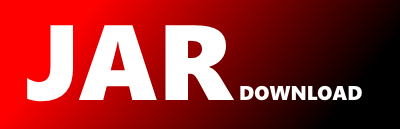
aws.sdk.kotlin.services.transcribe.model.MedicalTranscriptionSetting.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.transcribe.model
/**
* Optional settings for the StartMedicalTranscriptionJob
* operation.
*/
class MedicalTranscriptionSetting private constructor(builder: Builder) {
/**
* Instructs Amazon Transcribe Medical to process each audio channel separately and then merge the transcription output of each
* channel into a single transcription.
* Amazon Transcribe Medical also produces a transcription of each item detected on an audio channel, including the start time and end
* time of the item and alternative transcriptions of item. The alternative transcriptions also come with confidence scores
* provided by Amazon Transcribe Medical.
* You can't set both ShowSpeakerLabels and ChannelIdentification in the same
* request. If you set both, your request returns a BadRequestException
*/
val channelIdentification: kotlin.Boolean? = builder.channelIdentification
/**
* The maximum number of alternatives that you tell the service to return. If you specify the
* MaxAlternatives field, you must set the ShowAlternatives field to true.
*/
val maxAlternatives: kotlin.Int? = builder.maxAlternatives
/**
* The maximum number of speakers to identify in the input audio. If there are more speakers in the audio than this
* number, multiple speakers are identified as a single speaker. If you specify the MaxSpeakerLabels field,
* you must set the ShowSpeakerLabels field to true.
*/
val maxSpeakerLabels: kotlin.Int? = builder.maxSpeakerLabels
/**
* Determines whether alternative transcripts are generated along with the transcript that has the highest confidence.
* If you set ShowAlternatives field to true, you must also set the maximum number of alternatives to
* return in the MaxAlternatives field.
*/
val showAlternatives: kotlin.Boolean? = builder.showAlternatives
/**
* Determines whether the transcription job uses speaker recognition to identify different speakers in the input
* audio. Speaker recognition labels individual speakers in the audio file. If you set the ShowSpeakerLabels
* field to true, you must also set the maximum number of speaker labels in the MaxSpeakerLabels
* field.
* You can't set both ShowSpeakerLabels and ChannelIdentification in the same
* request. If you set both, your request returns a BadRequestException.
*/
val showSpeakerLabels: kotlin.Boolean? = builder.showSpeakerLabels
/**
* The name of the vocabulary to use when processing a medical transcription job.
*/
val vocabularyName: kotlin.String? = builder.vocabularyName
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.transcribe.model.MedicalTranscriptionSetting = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("MedicalTranscriptionSetting(")
append("channelIdentification=$channelIdentification,")
append("maxAlternatives=$maxAlternatives,")
append("maxSpeakerLabels=$maxSpeakerLabels,")
append("showAlternatives=$showAlternatives,")
append("showSpeakerLabels=$showSpeakerLabels,")
append("vocabularyName=$vocabularyName)")
}
override fun hashCode(): kotlin.Int {
var result = channelIdentification?.hashCode() ?: 0
result = 31 * result + (maxAlternatives ?: 0)
result = 31 * result + (maxSpeakerLabels ?: 0)
result = 31 * result + (showAlternatives?.hashCode() ?: 0)
result = 31 * result + (showSpeakerLabels?.hashCode() ?: 0)
result = 31 * result + (vocabularyName?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as MedicalTranscriptionSetting
if (channelIdentification != other.channelIdentification) return false
if (maxAlternatives != other.maxAlternatives) return false
if (maxSpeakerLabels != other.maxSpeakerLabels) return false
if (showAlternatives != other.showAlternatives) return false
if (showSpeakerLabels != other.showSpeakerLabels) return false
if (vocabularyName != other.vocabularyName) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.transcribe.model.MedicalTranscriptionSetting = Builder(this).apply(block).build()
class Builder {
/**
* Instructs Amazon Transcribe Medical to process each audio channel separately and then merge the transcription output of each
* channel into a single transcription.
* Amazon Transcribe Medical also produces a transcription of each item detected on an audio channel, including the start time and end
* time of the item and alternative transcriptions of item. The alternative transcriptions also come with confidence scores
* provided by Amazon Transcribe Medical.
* You can't set both ShowSpeakerLabels and ChannelIdentification in the same
* request. If you set both, your request returns a BadRequestException
*/
var channelIdentification: kotlin.Boolean? = null
/**
* The maximum number of alternatives that you tell the service to return. If you specify the
* MaxAlternatives field, you must set the ShowAlternatives field to true.
*/
var maxAlternatives: kotlin.Int? = null
/**
* The maximum number of speakers to identify in the input audio. If there are more speakers in the audio than this
* number, multiple speakers are identified as a single speaker. If you specify the MaxSpeakerLabels field,
* you must set the ShowSpeakerLabels field to true.
*/
var maxSpeakerLabels: kotlin.Int? = null
/**
* Determines whether alternative transcripts are generated along with the transcript that has the highest confidence.
* If you set ShowAlternatives field to true, you must also set the maximum number of alternatives to
* return in the MaxAlternatives field.
*/
var showAlternatives: kotlin.Boolean? = null
/**
* Determines whether the transcription job uses speaker recognition to identify different speakers in the input
* audio. Speaker recognition labels individual speakers in the audio file. If you set the ShowSpeakerLabels
* field to true, you must also set the maximum number of speaker labels in the MaxSpeakerLabels
* field.
* You can't set both ShowSpeakerLabels and ChannelIdentification in the same
* request. If you set both, your request returns a BadRequestException.
*/
var showSpeakerLabels: kotlin.Boolean? = null
/**
* The name of the vocabulary to use when processing a medical transcription job.
*/
var vocabularyName: kotlin.String? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.transcribe.model.MedicalTranscriptionSetting) : this() {
this.channelIdentification = x.channelIdentification
this.maxAlternatives = x.maxAlternatives
this.maxSpeakerLabels = x.maxSpeakerLabels
this.showAlternatives = x.showAlternatives
this.showSpeakerLabels = x.showSpeakerLabels
this.vocabularyName = x.vocabularyName
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.transcribe.model.MedicalTranscriptionSetting = MedicalTranscriptionSetting(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy