum number of vocabularies to return in each page of results. If there are fewer results than the
* value you specify, only the actual results are returned. If you do not specify a value, the default of 5 is used.
*/
var maxResults: kotlin.Int? = null
/**
* When specified, the vocabularies returned in the list are limited to vocabularies whose name contains the
* specified string. The search is not case sensitive, ListVocabularies returns both "vocabularyname"
* and "VocabularyName" in the response list.
*/
var nameContains: kotlin.String? = null
/**
* If the result of the previous request to ListVocabularies was truncated, include the
* NextToken to fetch the next set of jobs.
*/
var nextToken: kotlin.String? = null
/**
* When specified, only returns vocabularies with the VocabularyState field equal to the
* specified state.
*/
var stateEquals: aws.sdk.kotlin.services.transcribe.model.VocabularyState? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.transcribe.model.ListVocabulariesRequest) : this() {
this.maxResults = x.maxResults
this.nameContains = x.nameContains
this.nextToken = x.nextToken
this.stateEquals = x.stateEquals
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.transcribe.model.ListVocabulariesRequest = ListVocabulariesRequest(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy